How to Write console.log Wrapper for Angular 2 in TypeScript
- Understanding the Need for a Logging Wrapper
- Creating the Logging Service
- Using the Logging Service in Components
- Conclusion
- FAQ
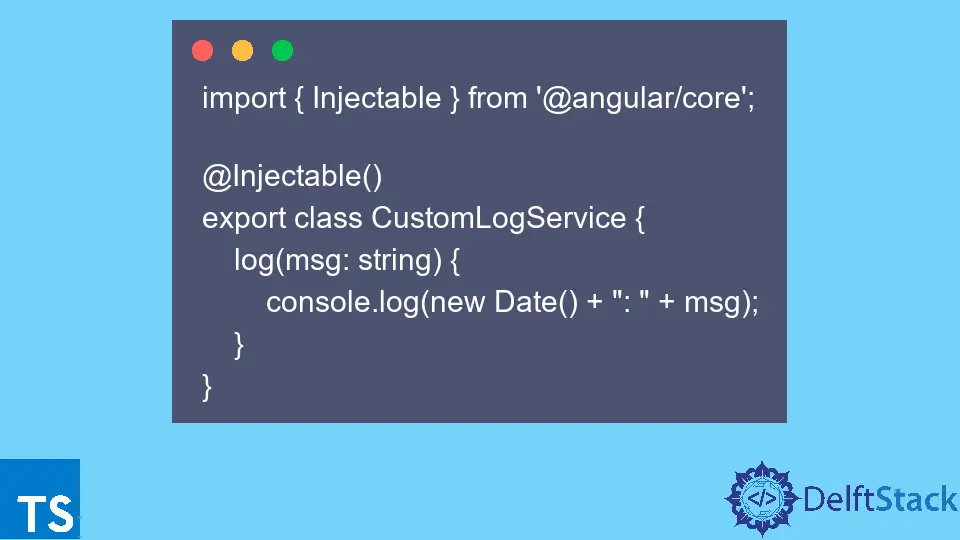
Logging is an essential part of any application development process, especially when you’re working with frameworks like Angular 2. It helps developers track down issues, monitor application behavior, and maintain code quality.
In this tutorial, we will explore how to create a simple console.log
wrapper in Angular 2 using TypeScript. This approach not only improves the readability of your logging but also allows for easier modifications in the future. By the end of this article, you’ll have a solid understanding of how to implement a logging service that can be utilized throughout your Angular application. So, let’s dive in!
Understanding the Need for a Logging Wrapper
When developing an Angular application, you might find yourself using console.log
statements frequently for debugging. However, relying solely on these statements can lead to cluttered code and make it challenging to manage logging effectively. A logging wrapper allows you to centralize your logging logic, making it easier to switch out the logging method, control the logging level, or even redirect logs to a remote server.
The primary advantage of creating a logging wrapper is the flexibility it offers. For instance, if you decide to switch from console logging to a more sophisticated logging service in the future, you only need to update your wrapper rather than hunt down every console.log
statement in your codebase. This can save time and reduce the risk of introducing bugs during such transitions.
Creating the Logging Service
To create a logging service in Angular 2, you’ll first need to generate a new service using the Angular CLI. This service will contain methods for logging messages at various levels, such as info, warn, and error. Here’s how to do it:
ng generate service logging
This command creates a new service file named logging.service.ts
. Open that file and implement the following code:
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class LoggingService {
logInfo(message: string): void {
console.log(`INFO: ${message}`);
}
logWarning(message: string): void {
console.warn(`WARNING: ${message}`);
}
logError(message: string): void {
console.error(`ERROR: ${message}`);
}
}
In this code, we define a LoggingService
class with three methods: logInfo
, logWarning
, and logError
. Each method prefixes the message with a corresponding label and uses the appropriate console method to log it.
Output:
INFO: This is an info message
WARNING: This is a warning message
ERROR: This is an error message
By using this logging service, you can easily categorize your logs, which helps in filtering and debugging. This centralization also allows you to extend functionality in the future, such as adding timestamps or sending logs to a server.
Using the Logging Service in Components
Once you have created the logging service, the next step is to inject it into your Angular components. This allows you to use the logging methods you just defined. Here’s how to do it:
import { Component } from '@angular/core';
import { LoggingService } from './logging.service';
@Component({
selector: 'app-example',
templateUrl: './example.component.html'
})
export class ExampleComponent {
constructor(private loggingService: LoggingService) {}
performAction() {
this.loggingService.logInfo('Action performed successfully.');
this.loggingService.logWarning('This action may have some side effects.');
this.loggingService.logError('An error occurred during the action.');
}
}
In this example, we inject the LoggingService
into the ExampleComponent
through the constructor. When the performAction
method is called, it logs messages at different levels using the logging service.
Output:
INFO: Action performed successfully.
WARNING: This action may have some side effects.
ERROR: An error occurred during the action.
By utilizing the logging service in your components, you maintain a clean and organized code structure. It also provides a consistent logging mechanism across your application, making it easier to manage logs.
Conclusion
Creating a console.log
wrapper in Angular 2 using TypeScript is a straightforward process that can significantly enhance your logging capabilities. By centralizing your logging logic in a dedicated service, you not only improve code readability but also gain the flexibility to adapt your logging strategy as your application evolves. Whether you’re debugging or monitoring application behavior, a well-structured logging service can save you time and effort. Start implementing this practice today, and watch your Angular applications become more manageable and easier to debug.
FAQ
-
How do I inject the logging service into my component?
You can inject the logging service into your component by adding it to the constructor of your component class. -
Can I extend the logging service to log to an external server?
Yes, you can modify the logging methods to send logs to an external server using HTTP requests. -
What are the benefits of using a logging service?
A logging service centralizes logging logic, making it easier to manage, filter, and modify logging behavior throughout your application. -
Is it possible to customize the logging format?
Absolutely! You can modify the logging methods to include timestamps, log levels, or any other information you find useful. -
Can I use this logging service in other Angular applications?
Yes, you can reuse the logging service across different Angular applications by creating a shared library or module.