How to Convert String to Enum in TypeScript
- The Enum DataType in Typescript
- Numeric Enums in TypeScript
- String-Based Enums in TypeScript
- Multiple Enums in TypeScript
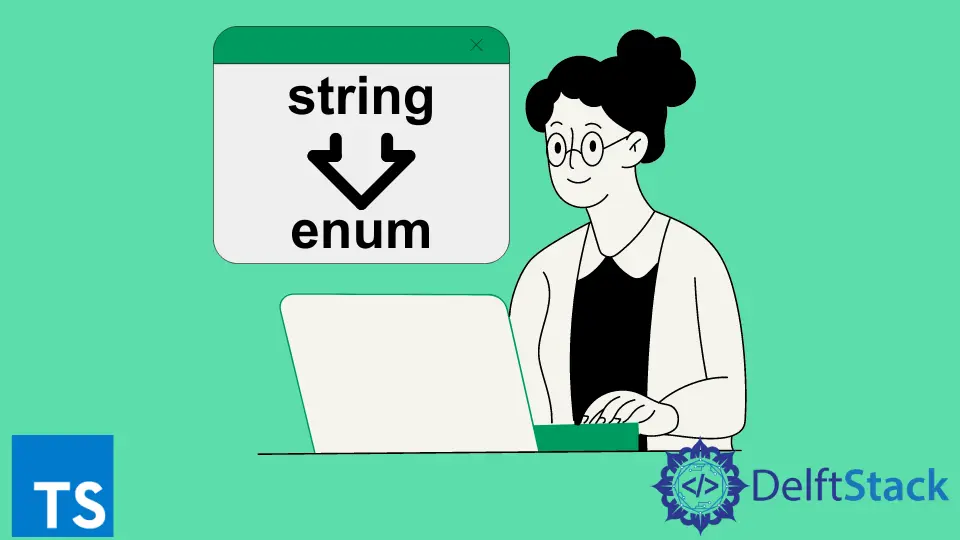
An enum type is a datatype to pre-define constants for a variable. The variable must have a value defined for it in the enum.
This tutorial will discuss the concept of enum in typescript.
The Enum DataType in Typescript
Typescript allows the user to use the enum type as a data type for a variable. A common example of an enum is the name of the months, where the user has to select a month defined in a year.
Typescript provides both the string-based and numeric enums.
Numeric Enums in TypeScript
An enum is defined using the keyword enum
as the name suggests that the numeric enum is for numeric values.
Example Code:
enum basicEnum {
a = 3,
b = 6,
c = 7,
}
console.log(basicEnum);
Output:
{
"3": "a",
"6": "b",
"7": "c",
"a": 3,
"b": 6,
"c": 7
}
This is a simple enum type instance. Let us take another example.
Example:
enum basicEnum {
a = 3,
b,
c,
}
console.log(basicEnum);
Output:
{
"3": "a",
"4": "b",
"5": "c",
"a": 3,
"b": 4,
"c": 5
}
In the above example, the first variable a
is declared, and the rest are incremented by 1 from that initialized value.
In case the user left each variable undefined. Then the values will start from 0, and the rest will be incremented by 1.
The user can also get an individual variable on an enum.
Example Code:
enum basicEnum {
a = 3,
b = 6,
c = 7,
}
console.log('The first enum variable a has a value = ' + basicEnum.a);
console.log('The second enum variable b has a value = ' + basicEnum.b);
console.log('The last enum variable c has a value = ' + basicEnum.c);
Output:
The first enum variable a has a value = 3
The second enum variable b has a value = 6
The last enum variable c has a value = 7
The above example shows the access of each variable of an enum.
String-Based Enums in TypeScript
The string-based enums operate the same as the numeric-based enums, but each variable has to be initialized in the string-based enum. If a variable needs to empty, it must be declared as an empty string.
Example Code:
enum stringEnum {
a = "Hello",
b = "Bye",
c = "",
}
console.log(stringEnum);
Output:
{
"a": "Hello",
"b": "Bye",
"c": ""
}
The above example shows the use of string-based enum. Notice how an empty string is defined for member c
.
If a user leaves a variable undefined, an error will be raised.
Example Code:
enum stringEnum {
a = "Hello",
b = "Bye",
c,
}
console.log(stringEnum)
Output:
{
"a": "Hello",
"b": "Bye",
"c": undefined,
"undefined": "c"
}
Notice in the output c
is undefined. For the variable c
enum member to not return undefined, it must have an initializer.
Multiple Enums in TypeScript
A user can define multiple enums in typescript. This is a good practice to keep the numeric and string-based enums separate.
Example Code:
enum firstEnum {
a = "Hello",
b = "Bye",
c = "",
}
enum secondEnum {
x = 2,
y = 4,
z = 8,
}
console.log(firstEnum);
console.log(secondEnum);
Output:
{
"a": "Hello",
"b": "Bye",
"c": ""
}
{
"2": "x",
"4": "y",
"8": "z",
"x": 2,
"y": 4,
"z": 8
}
Let us take another example to discuss the bad use of the enum data type.
Example Code:
enum badEnum {
a = "Hello",
b = 4,
}
console.log(badEnum);
Output:
{
"4": "b",
"a": "Hello",
"b": 4
}
Here, it shows that the respective members must have the same data type whenever an enum is defined. Although typescript does not raise an error, it is a bad practice to handle enum in this way.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn