TypeScript で Angular 2 の console.log ラッパーを作成する
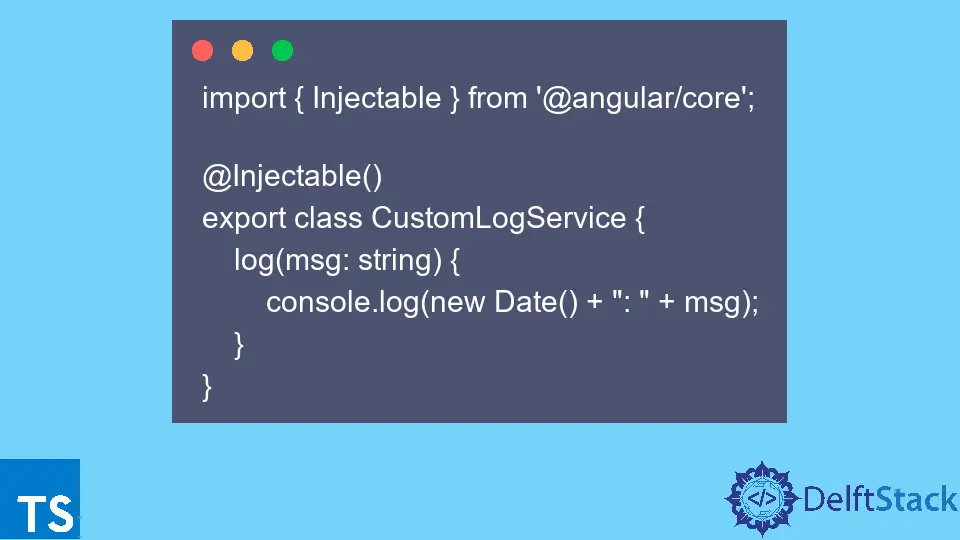
ロギングはアプリケーションにとって非常に重要であり、デバッグ目的に役立ち、本番環境でのアプリケーションの問題を知ることができます。 Angular はすべてサービスに関するものであり、console.log
関数のラッパーとして機能するロギング サービスを作成できるように、他のコンポーネントに挿入できます。
ロギング サービスは、分析やデータベースのためにリモート サーバーにログを送信するなど、ログを使用したさらなるアクションに拡張できます。 このチュートリアルでは、Angular のサービスを使用して TypeScript の console.log
関数のラッパーを作成する方法を示します。
TypeScript で Angular 2 の console.log
ラッパーを書く
Angular はサービスで構成されているため、新しいログ サービスを作成できます。 このサービスは、後で分析やオブザーバビリティなどのさらなるユースケースに拡張できます。
services/log.service.ts
import { Injectable } from '@angular/core';
@Injectable()
export class CustomLogService {
log(msg: string) {
console.log(new Date() + ": " + msg);
}
}
したがって、CustomLogService
は console.log
のラッパーとして機能し、他の Angular コンポーネントで使用できます。 CustomLogService
クラスを注入するために、HTML ファイルが使用できるコンポーネントを定義できます。
tests/log.component.html
<button (click)="wrapLog()">Log</button>
tests/log.component.ts
import { Component } from "@angular/core";
import { CustomLogService } from "../services/log.service";
@Component({
selector: "log-comp",
templateUrl: "./log.component.html"
})
export class LogComponent {
constructor(private logger: CustomLogService) {}
wrapLog(): void {
this.logger.log("A custom log method");
}
}
さらに app.module.ts
を定義する必要があります。
app.module.ts
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { AppComponent } from "./app.component";
import { CustomLogService } from "./services/log.service";
import { LogComponent } from "./tests/log.component";
@NgModule({
imports: [BrowserModule],
declarations: [AppComponent, LogComponent],
bootstrap: [AppComponent, LogComponent],
providers: [CustomLogService]
})
export class AppModule {}
tests/log.component.ts
で定義されたセレクター log-comp
を <log-comp></log-comp>
として使用して、クリックするとコンソールにログを記録する HTML ボタンを表示できるようになりました。 - tests/log.component.ts
で定義されている カスタム ログ メソッド
。
出力:
完全なソース コードの デモ を次に示します。