How to Add New Line in VBA
- Add New Line in VBA
-
Use the
vbNewLine
Method to Add New Line in VBA -
Use the
Chr(10)
Method to Add New Line in VBA
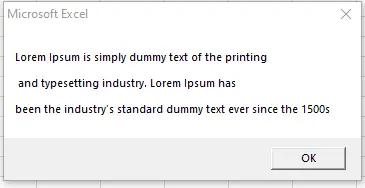
We will introduce how to continue code in the next line in VBA with an example. We will also introduce how to go to the next line in the message box with different methods in VBA.
Add New Line in VBA
There are two different types of situations in programming when we think about how to go to the next line without breaking the code. First is when a code in a certain line exceeds the screen size of the line.
The other method is when we have to display something in a message box, and we want to break the line after a certain point. If we want to keep coding in multiple lines using VBA, it provides a simple method that can be used.
We need to add space, put underscore(_
), and start the new line. It will take both lines as one line, and there won’t be any errors.
Example Code:
# VBA
Sub test()
MsgBox "Lorem Ipsum is simply dummy text of the printing" _
& " and typesetting industry. Lorem Ipsum has " _
& "been the industry's standard dummy text ever since the 1500s"
End Sub
Output:
From the above example, there’s no change in the message box, and it is because the underscore(_
) is only used to continue the code to the next line. The code was executed without any problem, which means that the underscore has taken the multiple lines as a single line.
Use the vbNewLine
Method to Add New Line in VBA
Suppose we want to add the text to a new line or add a line break in the message box or in the content we are displaying or saving in the excel file. Many different methods can be used for this purpose.
The first method used is vbNewLine
, and we can add it anywhere in the line where we want to add a break.
Example Code:
# VBA
Sub test()
MsgBox "Lorem Ipsum is simply dummy text of the printing" & vbNewLine & " and typesetting industry. Lorem Ipsum has " & vbNewLine & "been the industry's standard dummy text ever since the 1500s"
End Sub
Output:
It is displayed as we wanted it, but we used the msgBox only in one line. We can also use this method to add multiple new lines by using this method multiple times, as shown below.
Example Code:
# VBA
Sub test()
MsgBox "Lorem Ipsum is simply dummy text of the printing" & vbNewLine & " and typesetting industry. Lorem Ipsum has " & vbNewLine & "been the industry's standard dummy text ever since the 1500s"
End Sub
Output:
Using the vbNewLine
method multiple times will add multiple lines to the message box.
Use the Chr(10)
Method to Add New Line in VBA
Let’s discuss another method that can be used to achieve the same thing, known as Chr(10)
. We can use this method the same way we used the vbNewLine
method.
Example Code:
# VBA
Sub test()
MsgBox "Lorem Ipsum is simply dummy text of the printing" & Chr(10) & " and typesetting industry. Lorem Ipsum has " & Chr(10) & "been the industry's standard dummy text ever since the 1500s"
End Sub
Output:
The chr(10)
method is used the same way as the vbNewLine
method, giving the same results.