How to Return Type for setTimeout in TypeScript
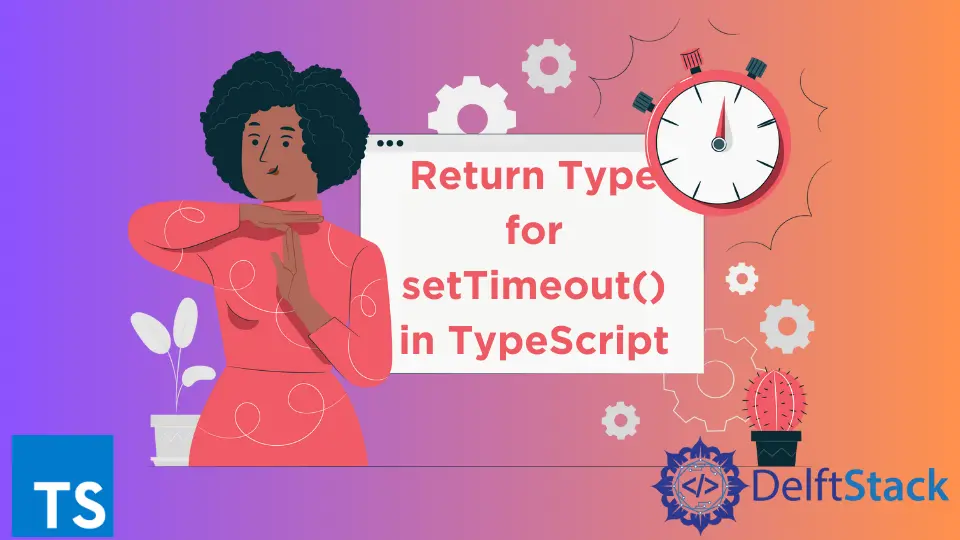
setTimeout()
is a method used to call a function after a specified amount of time in milliseconds. This method acts as a timer and executes the code after the timer expires.
setTimeout()
cannot be executed multiple times; it can only be executed once. If recurrent executions are needed, the setInterval()
method should be used instead of setTimeout()
.
Furthermore, setTimeout()
is also referred to as an asynchronous function. It does not perform tasks one at a time.
Instead, a later function in the pecking order is executed while another is executed. Therefore, asynchronous functions can be performed but cannot be used to create a pause between functions in a function stack.
This article will explain the return type for the setTimeout()
function and how to specify it in TypeScript.
Return Type for the setTimeout()
in TypeScript
There is no imperative need to specify a return type by letting type inference identify and return the correct return type.
We could use two main methods if it is logically essential to establish a return type, like an instance where there is no consistency between the browser and node declarations.
The ReturnType
method could be used so that the return type of setTimeout()
and variable type would be the same. The other method is window.setTimeout()
, which will return the actual return type.
Use ReturnType
to Specify Return Type for the setTimeout()
in TypeScript
One method that we can use is ReturnType
. It does not specify a particular type but instead lets the type get inferred automatically.
const timer: ReturnType<typeof setTimeout> = setTimeout(() => {
console.log("Hi!")
}, 3000);
Output:
Hi!
The output of the above code would appear three seconds after the code execution.
Consider another example where we specify the return type as number
.
const timer: number = setTimeout(() => {
console.log("Hi!")
}, 2000);
Output:
Hi!
The above method would come across as an error in Node.js.
In Node.js timer, the object is returned instead of the numeric return value. Hence, we can use window.setTimeout()
to avoid this situation.
Use window.setTimeout()
to Specify Return Type for the setTimeout()
in TypeScript
window.setTimeout()
and setTimeout()
both do a similar function, but they perform it in different places. window.setTimeout()
can only perform its actions on the browser whereas setTimeout()
can perform its actions anywhere.
When a browser is not used, we can call the methods of a global object without referring to the object. However, when TypeScript or JavaScript is run on a browser, Document Object Model (DOM) is responsible for providing the global object.
Hence, window
is specified in window.setTimeout()
as the Document Object Model specifies that the global object has a window
property.
Syntax:
window.setTimeout(function() {}, 0);
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.