TypeScript 中的整數型別
Shuvayan Ghosh Dastidar
2023年1月30日
TypeScript
TypeScript Number
-
在 TypeScript 中使用
number
型別表示數字 -
在 TypeScript 中使用
bigint
表示非常大的數字 -
在 TypeScript 中使用
parseInt
內建函式將字串轉換為整數 -
在 TypeScript 中使用
+
運算子將字串轉換為數字
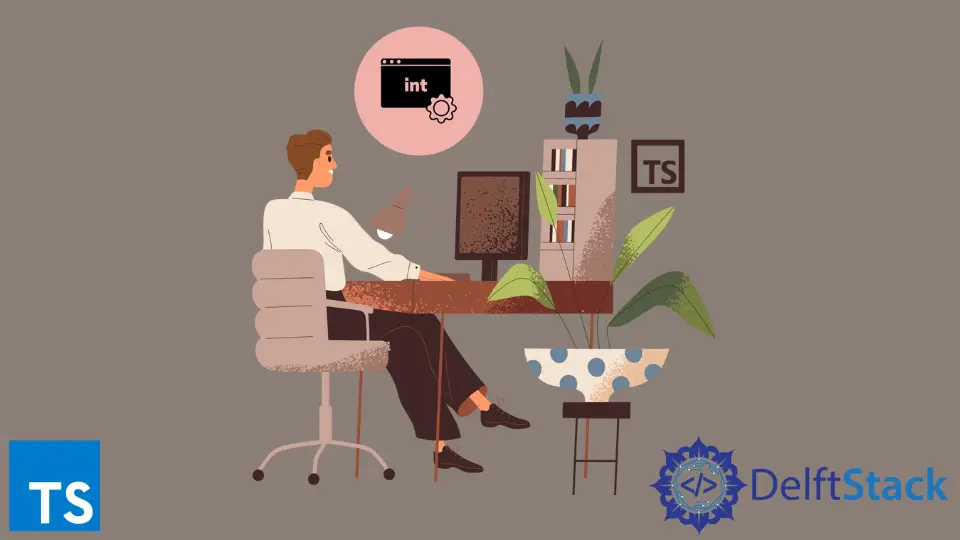
在 TypeScript 中,沒有像其他程式語言那樣的整數資料型別的概念。通常,只有 number
型別用於表示浮點數。
bigint
是 TypeScript 的最新版本,它表示大整數。
在 TypeScript 中使用 number
型別表示數字
TypeScript 中的 number
型別可以同時儲存整數和浮點資料型別。
它還可以儲存具有不同基數的數字,例如二進位制、八進位制和十六進位制。
// the count of something is in whole numbers
var countDogs : number = 10;
// the price of a bag can be a floating point number
var priceBag : number = 200.99;
var binaryNumber : number = 0b101;
var octalNumber : number = 0o30;
var hexadecimalNumber : number = 0xFF;
console.log(countDogs);
console.log(priceBag);
console.log(binaryNumber);
console.log(octalNumber);
console.log(hexadecimalNumber);
輸出:
10
200.99
5
24
255
不同基數的數字以 base 10
列印。二進位制或 base 2
可以通過字首 0b
或 0B
來表示,類似地對於 base 16
,它必須以 0x
或 0X
作為字首。
在 TypeScript 中使用 bigint
表示非常大的數字
bigint
型別用於表示非常大的數字 (numbers more than 2<sup>53</sup> - 1)
並且在整數文字的末尾有 n
字元。
var bigNumber: bigint = 82937289372323n;
console.log(bigNumber);
輸出:
82937289372323
在 TypeScript 中使用 parseInt
內建函式將字串轉換為整數
parseInt
函式用於從字串轉換為整數,parseFloat
函式用於從字串轉換為浮點資料型別。
var intString : string = "34";
var floatString : string = "34.56";
console.log(parseInt(intString));
console.log(parseInt(floatString));
console.log(parseFloat(intString));
console.log(parseFloat(floatString));
var notANumber : string = "string";
console.log(parseInt(notANumber));
輸出:
34
34
34
34.56
NaN
因此 parseInt
和 parseFloat
方法將返回 NaN
。
在 TypeScript 中使用 +
運算子將字串轉換為數字
+
運算子可以將字串文字轉換為 number
型別。將其轉換為 number
型別後,將執行進一步的操作。
function checkiFInt( val : number | string ) {
if (( val as any) instanceof String){
val = +val as number;
}
console.log(Math.ceil(val as number) == Math.floor(val as number));
}
checkiFInt('34.5');
checkiFInt('34');
checkiFInt('34.5232');
checkiFInt('34.9');
checkiFInt('0');
輸出:
false
true
false
false
true
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe