Unterschied zwischen Struct und Typedef Struct in C
- Struktur in C
-
Unterschied zwischen
struct
undtypedef struct
in C -
Wichtige Punkte bei der Verwendung des Schlüsselworts
typedef
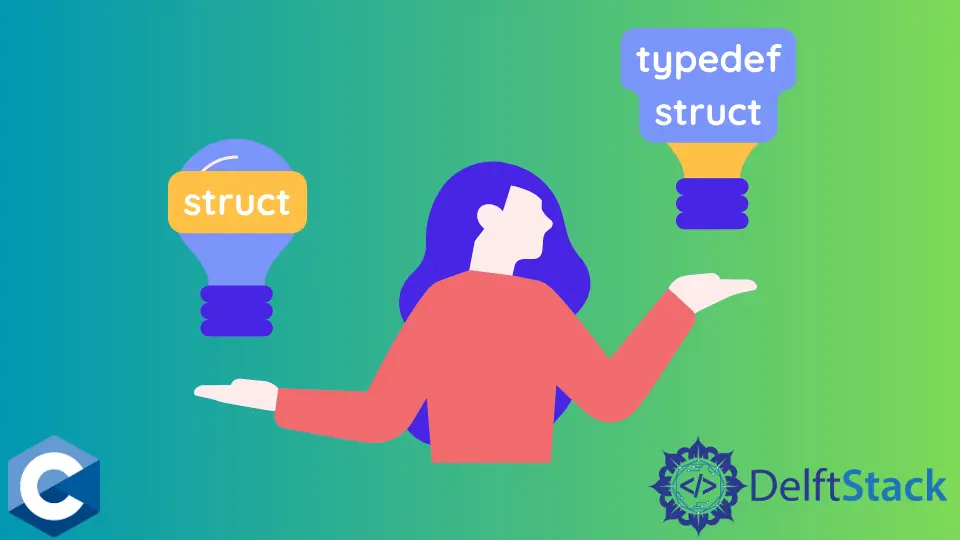
Dieses Tutorial erklärt und demonstriert anhand von Codebeispielen den Unterschied zwischen struct
und typedef struct
in der C-Programmierung.
Struktur in C
Bei der Verwendung von Arrays definieren wir den Typ der Variablen, der viele Datenelemente des gleichen Typs/der gleichen Art enthält. In ähnlicher Weise haben wir einen anderen benutzerdefinierten Datentyp in der C-Programmierung, der als Struktur bekannt ist und uns verschiedene Arten von Datenelementen kombinieren lässt.
Wir können einen Datensatz auch mithilfe von Strukturen darstellen. Beispielsweise können wir die Details der Person verfolgen, wobei jede Person die folgenden Attribute hat:
- Vorname
- Familienname, Nachname
- Geschlecht
- Alter
- Handy, Mobiltelefon
- Adresse
Unterschied zwischen struct
und typedef struct
in C
Wir können eine Struktur mit struct
und typedef struct
definieren, aber mit dem Schlüsselwort typedef
können wir alternative Namen für die benutzerdefinierten Datentypen (z. B. struct) und primitive Datentypen (z. B. int) schreiben.
Das Schlüsselwort typedef
erstellt einen brandneuen Namen für einen bereits vorhandenen Datentyp, erstellt jedoch nicht den neuen Datentyp. Wir können einen saubereren und besser lesbaren Code haben, wenn wir das typedef struct
verwenden, und es erspart uns (dem Programmierer) auch Tastatureingaben.
Das typedef struct
kann das Deklarieren von Variablen vereinfachen und den äquivalenten Code mit vereinfachter Syntax bereitstellen. Dies kann jedoch zu einem unübersichtlicheren globalen Namensraum führen, was bei umfangreicheren Programmen zu Problemen führen kann.
Lassen Sie uns einen Beispielcode für beide Szenarien (das struct
und das typedef struct
) haben und den Unterschied verstehen.
Beispielcode ohne das Schlüsselwort typedef
#include <stdio.h>
struct Books {
int id;
char author[50];
char title[50];
};
int main() {
// declare `book1` and `book2` of type `Books`
struct Books book1;
struct Books book2;
// the specifications for the `book1`
book1.id = 1;
strcpy(book1.author, "Zara Ali");
strcpy(book1.title, "Tutorials for C Programming");
// the specifications for the `book2`
book2.id = 2;
strcpy(book2.author, "Zaid Ali");
strcpy(book2.title, "Tutorials for Java Programming");
// display information for `book1` and `book2`
printf("The id of book1: %d\n", book1.id);
printf("The author of the book1: %s\n", book1.author);
printf("The title of the book1: %s\n", book1.title);
printf("The id of book2: %d\n", book2.id);
printf("The author of the book2: %s\n", book2.author);
printf("The title of the book2: %s\n", book2.title);
return 0;
}
Ausgang:
The id of book1: 1
The author of the book1: Zara Ali
The title of the book1: Tutorials for C Programming
The id of book2: 2
The author of the book2: Zaid Ali
The title of the book2: Tutorials for Java Programming
Der obige Code hat zwei Variablen, book1
und book2
, vom Typ Books
. Wir müssen immer wieder struct
eingeben, wenn wir mehr Variablen deklarieren müssen, z. B. book3
, book4
usw.
Hier kommt das typedef struct
ins Spiel. Siehe das folgende Code-Snippet, das die Verwendung von typedef struct
zeigt.
Beispielcode mit dem Schlüsselwort typedef
Wir können die typedef
in den folgenden zwei Methoden verwenden.
Methode 1:
#include <stdio.h>
struct Books {
int id;
char author[50];
char title[50];
};
typedef struct Books Book;
int main() {
// declare `book1` and `book2` of type `Book`
Book book1;
Book book2;
// the specifications for the `book1`
book1.id = 1;
strcpy(book1.author, "Zara Ali");
strcpy(book1.title, "Tutorials for C Programming");
// the specifications for the `book2`
book2.id = 2;
strcpy(book2.author, "Zaid Ali");
strcpy(book2.title, "Tutorials for Java Programming");
// display information for `book1` and `book2`
printf("The id of book1: %d\n", book1.id);
printf("The author of the book1: %s\n", book1.author);
printf("The title of the book1: %s\n", book1.title);
printf("The id of book2: %d\n", book2.id);
printf("The author of the book2: %s\n", book2.author);
printf("The title of the book2: %s\n", book2.title);
return 0;
}
Ausgang:
The id of book1: 1
The author of the book1: Zara Ali
The title of the book1: Tutorials for C Programming
The id of book2: 2
The author of the book2: Zaid Ali
The title of the book2: Tutorials for Java Programming
Methode 2:
#include <stdio.h>
typedef struct Books {
int id;
char author[50];
char title[50];
} Book;
int main() {
// declare `book1` and `book2` of type `Book`
Book book1;
Book book2;
// the specifications for the `book1`
book1.id = 1;
strcpy(book1.author, "Zara Ali");
strcpy(book1.title, "Tutorials for C Programming");
// the specifications for the `book2`
book2.id = 2;
strcpy(book2.author, "Zaid Ali");
strcpy(book2.title, "Tutorials for Java Programming");
// display information for `book1` and `book2`
printf("The id of book1: %d\n", book1.id);
printf("The author of the book1: %s\n", book1.author);
printf("The title of the book1: %s\n", book1.title);
printf("The id of book2: %d\n", book2.id);
printf("The author of the book2: %s\n", book2.author);
printf("The title of the book2: %s\n", book2.title);
return 0;
}
Ausgang:
The id of book1: 1
The author of the book1: Zara Ali
The title of the book1: Tutorials for C Programming
The id of book2: 2
The author of the book2: Zaid Ali
The title of the book2: Tutorials for Java Programming
Die zweite Methode zeigt, dass die Verwendung von typedef
organisierter, sauberer und einfacher zu verstehen und zu verwalten erscheint.
Wichtige Punkte bei der Verwendung des Schlüsselworts typedef
Bei der Verwendung des Schlüsselworts typedef
müssen wir einige Punkte beachten. In dem oben angegebenen Code definieren wir die Struktur wie folgt.
typedef struct Books {
int id;
char author[50];
char title[50];
} Book;
Hier haben wir einen neuen Strukturtyp, struct Books
, und den Alias namens Book
für die struct Books
. Sie fragen sich vielleicht, warum der Aliasname mit dem Zusatz typedef
wie bei jeder anderen gewöhnlichen Deklaration am Ende der Deklaration steht.
typedef
wurde in die exakte Spezifiziererkategorie wie die Speicherklassenspezifizierer eingefügt, zum Beispiel auto
und static
. Wir können auch ohne den Tag-Namen deklarieren, wenn wir nur die Typkennung Book
verwenden wollen.
typedef struct {
int id;
char author[50];
char title[50];
} Book;
Bei der Verwendung von Strukturen müssen wir uns um das kümmern, was wir eine Variable und einen Alias nennen sollten. Wenn wir die anonyme Struktur wie folgt verwenden (anonym, weil die Struktur keinen Namen hat), dann wäre das Buch
die Strukturvariable des benutzerdefinierten Datentyps.
struct {
int id;
char author[50];
char title[50];
} Book;
Wenn wir dieselbe Struktur wie oben verwenden, aber mit dem Schlüsselwort typedef
, dann wäre das Book
ein Alias (Synonym) für die Datentypstruktur, und wir können damit Variablen deklarieren (siehe unten).
typedef struct {
int id;
char author[50];
char title[50];
} Book;