How to Convert Integer to String Data Type in Microsoft Excel VBA
- What Is a Data Type
-
the
Integer
Data Type in VBA -
the
String
Data Type in VBA - Conversion of Data Types
-
How to Convert From
Integer
toString
Data Type in VBA - Conclusion
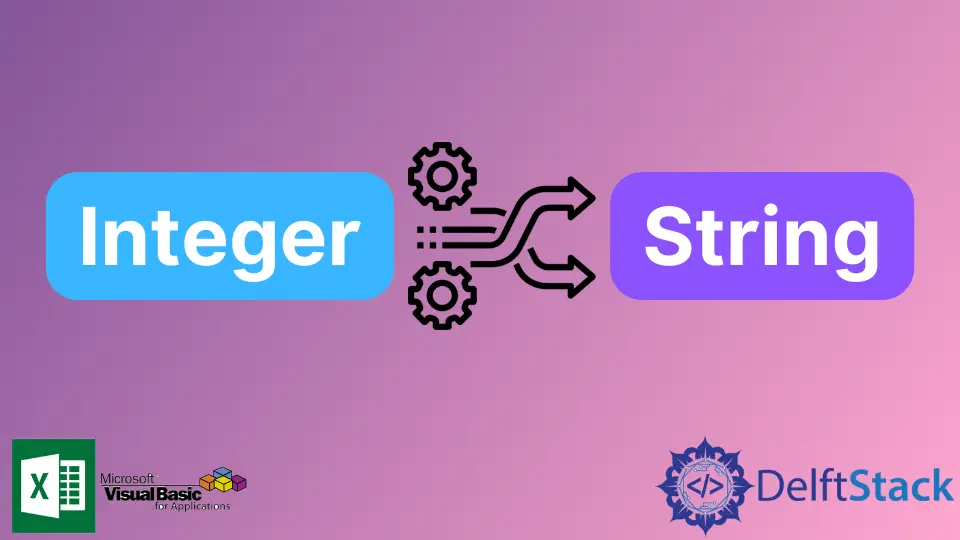
VBA is short for Visual Basic for Applications
. It is a programming language for all Microsoft Office applications, such as Excel, Word, and PowerPoint.
Using VBA allows the users to optimize their time on the Microsoft Office application by writing codes, known as Macros
. This way, the user can run the Macro for a task to make it automated instead of manually performing the same actions repeatedly, saving time.
VBA provides developers with a variety of different functions and features. In this article, we will learn about converting the Integer
data type to the String
data type.
But first, let us give you a basic introduction to these data types in VBA.
What Is a Data Type
A data type is a classification of the types of variables having different types of values and different operations allowed on them. A data type gives context to any data and helps the compiler understand the data’s behavior.
There are many different data types like Integer
, Character
, Boolean
, String
, etc.
the Integer
Data Type in VBA
As the name suggests, this data type is assigned to any variable you want to store numeric integer values like 1
, -3
, 100
, etc. It stores non-fractional values.
This data type takes 2
bytes of memory with values ranging from -32,768
to 32,767
.
Here is the syntax to declare an Integer
type variable.
Dim X As Integer 'where X will be the Integer type variable
the String
Data Type in VBA
A string is a sequence of characters. A character can be an alphabet, numeric, or special character.
So, any text can be stored in the String
type variable. It has two types, namely variable length and fixed variable length.
Variable Length String
In a variable length String
, 10
bytes are added to the string length, and the range is from 0
to 2
billion characters. The syntax to declare this data type is below.
Dim strName As String 'where strName will be the string type variable
Fixed Variable Length String
It has a fixed length defined by the user, ranging from 1
to 65,400
characters. Here is the syntax to declare this data type in VBA.
Dim strName As String * 20 'where strName will be the string type variable
Conversion of Data Types
Conversion of data types means changing from one data type to another. For example, the conversion from a floating-point float
to an integer
may be implicit or explicit.
Implicit conversion is when the data types are compatible, like float
to integer
. Such conversions happen automatically.
Explicit conversion is when the data types are not compatible, like integer
to string
. Such data types are converted manually by the user.
How to Convert From Integer
to String
Data Type in VBA
Now that you know the basics about data types and conversion, the question is how can we convert from an Integer
to a String
in VBA? The simple answer is the function CStr(expression)
, where expression
is the Integer
value we are trying to change to String
.
Like any other language, VBA allows us to use functions. Functions are procedures that return us a value after performing certain operations on the data.
All languages, including VBA, provide us with some built-in functions from already existing libraries.
CStr(expression)
is also a built-in function that returns a String
type value. Arguments include any expression.
When any data type expression is passed through parameters, the function converts and returns a String
type value. Following are some cases of the expression passed to the CStr(expression)
function.
Empty
: If nothing is passed in parameters, i.e., theCStr()
code is run, then the function will return an emptyString
, i.e.," "
.Boolean
: AString
containingTrue
orFalse
will be returned if aboolean
is passed toCStr()
.Numeric Value
: AString
containing that number will be returned.Date
: AString
containing the date will be returned, which means we will have the date in text form now.
It is obvious from the above discussion that to convert an Integer
to a String
data type; we need to pass the Integer
value to the CStr(expression)
function. Let’s see a few examples to further understand this conversion.
Example 1
Sub example1()
Dim intX As Integer
Dim ReturnedValue As String
intX = 23
ReturnedValue = CStr(intX)
MsgBox ReturnedValue
End Sub
In this example, we first declared an Integer
type variable intX
and later assigned it a value of 23
. A String
type variable ReturnedValue
was also declared at the start.
CStr()
function call is then made with intX
passed as a parameter. The returned value is stored in the ReturnedValue
variable.
Now the ReturnedValue
contains the text "23"
, instead of the numeric value. In the output, 23
will be displayed through the MsgBox
command.
Example 2
Sub Example2()
Dim ReturnedValue As String
ReturnedValue = CStr(1000)
MsgBox ReturnedValue
End Sub
The Integer
value is passed directly instead of a variable in this example. After the CStr()
function call, "1000"
is now stored in the ReturnedValue
variable as a String
.
Output 1000
will be displayed through the MsgBox
command.
Conclusion
VBA allows us to use functions to perform some operations on our data. Conversion from one data type to another is a useful operation.
For example, we can convert any data type to the String
type to display the value through the MsgBox
command, which only takes the String
type prompt.
In this article, we explained what data types are and what we mean by conversion between them. We learned the conversion from the Integer
data type to the String
data type using the CStr(expression)
function and saw a couple of examples to understand the use of the CStr(expression)
function.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub