How to Use RxJS With TypeScript
- TypeScript Asynchronous Execution
- Reactive Programming With RxJS
- Setup RxJS With TypeScript
- Consume RxJS Inside TypeScript
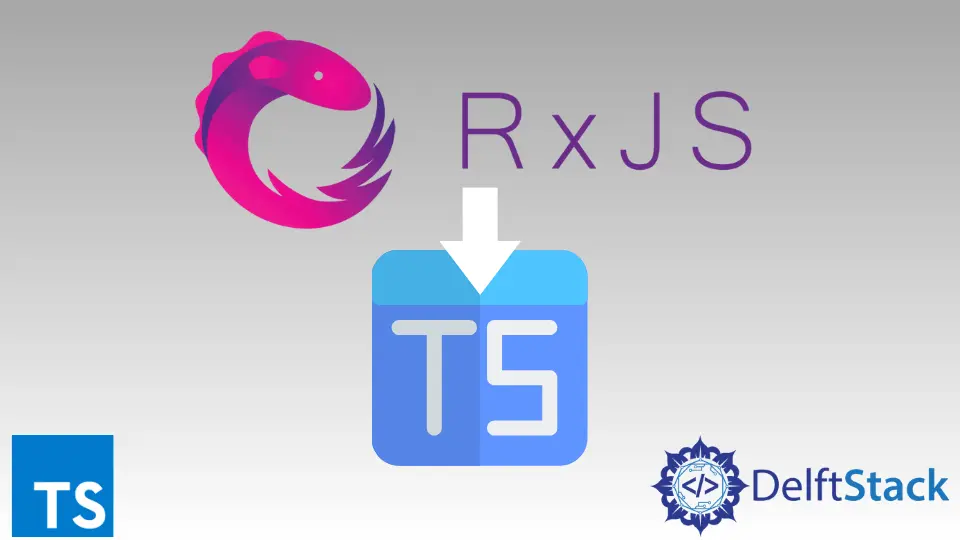
TypeScript is a superset of JavaScript language which supports static typing and type checking while keeping all the other features that JavaScript offers. One of the advanced and widely used features is asynchronous program execution.
Modern-day applications are highly interactive and consumer-oriented. Hence, most of the information is available asynchronously.
Several techniques are available in TypeScript to handle asynchronous logic such as callbacks, promises, and events.
TypeScript Asynchronous Execution
The callback functions were the oldest technique used to handle asynchronous code. There were some drawbacks with callbacks, such as no proper way to catch errors, introducing callback hell, and maintenance issues.
Hence, the concept of the promise has been introduced. A promise mimics a real-world promise where an asynchronous operation generates an eventual result that might be a success or failure.
In TypeScript, a promise object accepts a function with two parameters named resolve and reject to keep or fail the promise. This is a big step in asynchronous programming, but the callback hell issues are still there.
The publisher-subscriber technique is another popular way of handling asynchronous events. Whenever an object’s state changes, it will raise notifications to its subscribers or observers.
It is a more advanced way of handling asynchronous operations than callbacks, but it still has some drawbacks, such as can’t subscribe to a series of events to handle them sequentially, events might lose forever, etc.
Reactive Programming With RxJS
As mentioned earlier, event processing is like finding the way out of a maze. Hence, the event-driven application implementation was cumbersome.
Hence, the reactive programming methodology was introduced. It simplified the execution of asynchronous code and the implementation of event-driven programs in TypeScript.
It relies on the concept of Observable
. Furthermore, the observer and iterator patterns have been used.
Setup RxJS With TypeScript
First, we need to create a Node.js project. You can install Node.js from the official site.
When you install Node.js, it automatically sets up the NPM
for you. Let’s generate the package.json
file as shown in the following.
npm init
It will prompt you to enter additional details for your Node project and stick to the default ones for now.
The RxJS
library is available as an NPM
package. Hence we can install it with npm
as shown in the following.
npm install rxjs
OR
npm i rxjs
It would create a separate node_modules
folder to keep all the third-party dependencies, including the RxJS
module.
Next, we should generate the TypeScript configuration file, which holds some basic configurations for the TypeScript compiler.
tsc --init
This would generate a new JSON file called tsconfig.json
. Let’s add the following entries to it.
{
"compilerOptions": {
"target": "es2016",
"module": "commonjs",
"esModuleInterop": true,
"forceConsistentCasingInFileNames": true,
"skipLibCheck": true
},
"files": [
"rxjstest.ts",
]
}
Here the files array contains all the files that we need to compile. In this example, we will write all the TypeScript logic in the rxjstest.ts
file.
Consume RxJS Inside TypeScript
Since we have already installed the RxJS
library, we can import the necessary types and methods from the rxjs
module. In this example, we will import the Observable
type and of
method from the rxjs
library.
import { of, Observable } from "rxjs";
Let’s create a new Observable
type object that publishes a city’s temperature values.
const weatherPublisher : Observable<number> = of(25, 12,45,18);
The weatherPublisher
is an Observable
type object that generates four number values using the RxJS
of
method.
Next, we can subscribe to the weatherPublisher
observable. It emits four temperatures, as shown in the following.
weatherPublisher.subscribe((value: number) => {
console.log(`Temperature: ${value}`)
})
Since we are done with the code, let’s compile the rxjstest.ts
file using the following command.
tsc -p ./tsconfig.json
It would generate the corresponding JavaScript file. Then, we can run the JavaScript file as shown in the following.
node rxjstest.js
Output:
Temperature: 25
Temperature: 12
Temperature: 45
Temperature: 18
The RxJS
library works fine with the TypeScript setup.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.