How to Represent Integer in TypeScript
-
Use the
number
Type to Represent Numbers in TypeScript -
Use the
bigint
to Represent Very Large Numbers in TypeScript -
Use the
parseInt
Built-In Function to Convert From String to Integer in TypeScript -
Use the
+
Operator to Convert a String to Number in TypeScript
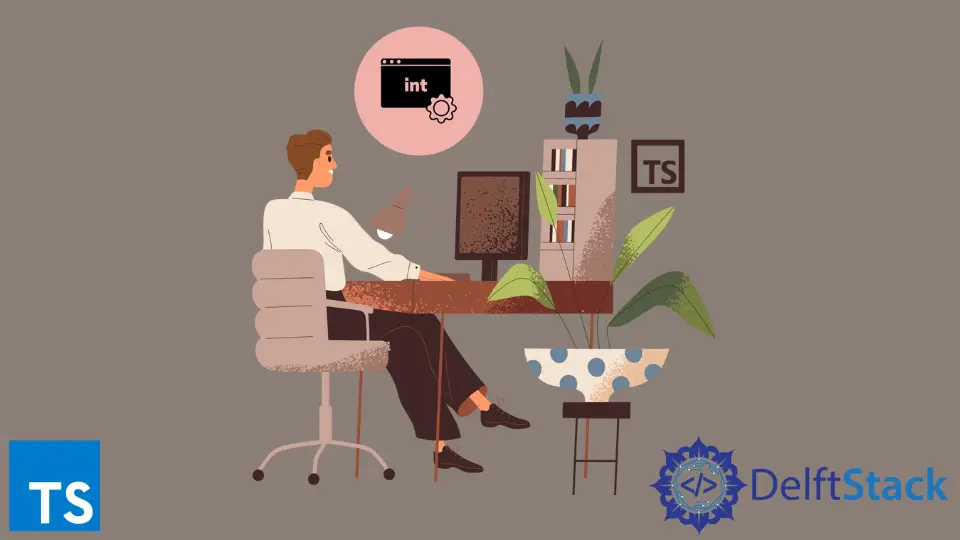
In TypeScript, there is no concept of integer data types like in other programming languages. Only a number
type is used to represent floating-point numbers in general.
The bigint
is the latest version of TypeScript, which represents big integers.
Use the number
Type to Represent Numbers in TypeScript
The number
type in TypeScript can hold both integer and floating-point data types.
It can also store numbers having different bases like binary, octal and hexadecimal.
// the count of something is in whole numbers
var countDogs : number = 10;
// the price of a bag can be a floating point number
var priceBag : number = 200.99;
var binaryNumber : number = 0b101;
var octalNumber : number = 0o30;
var hexadecimalNumber : number = 0xFF;
console.log(countDogs);
console.log(priceBag);
console.log(binaryNumber);
console.log(octalNumber);
console.log(hexadecimalNumber);
Output:
10
200.99
5
24
255
The numbers in different bases are printed out in base 10
. The binary or base 2
can be represented by prefixing with 0b
or 0B
, similarly for base 16
, it has to be prefixed with 0x
or 0X
.
Use the bigint
to Represent Very Large Numbers in TypeScript
The bigint
type is used for representing very large numbers (numbers more than 2<sup>53</sup> - 1)
and has the n
character at the end of the integer literal.
var bigNumber: bigint = 82937289372323n;
console.log(bigNumber);
Output:
82937289372323
Use the parseInt
Built-In Function to Convert From String to Integer in TypeScript
The parseInt
function is used for conversion from string to integer, and the parseFloat
function is used to convert from string to floating-point data type.
var intString : string = "34";
var floatString : string = "34.56";
console.log(parseInt(intString));
console.log(parseInt(floatString));
console.log(parseFloat(intString));
console.log(parseFloat(floatString));
var notANumber : string = "string";
console.log(parseInt(notANumber));
Output:
34
34
34
34.56
NaN
Thus the parseInt
and parseFloat
methods will return NaN
.
Use the +
Operator to Convert a String to Number in TypeScript
The +
operator can convert a string literal to a number
type. Further operations are done once it is converted to a number
type.
function checkiFInt( val : number | string ) {
if (( val as any) instanceof String){
val = +val as number;
}
console.log(Math.ceil(val as number) == Math.floor(val as number));
}
checkiFInt('34.5');
checkiFInt('34');
checkiFInt('34.5232');
checkiFInt('34.9');
checkiFInt('0');
Output:
false
true
false
false
true