Cのstructとtypedef structの違い
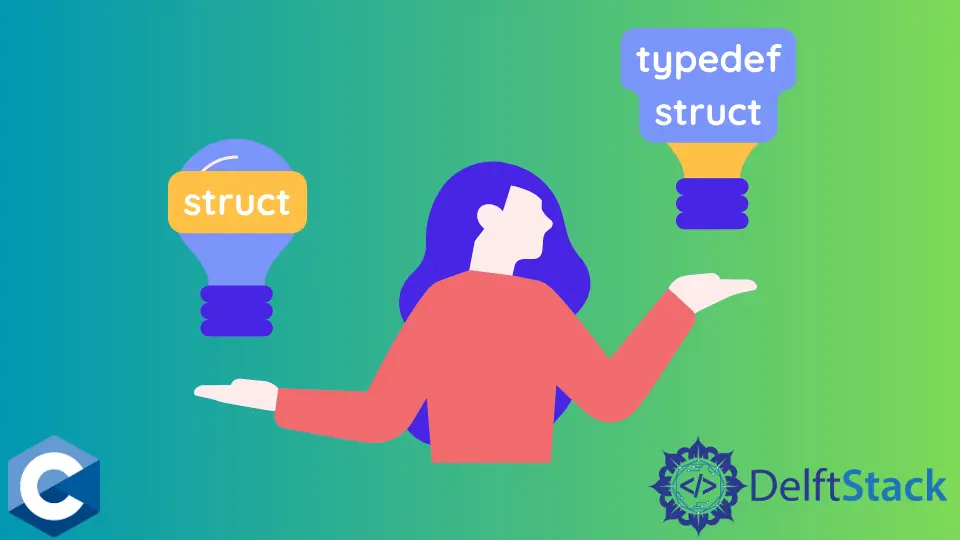
このチュートリアルでは、コード例を使用して、C プログラミングにおける struct
と typedef struct
の違いを説明し、実演します。
C の構造体
配列を使用する場合、同じ型/種類の多くのデータ項目を保持する変数の型を定義します。 同様に、C プログラミングには、構造体 と呼ばれる別のユーザー定義データ型があり、異なる型のデータ項目を組み合わせることができます。
構造体を使用してレコードを表すこともできます。 たとえば、各人物が次の属性を持つ人物の詳細を追跡できます。
- ファーストネーム
- 苗字
- 性別
- 年
- モバイル
- Eメール
- 住所
Cのstruct
とtypedef struct
の違い
struct
と typedef struct
を使用して構造体を定義できますが、typedef
キーワードを使用すると、ユーザー定義のデータ型 (struct など) とプリミティブ データ型 (int など) の代替名を記述できます。
typedef
キーワードは、既存のデータ型に新しい名前を作成しますが、新しいデータ型は作成しません。 typedef struct
を使用すると、よりクリーンで読みやすいコードを作成できます。これにより、プログラマーはキーストロークからも解放されます。
typedef struct
は、変数の宣言を簡素化し、同等のコードに簡素化された構文を提供します。 ただし、グローバル名前空間がより乱雑になる可能性があり、より大規模なプログラムで問題が発生する可能性があります。
両方のシナリオ (struct
と typedef struct
) のサンプル コードを用意して、違いを理解しましょう。
typedef
キーワードを使用しないコード例
#include <stdio.h>
struct Books {
int id;
char author[50];
char title[50];
};
int main() {
// declare `book1` and `book2` of type `Books`
struct Books book1;
struct Books book2;
// the specifications for the `book1`
book1.id = 1;
strcpy(book1.author, "Zara Ali");
strcpy(book1.title, "Tutorials for C Programming");
// the specifications for the `book2`
book2.id = 2;
strcpy(book2.author, "Zaid Ali");
strcpy(book2.title, "Tutorials for Java Programming");
// display information for `book1` and `book2`
printf("The id of book1: %d\n", book1.id);
printf("The author of the book1: %s\n", book1.author);
printf("The title of the book1: %s\n", book1.title);
printf("The id of book2: %d\n", book2.id);
printf("The author of the book2: %s\n", book2.author);
printf("The title of the book2: %s\n", book2.title);
return 0;
}
出力:
The id of book1: 1
The author of the book1: Zara Ali
The title of the book1: Tutorials for C Programming
The id of book2: 2
The author of the book2: Zaid Ali
The title of the book2: Tutorials for Java Programming
上記のコードには、Books
型の 2つの変数 book1
と book2
があります。 さらに変数を宣言する必要がある場合は、book3
、book4
などのように、struct
を何度も入力する必要があります。
ここで、typedef struct
の出番です。 typedef struct
の使用を示す次のコード スニペットを参照してください。
typedef
キーワードを使用したコード例
次の 2つの方法で typedef
を使用できます。
方法 1:
#include <stdio.h>
struct Books {
int id;
char author[50];
char title[50];
};
typedef struct Books Book;
int main() {
// declare `book1` and `book2` of type `Book`
Book book1;
Book book2;
// the specifications for the `book1`
book1.id = 1;
strcpy(book1.author, "Zara Ali");
strcpy(book1.title, "Tutorials for C Programming");
// the specifications for the `book2`
book2.id = 2;
strcpy(book2.author, "Zaid Ali");
strcpy(book2.title, "Tutorials for Java Programming");
// display information for `book1` and `book2`
printf("The id of book1: %d\n", book1.id);
printf("The author of the book1: %s\n", book1.author);
printf("The title of the book1: %s\n", book1.title);
printf("The id of book2: %d\n", book2.id);
printf("The author of the book2: %s\n", book2.author);
printf("The title of the book2: %s\n", book2.title);
return 0;
}
出力:
The id of book1: 1
The author of the book1: Zara Ali
The title of the book1: Tutorials for C Programming
The id of book2: 2
The author of the book2: Zaid Ali
The title of the book2: Tutorials for Java Programming
方法 2:
#include <stdio.h>
typedef struct Books {
int id;
char author[50];
char title[50];
} Book;
int main() {
// declare `book1` and `book2` of type `Book`
Book book1;
Book book2;
// the specifications for the `book1`
book1.id = 1;
strcpy(book1.author, "Zara Ali");
strcpy(book1.title, "Tutorials for C Programming");
// the specifications for the `book2`
book2.id = 2;
strcpy(book2.author, "Zaid Ali");
strcpy(book2.title, "Tutorials for Java Programming");
// display information for `book1` and `book2`
printf("The id of book1: %d\n", book1.id);
printf("The author of the book1: %s\n", book1.author);
printf("The title of the book1: %s\n", book1.title);
printf("The id of book2: %d\n", book2.id);
printf("The author of the book2: %s\n", book2.author);
printf("The title of the book2: %s\n", book2.title);
return 0;
}
出力:
The id of book1: 1
The author of the book1: Zara Ali
The title of the book1: Tutorials for C Programming
The id of book2: 2
The author of the book2: Zaid Ali
The title of the book2: Tutorials for Java Programming
2 番目の方法は、typedef
を使用すると、より整理され、クリーンで、理解しやすく、管理しやすいことを示しています。
typedef
キーワードを使用する際の重要なポイント
typedef
キーワードを使用する際には、いくつかの点を覚えておく必要があります。 上記のコードでは、次のように構造体を定義しています。
typedef struct Books {
int id;
char author[50];
char title[50];
} Book;
ここでは、新しい構造体型 struct Books
と、struct Books
の Book
という名前のエイリアスがあります。 typedef
を含む別名が、他の通常の宣言と同様に宣言の最後に表示される理由を不思議に思うかもしれません。
typedef
は、auto
や static
などのストレージ クラス指定子として正確な指定子のカテゴリに入れられました。 Book
型識別子のみを使用したい場合は、タグ名なしで宣言することもできます。
typedef struct {
int id;
char author[50];
char title[50];
} Book;
構造体を使用している間は、変数とエイリアスと呼ぶべきものに注意する必要があります。 次のように匿名構造体を使用する場合 (構造体に名前がないため匿名)、Book
はユーザー定義のデータ型構造体変数になります。
struct {
int id;
char author[50];
char title[50];
} Book;
上記と同じ構造体を typedef
キーワードとともに使用すると、Book
はデータ型構造体のエイリアス (同義語) になり、それを使用して変数を宣言できます (以下を参照)。
typedef struct {
int id;
char author[50];
char title[50];
} Book;