How to Add Delay During Code Execution Using VBA
- Method 1: Using the Sleep Function
- Method 2: Using Application.Wait
- Method 3: Using a Loop with DoEvents
- Conclusion
- FAQ
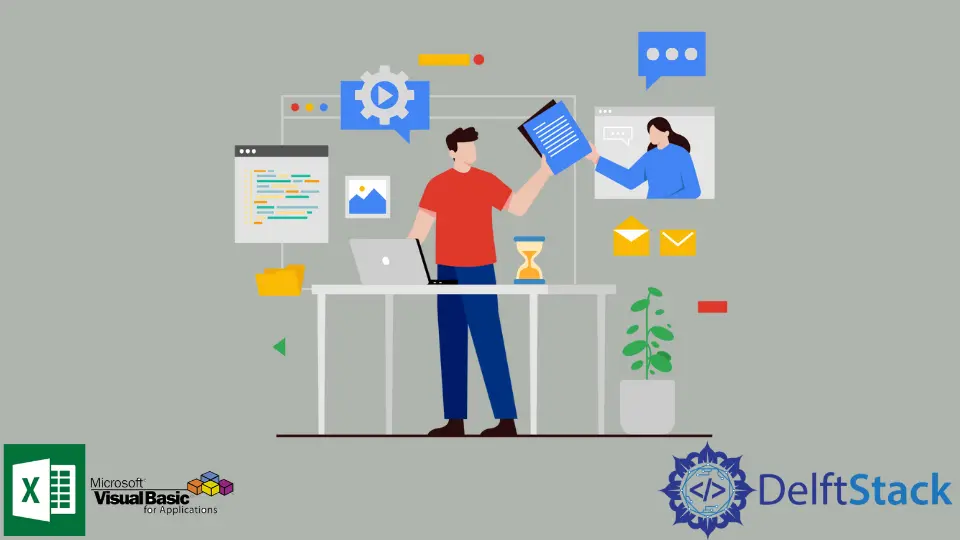
Adding a delay during code execution can be crucial in various programming scenarios, especially when working with user interfaces or when you need to wait for an external process to complete.
In this article, we will explore how to implement delays in VBA (Visual Basic for Applications), the programming language commonly used in Microsoft Office applications. Whether you’re developing an Excel macro or automating tasks in Word, understanding how to introduce pauses can enhance user experience and improve the efficiency of your code. Let’s dive into the different methods to achieve this.
Method 1: Using the Sleep Function
The Sleep function is a straightforward way to add a delay in VBA. This function pauses the execution of your code for a specified number of milliseconds. To use it, you need to declare the Sleep function from the Windows API at the beginning of your module. Here’s how you can do it:
Declare Sub Sleep Lib "kernel32" (ByVal dwMilliseconds As Long)
Sub DelayExample()
MsgBox "The code will pause for 5 seconds."
Sleep 5000
MsgBox "5 seconds have passed."
End Sub
In this example, we first declare the Sleep function, allowing us to call it within our VBA code. The DelayExample
subroutine shows a message box, pauses for 5000 milliseconds (or 5 seconds), and then displays another message box. This method is particularly useful for creating pauses in your code without blocking the entire application.
Method 2: Using Application.Wait
Another effective way to introduce delays in your VBA code is by using the Application.Wait
method. This method allows you to pause the execution until a specific time. Here’s how it works:
Sub WaitExample()
MsgBox "The code will pause for 10 seconds."
Application.Wait (Now + TimeValue("0:00:10"))
MsgBox "10 seconds have passed."
End Sub
In this code, we display a message box to inform the user that the code will pause. The Application.Wait
method takes a time value as its argument. In this case, we specify a 10-second delay by adding 10 seconds to the current time using Now + TimeValue("0:00:10")
. After the wait, another message box appears to indicate that the delay has ended. This method is ideal for scenarios where you want to wait until a specific time of day or duration.
Method 3: Using a Loop with DoEvents
If you want to create a delay while still allowing other processes to run, you can use a loop combined with the DoEvents
function. This method is a bit more complex but provides flexibility. Here’s an example:
Sub LoopDelayExample()
Dim endTime As Double
endTime = Timer + 5 ' 5 seconds delay
MsgBox "The code will pause for 5 seconds."
Do While Timer < endTime
DoEvents
Loop
MsgBox "5 seconds have passed."
End Sub
In this example, we first set a variable endTime
to the current time plus 5 seconds using the Timer
function. We then enter a loop that continues until the current time exceeds endTime
. The DoEvents
function allows the application to process other events while waiting, ensuring that the user interface remains responsive. This approach is particularly useful in long-running processes where you want to keep the application responsive to user actions.
Conclusion
Adding delays during code execution in VBA can significantly improve the user experience and the functionality of your applications. By using methods like the Sleep function, Application.Wait, or a loop with DoEvents, you can effectively manage the timing of your code execution. Each method has its unique advantages, so choose the one that best fits your specific needs. With these techniques, you can create smoother and more efficient VBA applications that enhance productivity and user satisfaction.
FAQ
-
What is the Sleep function in VBA?
The Sleep function is a Windows API function that pauses the execution of code for a specified number of milliseconds. -
How does Application.Wait work in VBA?
Application.Wait pauses code execution until a specified time, allowing you to set delays based on the current time. -
Can I use a loop to create a delay in VBA?
Yes, using a loop with DoEvents allows you to create a delay while keeping the application responsive to user actions. -
What are the advantages of using delays in VBA?
Delays can improve user experience, allow time for processes to complete, and ensure that the application remains responsive. -
Are there any limitations to using delays in VBA?
Excessive use of delays can lead to unresponsive applications or poor performance, so it’s essential to use them judiciously.