Diferencia entre struct y typedef struct en C
- Estructura en C
-
Diferencia entre
struct
ytypedef struct
en C -
Puntos clave al usar la palabra clave
typedef
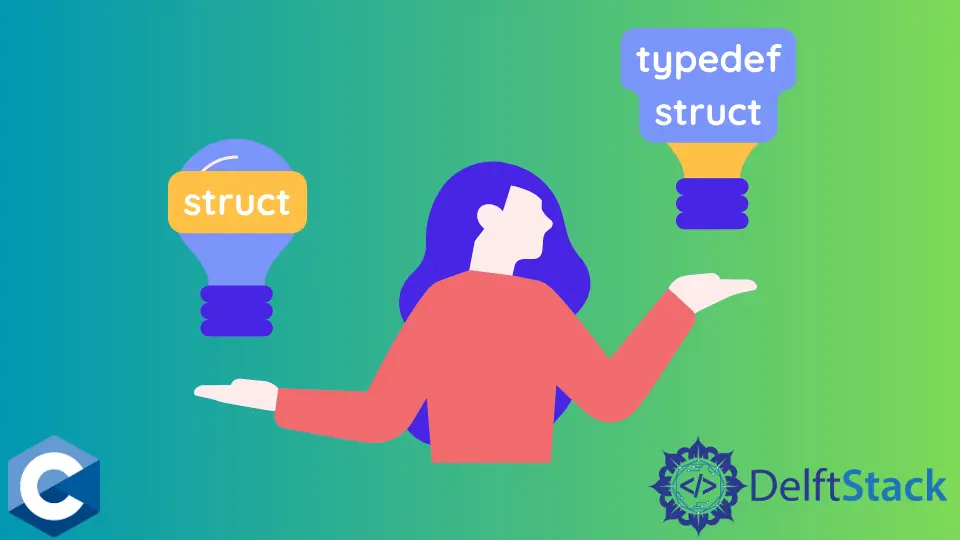
Este tutorial explicará y demostrará la diferencia entre struct
y typedef struct
en programación C con la ayuda de ejemplos de código.
Estructura en C
Cuando usamos matrices, definimos el tipo de variable que contiene muchos elementos de datos del mismo tipo/tipo. De manera similar, tenemos otro tipo de datos definido por el usuario en la programación C, conocido como estructura, que nos permite combinar diferentes tipos de elementos de datos.
También podemos representar un registro usando estructuras. Por ejemplo, podemos realizar un seguimiento de los detalles de la persona donde cada persona tiene los siguientes atributos:
- Nombre de pila
- Apellido
- Género
- Edad
- Móvil
- Correo electrónico
- DIRECCIÓN
Diferencia entre struct
y typedef struct
en C
Podemos definir una estructura usando struct
y typedef struct
, pero la palabra clave typedef
nos permite escribir nombres alternativos para los tipos de datos definidos por el usuario (p. ej., struct) y tipos de datos primitivos (p. ej., int).
La palabra clave typedef
crea un nombre completamente nuevo para un tipo de datos ya existente, pero no crea el nuevo tipo de datos. Podemos tener un código más limpio y legible si usamos la typedef struct
, y además nos ahorra (al programador) pulsaciones de teclas.
La typedef struct
puede simplificar la declaración de variables, proporcionando el código equivalente con una sintaxis simplificada. Sin embargo, puede conducir a un espacio de nombres global más desordenado, lo que puede causar problemas para programas más extensos.
Tengamos un código de muestra para ambos escenarios (la struct
y la typedef struct
) y comprendamos la diferencia.
Código de ejemplo sin la palabra clave typedef
#include <stdio.h>
struct Books {
int id;
char author[50];
char title[50];
};
int main() {
// declare `book1` and `book2` of type `Books`
struct Books book1;
struct Books book2;
// the specifications for the `book1`
book1.id = 1;
strcpy(book1.author, "Zara Ali");
strcpy(book1.title, "Tutorials for C Programming");
// the specifications for the `book2`
book2.id = 2;
strcpy(book2.author, "Zaid Ali");
strcpy(book2.title, "Tutorials for Java Programming");
// display information for `book1` and `book2`
printf("The id of book1: %d\n", book1.id);
printf("The author of the book1: %s\n", book1.author);
printf("The title of the book1: %s\n", book1.title);
printf("The id of book2: %d\n", book2.id);
printf("The author of the book2: %s\n", book2.author);
printf("The title of the book2: %s\n", book2.title);
return 0;
}
Producción :
The id of book1: 1
The author of the book1: Zara Ali
The title of the book1: Tutorials for C Programming
The id of book2: 2
The author of the book2: Zaid Ali
The title of the book2: Tutorials for Java Programming
El código anterior tiene dos variables, libro1
y libro2
, de tipo Libros
. Tendremos que teclear struct
una y otra vez si necesitamos declarar más variables, por ejemplo, book3
, book4
, etc.
Aquí es donde entra en escena la typedef struct
. Consulte el siguiente fragmento de código que muestra el uso de typedef struct
.
Código de ejemplo con la palabra clave typedef
Podemos usar el typedef
en los siguientes dos métodos.
Método 1:
#include <stdio.h>
struct Books {
int id;
char author[50];
char title[50];
};
typedef struct Books Book;
int main() {
// declare `book1` and `book2` of type `Book`
Book book1;
Book book2;
// the specifications for the `book1`
book1.id = 1;
strcpy(book1.author, "Zara Ali");
strcpy(book1.title, "Tutorials for C Programming");
// the specifications for the `book2`
book2.id = 2;
strcpy(book2.author, "Zaid Ali");
strcpy(book2.title, "Tutorials for Java Programming");
// display information for `book1` and `book2`
printf("The id of book1: %d\n", book1.id);
printf("The author of the book1: %s\n", book1.author);
printf("The title of the book1: %s\n", book1.title);
printf("The id of book2: %d\n", book2.id);
printf("The author of the book2: %s\n", book2.author);
printf("The title of the book2: %s\n", book2.title);
return 0;
}
Producción :
The id of book1: 1
The author of the book1: Zara Ali
The title of the book1: Tutorials for C Programming
The id of book2: 2
The author of the book2: Zaid Ali
The title of the book2: Tutorials for Java Programming
Método 2:
#include <stdio.h>
typedef struct Books {
int id;
char author[50];
char title[50];
} Book;
int main() {
// declare `book1` and `book2` of type `Book`
Book book1;
Book book2;
// the specifications for the `book1`
book1.id = 1;
strcpy(book1.author, "Zara Ali");
strcpy(book1.title, "Tutorials for C Programming");
// the specifications for the `book2`
book2.id = 2;
strcpy(book2.author, "Zaid Ali");
strcpy(book2.title, "Tutorials for Java Programming");
// display information for `book1` and `book2`
printf("The id of book1: %d\n", book1.id);
printf("The author of the book1: %s\n", book1.author);
printf("The title of the book1: %s\n", book1.title);
printf("The id of book2: %d\n", book2.id);
printf("The author of the book2: %s\n", book2.author);
printf("The title of the book2: %s\n", book2.title);
return 0;
}
Producción :
The id of book1: 1
The author of the book1: Zara Ali
The title of the book1: Tutorials for C Programming
The id of book2: 2
The author of the book2: Zaid Ali
The title of the book2: Tutorials for Java Programming
El segundo método muestra que usar typedef
parece más organizado, limpio y fácil de entender y administrar.
Puntos clave al usar la palabra clave typedef
Debemos recordar algunos puntos al usar la palabra clave typedef
. En el código anterior, estamos definiendo la estructura de la siguiente manera.
typedef struct Books {
int id;
char author[50];
char title[50];
} Book;
Aquí tenemos un nuevo tipo de estructura, struct Books
, y el alias denominado Book
para los struct Books
. Quizás se pregunte por qué el nombre de alias con el typedef
aparece al final de la declaración como cualquier otra declaración ordinaria.
El typedef
se colocó en la categoría de especificador exacto como los especificadores de clase de almacenamiento, por ejemplo, auto
y static
. También podemos declarar sin el nombre de la etiqueta si solo queremos utilizar el identificador de tipo Libro
.
typedef struct {
int id;
char author[50];
char title[50];
} Book;
Mientras usamos estructuras, debemos tener cuidado con lo que deberíamos llamar una variable y un alias. Si usamos la estructura anónima de la siguiente manera (anónima porque la estructura no tiene nombre), entonces el Book
sería la variable de estructura de tipo de datos definida por el usuario.
struct {
int id;
char author[50];
char title[50];
} Book;
Si usamos la misma estructura dada arriba pero con la palabra clave typedef
, entonces el Book
sería un alias (sinónimo) de la estructura del tipo de datos, y podemos usar eso para declarar variables (vea lo siguiente).
typedef struct {
int id;
char author[50];
char title[50];
} Book;