C에서 구조체와 Typedef 구조체의 차이점
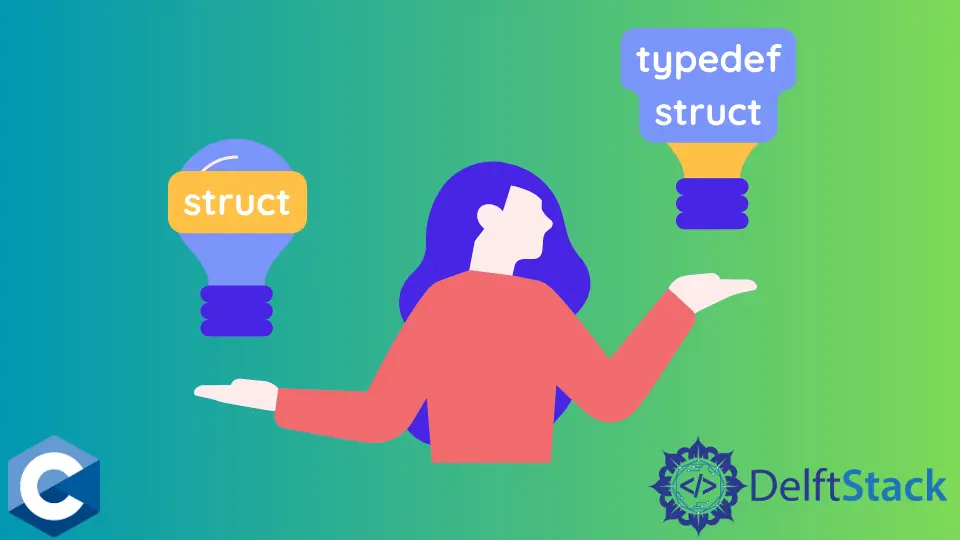
이 자습서에서는 코드 예제를 통해 C 프로그래밍에서 struct
와 typedef struct
의 차이점을 설명하고 시연합니다.
C의 구조
배열을 사용할 때 동일한 유형/종류의 많은 데이터 항목을 보유하는 변수의 유형을 정의합니다. 마찬가지로 C 프로그래밍에는 구조라고 하는 또 다른 사용자 정의 데이터 유형이 있어 다양한 유형의 데이터 항목을 결합할 수 있습니다.
구조를 사용하여 레코드를 나타낼 수도 있습니다. 예를 들어, 각 사람이 다음과 같은 속성을 가지고 있는 사람의 세부 정보를 추적할 수 있습니다.
- 이름
- 성
- 성별
- 나이
- 이동하는
- 이메일
- 주소
C에서 struct
와 typedef struct
의 차이점
struct
및 typedef struct
를 사용하여 구조를 정의할 수 있지만 typedef
키워드를 사용하면 사용자 정의 데이터 유형(예: struct) 및 기본 데이터 유형(예: int)에 대한 대체 이름을 작성할 수 있습니다.
typedef
키워드는 기존 데이터 유형에 새로운 이름을 생성하지만 새 데이터 유형을 생성하지는 않습니다. typedef struct
를 사용하면 더 깨끗하고 읽기 쉬운 코드를 가질 수 있으며 키 입력으로부터 우리(프로그래머)를 구해줍니다.
typedef struct
는 변수 선언을 단순화하여 단순화된 구문으로 동등한 코드를 제공할 수 있습니다. 그러나 더 복잡한 전역 이름 공간이 생겨 더 광범위한 프로그램에 문제가 발생할 수 있습니다.
두 시나리오(struct
및 typedef struct
)에 대한 샘플 코드를 가지고 차이점을 이해해 봅시다.
typedef
키워드가 없는 예제 코드
#include <stdio.h>
struct Books {
int id;
char author[50];
char title[50];
};
int main() {
// declare `book1` and `book2` of type `Books`
struct Books book1;
struct Books book2;
// the specifications for the `book1`
book1.id = 1;
strcpy(book1.author, "Zara Ali");
strcpy(book1.title, "Tutorials for C Programming");
// the specifications for the `book2`
book2.id = 2;
strcpy(book2.author, "Zaid Ali");
strcpy(book2.title, "Tutorials for Java Programming");
// display information for `book1` and `book2`
printf("The id of book1: %d\n", book1.id);
printf("The author of the book1: %s\n", book1.author);
printf("The title of the book1: %s\n", book1.title);
printf("The id of book2: %d\n", book2.id);
printf("The author of the book2: %s\n", book2.author);
printf("The title of the book2: %s\n", book2.title);
return 0;
}
출력:
The id of book1: 1
The author of the book1: Zara Ali
The title of the book1: Tutorials for C Programming
The id of book2: 2
The author of the book2: Zaid Ali
The title of the book2: Tutorials for Java Programming
위의 코드에는 Books
유형의 book1
및 book2
라는 두 개의 변수가 있습니다. 더 많은 변수(예: book3
, book4
등)를 선언해야 하는 경우 struct
를 반복해서 입력해야 합니다.
여기서 typedef struct
가 등장합니다. typedef struct
사용을 보여주는 다음 코드 스니펫을 참조하십시오.
typedef
키워드가 있는 예제 코드
다음 두 가지 방법에서 typedef
를 사용할 수 있습니다.
방법 1:
#include <stdio.h>
struct Books {
int id;
char author[50];
char title[50];
};
typedef struct Books Book;
int main() {
// declare `book1` and `book2` of type `Book`
Book book1;
Book book2;
// the specifications for the `book1`
book1.id = 1;
strcpy(book1.author, "Zara Ali");
strcpy(book1.title, "Tutorials for C Programming");
// the specifications for the `book2`
book2.id = 2;
strcpy(book2.author, "Zaid Ali");
strcpy(book2.title, "Tutorials for Java Programming");
// display information for `book1` and `book2`
printf("The id of book1: %d\n", book1.id);
printf("The author of the book1: %s\n", book1.author);
printf("The title of the book1: %s\n", book1.title);
printf("The id of book2: %d\n", book2.id);
printf("The author of the book2: %s\n", book2.author);
printf("The title of the book2: %s\n", book2.title);
return 0;
}
출력:
The id of book1: 1
The author of the book1: Zara Ali
The title of the book1: Tutorials for C Programming
The id of book2: 2
The author of the book2: Zaid Ali
The title of the book2: Tutorials for Java Programming
방법 2:
#include <stdio.h>
typedef struct Books {
int id;
char author[50];
char title[50];
} Book;
int main() {
// declare `book1` and `book2` of type `Book`
Book book1;
Book book2;
// the specifications for the `book1`
book1.id = 1;
strcpy(book1.author, "Zara Ali");
strcpy(book1.title, "Tutorials for C Programming");
// the specifications for the `book2`
book2.id = 2;
strcpy(book2.author, "Zaid Ali");
strcpy(book2.title, "Tutorials for Java Programming");
// display information for `book1` and `book2`
printf("The id of book1: %d\n", book1.id);
printf("The author of the book1: %s\n", book1.author);
printf("The title of the book1: %s\n", book1.title);
printf("The id of book2: %d\n", book2.id);
printf("The author of the book2: %s\n", book2.author);
printf("The title of the book2: %s\n", book2.title);
return 0;
}
출력:
The id of book1: 1
The author of the book1: Zara Ali
The title of the book1: Tutorials for C Programming
The id of book2: 2
The author of the book2: Zaid Ali
The title of the book2: Tutorials for Java Programming
두 번째 방법은 typedef
를 사용하는 것이 더 조직적이고 깨끗하며 이해하고 관리하기 쉽다는 것을 보여줍니다.
typedef
키워드 사용 시 핵심 사항
typedef
키워드를 사용하는 동안 몇 가지 사항을 기억해야 합니다. 위에 주어진 코드에서 다음과 같이 구조를 정의하고 있습니다.
typedef struct Books {
int id;
char author[50];
char title[50];
} Book;
여기에는 struct Books
라는 새로운 구조 유형과 struct Books
에 대한 Book
이라는 별칭이 있습니다. typedef
가 있는 별칭 이름이 다른 일반 선언과 마찬가지로 선언 끝에 나타나는 이유가 궁금할 수 있습니다.
typedef
는 스토리지 클래스 지정자(예: auto
및 static
)와 같은 정확한 지정자 범주에 포함되었습니다. Book
유형 식별자만 사용하려는 경우 태그 이름 없이 선언할 수도 있습니다.
typedef struct {
int id;
char author[50];
char title[50];
} Book;
구조체를 사용하는 동안 변수와 별칭을 호출해야 하는 항목에 주의해야 합니다. 다음과 같이 익명 구조를 사용하는 경우(구조에 이름이 없기 때문에 익명) 도서
는 사용자 정의 데이터 유형 구조 변수가 됩니다.
struct {
int id;
char author[50];
char title[50];
} Book;
위에 주어진 것과 동일한 구조를 사용하지만 typedef
키워드와 함께 사용하는 경우 Book
은 데이터 유형 구조의 별칭(동의어)이 되며 이를 사용하여 변수를 선언할 수 있습니다(다음 참조).
typedef struct {
int id;
char author[50];
char title[50];
} Book;