How to Exit Sub in VBA
- Understanding Exit Sub in VBA
- Basic Example of Exit Sub
- Using Exit Sub with Error Handling
- Conditional Exit Sub
- Conclusion
- FAQ
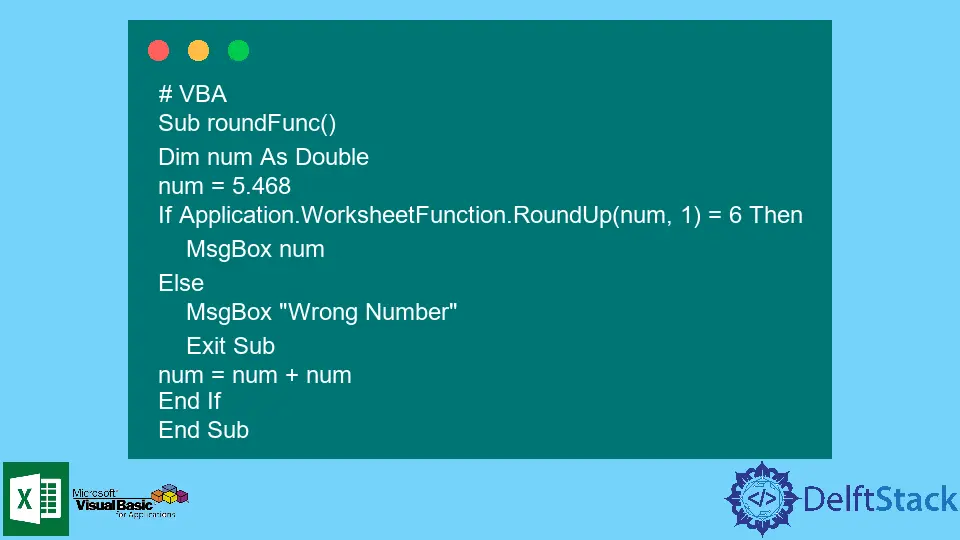
When working with Visual Basic for Applications (VBA), managing code execution flow is crucial for creating efficient programs. One important statement that helps control this flow is the “Exit Sub” statement. This command allows you to exit a subroutine prematurely, which can be particularly useful in error handling or when certain conditions are met.
In this article, we will demonstrate how to use “Exit Sub” in VBA, providing clear examples and explanations to enhance your understanding. Whether you are a beginner or an experienced developer, mastering this concept will improve your coding skills and streamline your workflows.
Understanding Exit Sub in VBA
The “Exit Sub” statement is a powerful tool in VBA that allows you to exit a subroutine before it reaches the end. This can be beneficial in various scenarios, such as when an error occurs or when specific conditions are met. By using “Exit Sub,” you can prevent unnecessary code execution, making your program more efficient and user-friendly.
Using “Exit Sub” is straightforward. When the VBA interpreter encounters this statement, it immediately stops executing the current subroutine and returns control to the calling procedure or the next line of code after the subroutine call. This functionality allows for greater control over your code’s behavior, especially in complex applications.
Basic Example of Exit Sub
Let’s look at a simple example to illustrate how “Exit Sub” works in a VBA subroutine.
Sub ExampleExitSub()
Dim x As Integer
x = 10
If x > 5 Then
Exit Sub
End If
MsgBox "This message will not be displayed."
End Sub
In this example, we have a subroutine called ExampleExitSub
. The variable x
is assigned a value of 10. The If
statement checks if x
is greater than 5. Since this condition is true, the Exit Sub
statement is executed, and the subroutine terminates immediately. As a result, the message box will not be displayed.
This example demonstrates how “Exit Sub” can effectively control the flow of your code. By placing this statement strategically, you can prevent unnecessary actions from occurring.
Using Exit Sub with Error Handling
One of the most common uses for “Exit Sub” is in error handling. By incorporating this statement into your error handling routines, you can ensure that your code exits gracefully when an error occurs.
Sub ErrorHandlingExample()
On Error GoTo ErrorHandler
Dim result As Double
result = 10 / 0 ' This will cause a division by zero error
Exit Sub
ErrorHandler:
MsgBox "An error occurred: " & Err.Description
End Sub
In this example, we have a subroutine that attempts to perform a division operation. However, dividing by zero will trigger an error. The On Error GoTo ErrorHandler
statement tells VBA to jump to the ErrorHandler
label when an error occurs. If an error is encountered, the code displays a message box with the error description. The Exit Sub
statement ensures that the code exits the subroutine before reaching the error handler if no error occurs.
Output:
An error occurred: Division by zero
By utilizing “Exit Sub” in conjunction with error handling, you can create robust VBA applications that respond appropriately to unexpected situations.
Conditional Exit Sub
Another effective way to use “Exit Sub” is in conjunction with conditional statements. This allows you to exit a subroutine based on specific criteria.
Sub ConditionalExitExample()
Dim userInput As String
userInput = InputBox("Please enter a number:")
If Not IsNumeric(userInput) Then
MsgBox "Invalid input. Exiting subroutine."
Exit Sub
End If
MsgBox "You entered: " & userInput
End Sub
In this example, we prompt the user to enter a number. The If Not IsNumeric(userInput)
statement checks if the input is not a number. If the input is invalid, a message box informs the user, and the subroutine exits immediately using “Exit Sub.” If the input is valid, the subroutine proceeds to display the entered number.
Output:
Invalid input. Exiting subroutine.
This method demonstrates how you can enhance user experience by validating input and exiting the subroutine when necessary.
Conclusion
Understanding how to use “Exit Sub” in VBA is essential for managing code execution flow effectively. By employing this statement in various scenarios, such as error handling and conditional checks, you can create more efficient and user-friendly applications. These techniques will not only improve your coding skills but also enhance the overall performance of your VBA projects. With practice, you’ll find that mastering “Exit Sub” will significantly streamline your coding process.
FAQ
-
What is the purpose of Exit Sub in VBA?
Exit Sub is used to terminate a subroutine prematurely, allowing for better control over code execution. -
Can Exit Sub be used in conjunction with error handling?
Yes, Exit Sub is often used in error handling routines to ensure that the code exits gracefully when an error occurs. -
How does Exit Sub affect the flow of a VBA program?
When Exit Sub is encountered, the subroutine stops executing immediately, returning control to the calling procedure. -
Can I use multiple Exit Sub statements in one subroutine?
Yes, you can use multiple Exit Sub statements in a single subroutine, but only the first one encountered will execute. -
What happens if I don’t use Exit Sub in a long-running subroutine?
Without Exit Sub, the subroutine will continue executing until it reaches the end, which may lead to unnecessary processing and slower performance.