How to Ignore Next Line in TypeScript
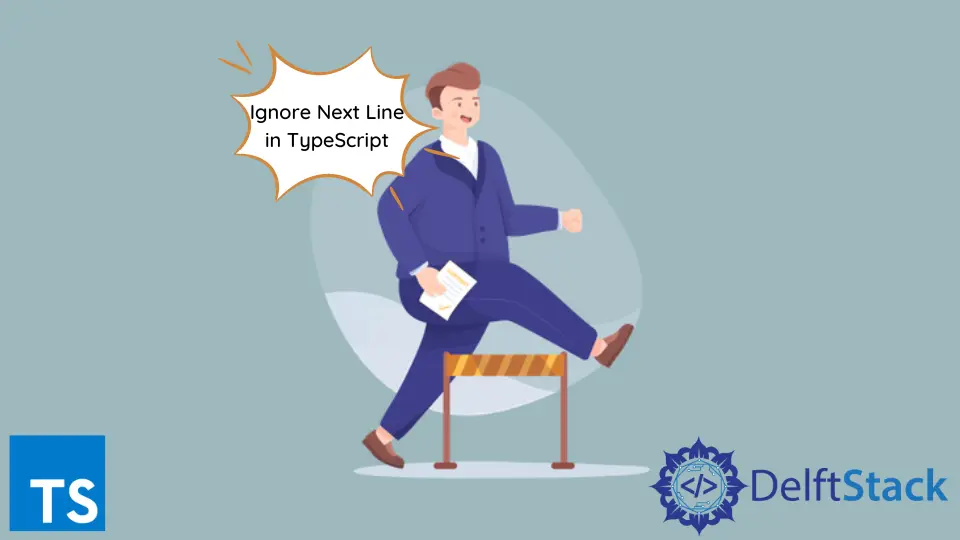
We will introduce how to ignore the next line in TypeScript with examples.
Ignore Next Line in TypeScript
This tutorial will teach us about the new important thing in the TypeScript programming language. We will learn how to skip or ignore the following line in the TypeScript programming language.
Now we will give you an overview of the TypeScript language.
TypeScript is a programming language, a subset of JavaScript, developed by Microsoft company. In this language, Microsoft introduces an additional feature, strict type binding.
It is widely used in significant applications, transpiles to JavaScript, and developing interactive web pages.
If we are studying or working in a TypeScript programming language, many occasions occur when we need to skip or disable the following line for the performance of any task. If we need to disable a line at this point, we use the function of line skipper.
For this task, we use the // @ts-ignore
function. This function automatically disables the next line in the TypeScript code.
This disables the checking of the next line. The comment // @ts-ignore
disables checking all the types in the following line.
If we want to ignore the type checking of a single file, then the // @ts-nocheck
comment is best for this purpose.
# Typescript
// @ts-ignore
function logMessage(message) {
console.log(message);
return message;
}
Output before @ts-ignore
comment:
After using the @ts-ignore
comment:
As we can see from the above examples, the error displays are removed because it is skipping the type checking for the message variable.
Now, let’s go through an example of @ts-nocheck
. As shown below, we will create two functions without defining the variable type.
# Typescript
// @ts-ignore
function logMessage(message) {
console.log(message);
return message;
}
function logMessage2(message2) {
console.log(message2);
return message2;
}
Output:
Now, if we use the // @ts-nocheck
, it will ignore type checking for all the lines after the comment. Let’s use it and check how it works, as shown below.
# typescript
// @ts-nocheck
function logMessage(message) {
console.log(message);
return message;
}
function logMessage2(message2) {
console.log(message2);
return message2;
}
Output:
As we can see from the above example, it ignored all the errors after the comment using the @ts-nocheck
, unlike @ts-ignore
.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn