How to Use Excel VBA to Run SQL Query
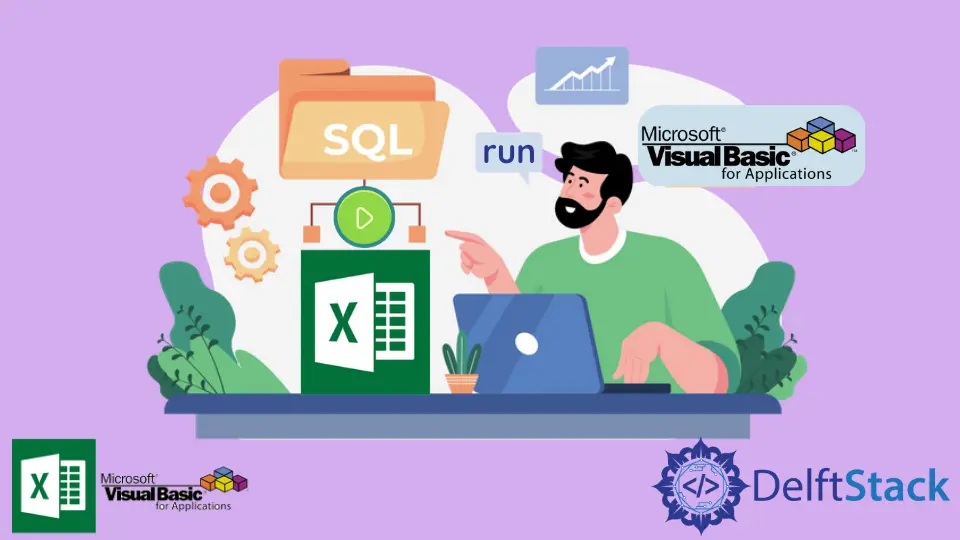
One of the strong capabilities of Excel is data processing and data visualization. When it comes to data, Structured Query Language (SQL) is very useful as it is the main programming language used to fetch data from the database.
Normally, SQL programs have built-in data output interfaces where you can view the results of your queries. However, no one of these data output interfaces will match the level of flexibility and functionality that Excel could deliver.
In this tutorial, we will combine the data extraction capability of SQL and the data processing prowess of Excel. This scheme is typically used in industries where data centers are established, and the need for quick and easy data extraction is inevitable.
The code below connects Excel with the SQL server using an ADO
object which allows connection through a remote data source. With this object, VBA can access and manipulate the database.
However, the ADODB
object does not come automatically with the default library used by VBA. To enable the ADODB
object, we need to enable it in the References
toolbar.
To do this, please follow the steps below.
-
Open Excel file.
-
From the
Developer
tab, open theVisual Basic
Editor. -
From the
Tools
toolbar, clickReferences
. -
Tick the
Microsoft ActiveX Data Objects 2.8 Library
checkbox.
You are now all set.
The code block below has eight parameters; refer to the table below:
Parameter | Description |
---|---|
Sql |
Sql script to run |
nRow |
the row where to return the data extracted |
nCol |
the column where to return the data extracted |
sheetDes |
the worksheet where to return the data extracted |
usrID |
username to access in the database |
pssWrd |
password of the username to access in the database |
sidStr |
SID to be used |
hst |
hostname to be used |
Sub getData(Sql As String, nRow As Integer, nCol As Integer, sheetDes As String, usrID as String, pssWrd as String, sidStr as string, hst as String)
Dim Connct As ADODB.Connection
Dim RcrdSet As ADODB.Recordset
Dim RcrdVal As Variant
Dim reference_x As Integer
Dim reference_y As Integer
Dim records_count As Integer
'CONNECTION STRING
connection_string = "(DESCRIPTION = (ADDRESS = (PROTOCOL = TCP)(HOST = hst)(PORT = 1521))(CONNECT_DATA = (SID = sidStr)))"
user_id = usrID
pass_word = pssWrd
'EXCEL PRINT LOCATION
reference_col = nCol
reference_row = nRow
' start ------ default connection setup & SQL execution code (Do not Edit entries)
cs = "Provider=OraOLEDB.Oracle;Data Source=" & connection_string & ";User Id=" & user_id & ";Password=" & pass_word & ";"
Set Connct = New ADODB.Connection
Set RcrdSet = New ADODB.Recordset
With Connct
.CursorLocation = adUseClient
.Open cs
.CommandTimeout = 0
RcrdSet.CursorType = asOpenForwardOnly
RcrdSet.Open (Sql), Connct
records_count = CInt(RcrdSet.RecordCount)
' end ------ default connection setup & SQL execution code (Do not Edit entries)
' start ------ default data print setup & close procedures (Do not Edit)
If records_count > 0 Then
RcrdSet.MoveFirst
For x = 0 To RcrdSet.Fields.Count - 1
ThisWorkbook.Sheets(sheetDes).Cells(reference_row, x + reference_col) = RcrdSet.Fields(x).Name
Next
ThisWorkbook.Sheets(sheetDes).Cells(reference_row + 1, reference_col).CopyFromRecordset RcrdSet
End If
End With
RcrdSet.Close
Connct.Close
Set RcrdSet = Nothing
Set Connct = Nothing
'end ------ default data print setup & close procedures (Do not Edit)
End Sub
Now, suppose we have the following information about your database and the database connection:
Host: database1
SID: database1@server.com
Username: username123
Password: pw123
database1
, table1
content:
| Names | Age | Gender | Sports |
|------------|----------|--------------|--------------|
| Juan | 17 | Male | Chess |
| Pedro | 19 | Male | Badminton |
| Maria | 25 | Female | Volleyball |
| Rodolfo | 29 | Male | Basketball |
| Cathy | 18 | Female | Chess |
| Michelle | 21 | Female | Swimming |
| Glen | 24 | Male | Billiards |
SQL Query:
Select Names, Gender, Sports
from table1@database1
where Age <= 25
To execute this SQL query and output the entries on Sheet1
on column 1 and row 1 of an Excel workbook, use the code block below:
Sub getData(Sql As String, nRow As Integer, nCol As Integer, sheetDes As String, usrID As String, pssWrd As String, sidStr As String, hst As String)
Dim Connct As ADODB.Connection
Dim RcrdSet As ADODB.Recordset
Dim RcrdVal As Variant
Dim reference_x As Integer
Dim reference_y As Integer
Dim records_count As Integer
'CONNECTION STRING
connection_string = "(DESCRIPTION = (ADDRESS = (PROTOCOL = TCP)(HOST = hst)(PORT = 1521))(CONNECT_DATA = (SID = sidStr)))"
user_id = usrID
pass_word = pssWrd
'EXCEL PRINT LOCATION
reference_col = nCol
reference_row = nRow
' start ------ default connection setup & SQL execution code (Do not Edit entries)
cs = "Provider=OraOLEDB.Oracle;Data Source=" & connection_string & ";User Id=" & user_id & ";Password=" & pass_word & ";"
Set Connct = New ADODB.Connection
Set RcrdSet = New ADODB.Recordset
With Connct
.CursorLocation = adUseClient
.Open cs
.CommandTimeout = 0
RcrdSet.CursorType = asOpenForwardOnly
RcrdSet.Open (Sql), Connct
records_count = CInt(RcrdSet.RecordCount)
' end ------ default connection setup & SQL execution code (Do not Edit entries)
' start ------ default data print setup & close procedures (Do not Edit)
If records_count > 0 Then
RcrdSet.MoveFirst
For x = 0 To RcrdSet.Fields.Count - 1
ThisWorkbook.Sheets(sheetDes).Cells(reference_row, x + reference_col) = RcrdSet.Fields(x).Name
Next
ThisWorkbook.Sheets(sheetDes).Cells(reference_row + 1, reference_col).CopyFromRecordset RcrdSet
End If
End With
RcrdSet.Close
Connct.Close
Set RcrdSet = Nothing
Set Connct = Nothing
'end ------ default data print setup & close procedures (Do not Edit)
End Sub
Sub testSQLVBAConnection()
Dim sqlStr As String
sqlStr = "Select Names, Gender, Sports "
sqlStr = sqlStr & " from table1@database1 "
sqlStr = sqlStr & " where Age <= 25 "
Call getData(sqlStr, 1, 1, "Sheet1", "username123", "pw123", "database1@server.com", "database1")
End Sub
testSQLVBAConnection
Output:
| Names | Gender | Sports |
|------------|--------------|--------------|
| Juan | Male | Chess |
| Pedro | Male | Badminton |
| Maria | Female | Volleyball |
| Cathy | Female | Chess |
| Michelle | Female | Swimming |