How to Watch and Compile All TypeScript Sources
- JavaScript Execution
- TypeScript to JavaScript Transpilation Process
- Compile TypeScript Sources
- Configure TypeScript Compiler to Watch
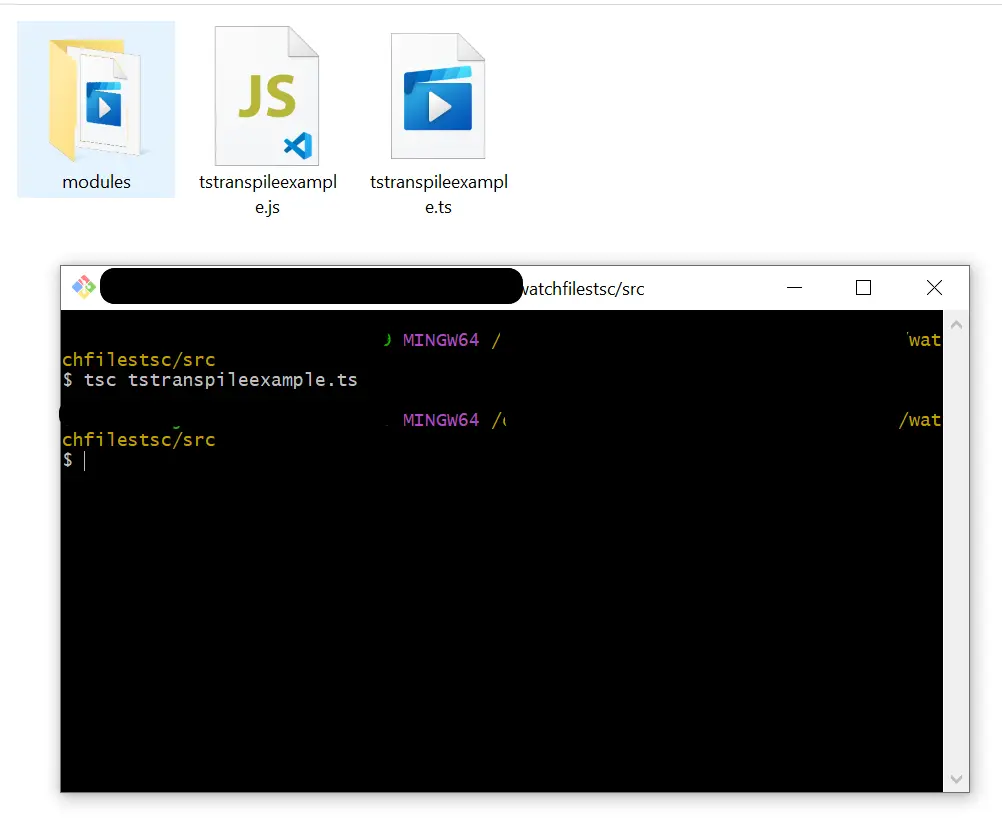
This article explains how to watch and compile all TypeScript sources.
JavaScript Execution
TypeScript language is the typed version of JavaScript. Every JavaScript code is a syntactically valid TypeScript because the TypeScript syntax is a superset of ES2015 syntax.
In some cases, the JavaScript code might not be processed as a valid code by the TypeScript compiler. It is due to the compile-time type checking feature in TypeScript language.
It avoids most runtime errors that occur due to the wrong usage of types.
As we all know, browsers are capable of executing JavaScript code. All the browsers contain a JavaScript engine specific to the browser, which interprets JavaScript programs and runs on the fly.
Usually, the Chrome browser comes with Google’s V8 JavaScript engine, and Firefox uses the SpideMonkey engine to handle JavaScript. Nowadays, JavaScript can be executed on the server-side as well.
Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine to run the JavaScript code outside the browser. Hence, the browsers and server-side run time environments like Node.js are only capable of interpreting JavaScript code.
TypeScript to JavaScript Transpilation Process
With the evolution of the TypeScript language, there was a need for a transpilation process because the JavaScript engines are only known to interact with JavaScript language. JavaScript engines do not have a clue about TypeScript.
The transpilation is where the TypeScript code converts back to its JavaScript version. Then the converted JavaScript pieces can be thrown to the JavaScript engine for execution.
Whenever you encounter a TypeScript source code, there is an additional step to compile all the TypeScript sources to JavaScript. We will discuss the TypeScript source compilation more in the following section.
Compile TypeScript Sources
A TypeScript compiler must be installed in your system to transpile TypeScript source code to regular JavaScript. The most convenient way to install TypeScript is via the node package manager
(NPM
).
It can be installed only for a specific project, as shown in the following. Node.js should be installed ahead that installs npm
as well.
npm install typescript --save-dev
You can install TypeScript globally as well.
npm install -g typescript
Finally, it is recommended to check the TypeScript version as shown in the following. If the TypeScript has been installed properly, the command will display the version correctly.
tsc -v
Output:
Version 4.6.4
This version might be different for you.
You can use the tsc
command to transpile TypeScript source code into a regular JavaScript code.
Syntax:
tsc <source.ts>
Let’s create a TypeScript source file called tstranspileexample.ts
with some TypeScript code, as shown in the following.
class Vehicle {
vehicleNo: string;
vehicleBrand: string
}
Next, we can open up a command window within the folder where the created TypeScript file resides and run the following command.
tsc tstranspileexample.ts
Output:
As expected, the TypeScript compiler compiled the tstranspileexample.ts
source to the regular JavaScript file called tstranspileexample.js
. Now you can run the generated JavaScript code in the browser or Node.js environment.
In enterprise-level projects, hundreds of TypeScript files exist in different folders. Hence, it is not practical to transpile files one by one.
The following section has concerned with configuring the TypeScript compiler to compile multiple files on the fly.
Configure TypeScript Compiler to Watch
TypeScript introduced a configuration file called tsconfig.json
to override default TypeScript compiler options.
It is a JSON formatted file where we can add different configuration properties known to the TypeScript compiler. It can modify the default behavior of the tsc
command.
In most real-world TypeScript projects, we must instantly compile TypeScript sources from different locations and transpile them into regular JavaScript.
Whenever a modification is done in a TypeScript file, the TypeScript compiler should compile the modified file on the fly and recreate the relevant JavaScript code. This is called as watch and compile process.
Let’s create the tsconfig.json
file with the below content.
{
"compilerOptions": {
"target": "es2016",
"module": "commonjs",
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true,
"skipLibCheck": true,
"outDir": "ts-built",
"rootDir": "src"
}
}
The compilerOptions
section defined the configuration properties related to TypeScript code compilation. The target ECMAScript version is es2016
, the generated JavaScript files will be pushed to the ts-built
folder, and the rootDir
property defines the directory where the TypeScript compiler should look for the TypeScript source files.
With this configuration setup, you can run the following command to enable the watch and compile feature in the TypeScript compiler.
tsc -w
The command window will turn into the watch mode, as shown in the following.
If you modify one of your source TypeScript files and save it, the TypeScript compiler will recompile all the modified files and generate the regular JavaScript files. This powerful feature comes with the TypeScript compiler to use in larger projects containing hundreds of source files.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.