C# 中將浮點數四捨五入到小數點後兩位
Minahil Noor
2024年2月16日
Csharp
Csharp Decimal
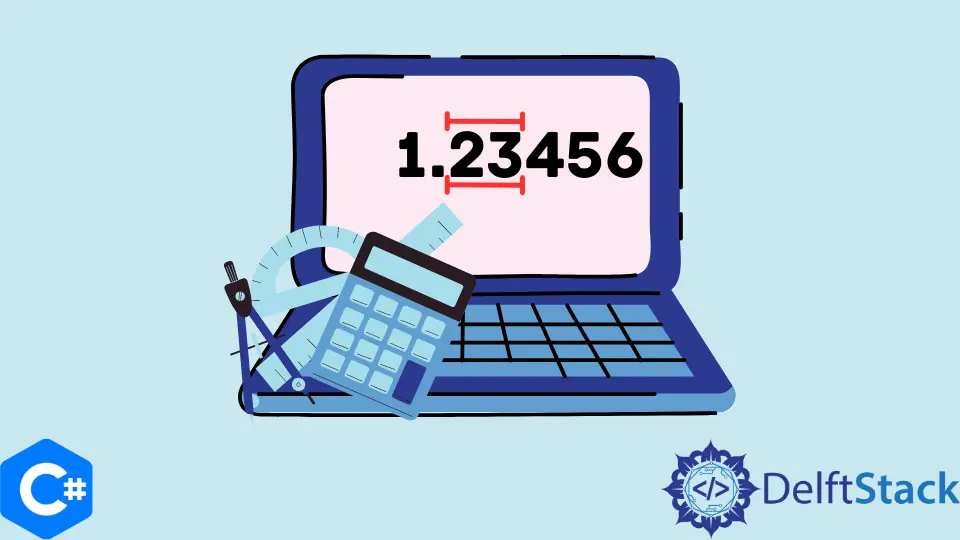
在 C# 中,我們可以使用不同的方法輕鬆舍入一個浮點數,例如 decimal.Round()
和 Math.Round()
。
本文將重點介紹將十進位制值浮點數舍入到兩個十進位制位的方法。
C# 使用 decimal.Round()
方法將十進位制值舍入到兩個十進位制位
decimal.Round()
方法是將十進位制數字四捨五入為指定的位數的最簡單的方法。此方法最多允許 28 個小數位。
此方法的正確語法如下:
decimal.Round(decimalValueName, integerValue);
示例程式碼:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 123.456M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = decimal.Round(decimalValue, 2);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
輸出:
The Decimal Value Before Applying Method: 123.456
The Decimal Value After Applying Method: 123.46
如果要舍入的小數位數的值不在 0-28 範圍內,則此方法將丟擲 ArgumentOutOfRangeException
。然後,通過使用 try-catch
塊來處理該異常。
還有另一種使用 decimal.Round()
方法的方式,示例程式碼如下:
示例程式碼:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 12.345M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = decimal.Round(decimalValue, 2, MidpointRounding.AwayFromZero);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
輸出:
The Decimal Value Before Applying Method: 12.345
The Decimal Value After Applying Method: 12.35
MidpointRounding.AwayFromZero
用於將數字從零舍入。它的對應物件是 MidpointRounding.ToEven
,它將給定的十進位制數四捨五入到最接近的偶數。
示例程式碼:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 12.345M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = decimal.Round(decimalValue, 2, MidpointRounding.ToEven);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
輸出:
The Decimal Value Before Applying Method: 12.345
The Decimal Value After Applying Method: 12.34
C# 使用 Math.Round()
方法將十進位制值四捨五入到兩個十進位制位
Math.Round()
方法與 decimal.Round()
相同。
此方法的正確語法如下:
Math.Round(decimalValueName, integerValue);
示例程式碼:
using System;
public class RoundDecimal {
public static void Main() {
decimal decimalValue = 123.456M;
Console.WriteLine("The Decimal Value Before Applying Method: {0}", decimalValue);
Console.WriteLine();
decimalValue = Math.Round(decimalValue, 2);
Console.WriteLine("The Decimal Value After Applying Method: {0}", decimalValue);
}
}
輸出:
The Decimal Value Before Applying Method: 123.456
The Decimal Value After Applying Method: 123.46
就像 decimal.Round()
方法一樣,它也會丟擲異常,然後使用 try-catch
塊來處理。我們也可以像 decimal.Round()
方法那樣指定 MidpointRounding
方法。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe