How to Check for Undefined in TypeScript
- Undefined vs Null in TypeScript
- Strict Undefined Check in Typescript Using “===”
-
Check Undefined in Typescript Using
==
-
Check Null Instead of
Undefined
in TypeScript - Check Undefined in Typescript at Root Level
- Juggling-Check for Undefined and Null in Typescript
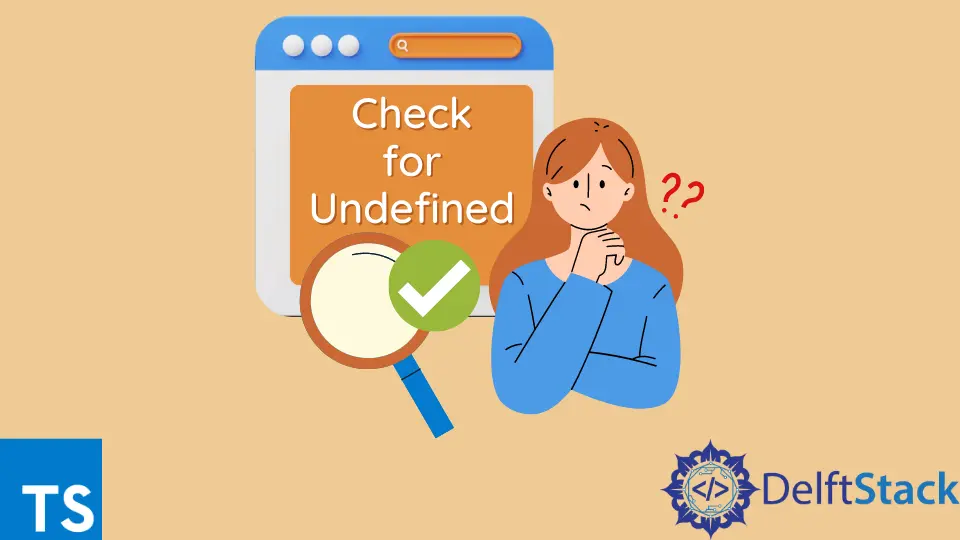
This tutorial will demonstrate how a programmer could check undefined in TypeScript with various coding examples and situations. It not only just gives you an idea of checking undefined in TypeScript but also helps in differentiating between null and undefined. First, let us see the main difference between the undefined and null.
Undefined vs Null in TypeScript
Like JavaScript, its extension TypeScript has two bottom types null and undefined. They both are intended to define different things.
- Hasn’t been initialized:
undefined
. - Currently unavailable:
null
.
Strict Undefined Check in Typescript Using “===”
In JavaScript, as well as in its extended form TypeScript, validating the variable using ===
will check the value its type as well as its value.
let userEmail:string|undefined;
if(userEmail===undefined)
{
alert('User Email is undefined');
}else{
alert(`User Email is ${userEmail}`);
}
The first line sets the data type of variable userEmail
as either string or undefined. After setting the datatype, it validates the variable in the if
condition. ===
in TypeScript will allow checking both the variable type as well as its value and performs the desired operation after the validation. If the userEmail
is assigned with the string value, the output will be following:
Else if it is not assigned with the value, it will be undefined and will be detected first if checked and displays the output as:
Check Undefined in Typescript Using ==
Rather than using ===
for the undefined checking in TypeScript, you could also use ==
, which check only the value.
let userEmail:string|undefined;
if(userEmail==undefined)
{
alert('User Email is undefined');
}else{
alert(`User Email is ${userEmail}`);
}
This will generate the same output as above in the previous example.
Check Null Instead of Undefined
in TypeScript
In TypeScript, you could also check undefined using null in the if condition in place of undefined; this will also return true if something is undefined plus will return true if null. It will be done using ==
in the condition because ===
checks both the type and value and will give an error because of the reason that null is not equal to undefined in type.
let userEmail:string|undefined;
if(userEmail==null)
{
alert('User Email is undefined');
}else{
alert(`User Email is ${userEmail}`);
}
If ===
is used, then the output will be below.
Check Undefined in Typescript at Root Level
If you use ==
at the root level for the undefined checking in TypeScript, and the variable is undefined, you get a ReferenceError
exception and the whole call stack unwinds. So for the checking, if the variable is undefined or not at the root level, typeof
is suggested.
let globalData:string|undefined;
if (typeof globalData == 'undefined')
{
alert(`globalData is ${globalData}`);
}
This solution is suggested in the open-source book for TypeScript Basarat Typescript Deep Dive.
Juggling-Check for Undefined and Null in Typescript
As ==
only checks the value instead of the type, and if we use null in the if condition for undefined checking in TypeScript, it will execute the same operation for the null. So to avoid this, we use a Juggling check which will perform the desired operation on the desired type.
var variableOne: any;
var variableTwo: any = null;
function typeCheck(x:any, name:any) {
if (x == null) {
console.log(name + ' == null');
}
if (x === null) {
console.log(name + ' === null');
}
if (typeof x === 'undefined') {
console.log(name + ' is undefined');
}
}
typeCheck(variableOne, 'variableOne');
typeCheck(variableTwo, 'variableTwo');
The first if
statement will be executed for both the undefined
and the null
, the second and third condition checks the type, and the matched type value does the given operation. This code performs both the undefined check and the null check in TypeScript.
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn