How to Extend Window in TypeScript
-
TypeScript
Window
Object -
Declare New Property in the
Window
Object in TypeScript - Interface Declaration Merging in TypeScript
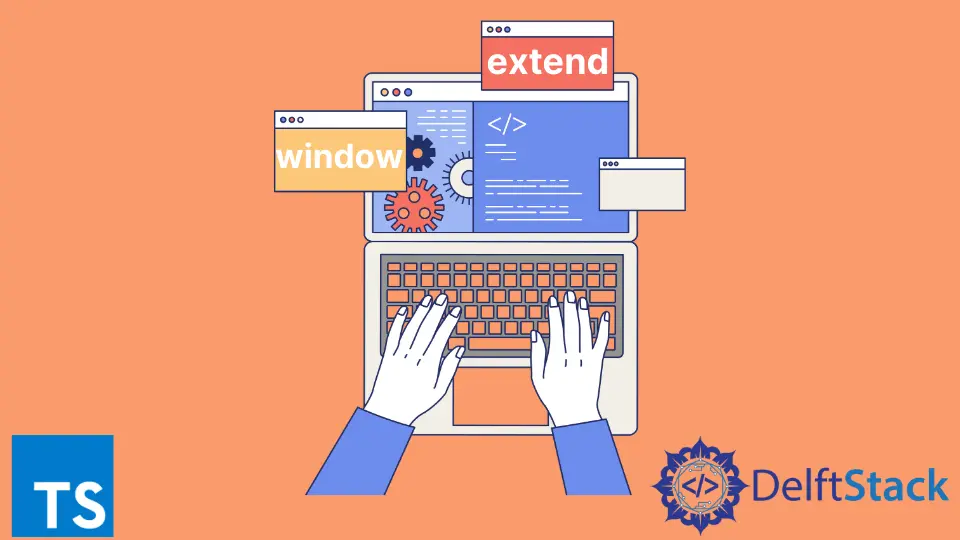
This article shows how to extend a TypeScript window
.
TypeScript Window
Object
A window
is a built-in object defined in the browser. It is the window
object that contains a DOM document.
You might need to access the properties and functions associated with the browser window
object. The built-in window
object carries a lot of useful properties and functions out of the box.
Some of the frequently used window
object properties and functions are listed in the following.
- Properties:
EventTarget
,document
,console
,event
,localStorage
,onLoad
, etc. - Methods:
addEventListener
,fetch
,alert
,atob
,btoa
,open
,prompt
,print
, etc.
Usually, TypeScript defines its types in different modules. Hence, the window
type is defined in the lib.dom
module.
In some scenarios, the built-in methods and properties are not enough. Whenever your TypeScript project uses Google Analytics or Tag Manager libraries, these scripts might not be loaded by your code.
Hence, we need a way to communicate with those third-party tools via the window
object properties and methods.
Declare New Property in the Window
Object in TypeScript
In JavaScript, it is quite straightforward to declare a new property or method in the built-in window
object. We can use the following ways to define a new property in the window
object with JavaScript.
window.myProp = 'newly declared property';
window['myProp'] = 'newly declared property';
Let’s inspect the window
object in the browser console.
console.log(window);
Output:
In TypeScript, the above approaches wouldn’t work. If we try to declare a new property in the window
object like in JavaScript, TypeScript will throw an error, as shown in the following.
window.anotherNewProp = 'this is a property on window object in typescript';
Output:
Usually, a TypeScript object type is based on a class or an interface. Let’s say we have a class called Animal
, as shown in the following.
class Animal {
name: string;
color: string;
}
Let’s create an Animal
object and assign some values to the name
and color
properties.
class Animal {
name: string;
color: string;
}
let newAnimal = new Animal();
newAnimal.name = 'lion';
newAnimal.color = 'grey';
The above code will compile without any issue.
Next, we will try to declare a new property numberOfLegs
on the same Animal
object and assign a value.
class Animal {
name: string;
color: string;
}
let newAnimal = new Animal();
newAnimal.name = 'lion';
newAnimal.color = 'grey';
newAnimal.numberOfLegs = 4;
It raises an error. We can’t declare new object properties like that in TypeScript.
To resolve that, we first need to add the numberOfLegs
property to the Animal
class.
This is the same issue with the built-in window
object also. The main concern is TypeScript window
object is not created by ourselves.
It comes with a browser. Hence we need a proper way to add a property to this built-in object.
Interface Declaration Merging in TypeScript
TypeScript supports the declaration merging technique out of the box. The simplest type of declaration merging is interface merging.
Whenever the multiple interfaces are declared with the same name, TypeScript will merge all the interfaces declarations into one.
The built-in window
object is based on the TypeScript window
interface. Hence, we can use the TypeScript interface merging technique to add a new property to the built-in interface.
Let’s create a new interface named window
.
interface Window {
myProp: string;
}
Next, we will try to access the myProp
property in the window
object.
interface Window {
myProp: string;
}
window.myProp = 'typescript window object property';
Output:
There are no issues raised by the TypeScript compiler this time. Defining new properties or methods in the window
object is recommended if you consider the types.
If there are no concerns on types, the following syntax can be used to declare new properties on the window
object.
(<any>.window).myProp = 'typescript property on window object';
You can declare functions as well.
(<any>.window).NewFunction = () => {
console.log('new function on window object');
}
Both techniques can be used with TypeScript to add new properties or methods to the window
object. The choice is yours based on the context.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.