Nullable Type in TypeScript
- Nullable Type in TypeScript
- Making Property Optional in TypeScript
- Union Type for Nullable in TypeScript
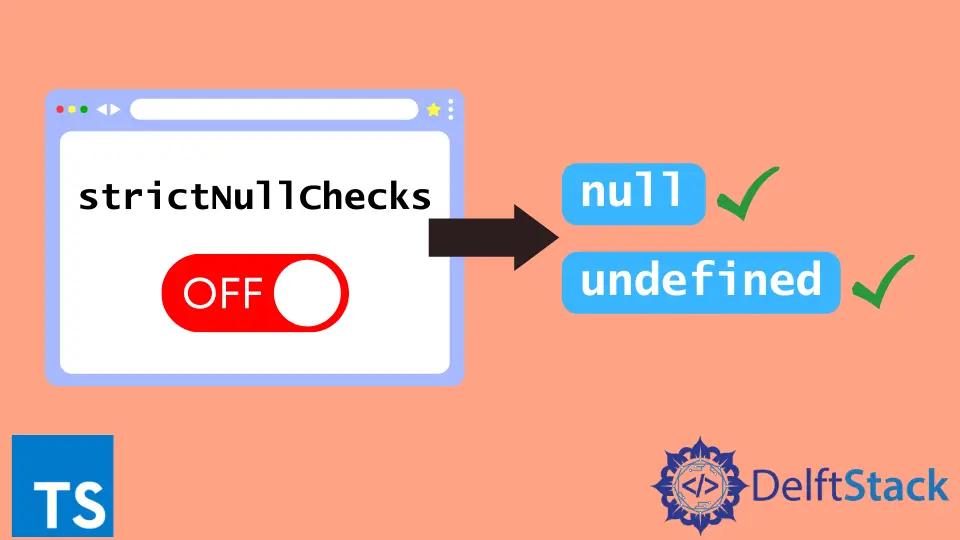
In recent TypeScript versions, null
and undefined
were not readable. However, the newer version supports this.
This tutorial will discuss the concept of nullable type in TypeScript.
Nullable Type in TypeScript
The user must switch off the type checking mode to use the null
and undefined
types in TypeScript. Use the strictNullChecks
flag to check the type of property.
If the strictNullChecks
flag is on will not allow the user to define types null
and undefined
. By default, the flag is turned on, and the user has to switch it off manually.
Code:
interface Info {
name: string,
age: number,
city: string,
}
const info: Info = {
name: 'John',
age: null,
city: 'Washington'
}
There will be no output since the type checker flag is turned on. An error will be raised that 'Type null is not assignable to type number'
.
The code will execute if the user manually switches off the type checker flag.
Code:
interface Info {
name: string,
age: number,
city: string,
}
const info: Info = {
name: 'John',
age: null,
city: 'Washington'
}
console.log(info)
Output:
{
"name": "John",
"age": null,
"city": "Washington"
}
Now the above only executes when the type checker flag is turned off.
Making Property Optional in TypeScript
There is another method to eliminate null
and undefined
. It is the most preferred way to handle properties.
TypeScript allows making properties optional so that these properties can only be used when needed. Instead of declaring a property null
each time it is of no use, an optional rendering is preferred.
Code:
interface Info {
name: string,
age?: number,
city: string,
}
const info1: Info = {name: 'John', city: 'Washington'};
const info2: Info = {name: 'Jack', age: 13, city: 'London'};
console.log(info1);
console.log(info2);
Output:
{
"name": "John",
"city": "Washington"
}
{
"name": "Jack",
"age": 13,
"city": "London"
}
Notice in the above example that the property age
is optional. There was no need for the property age in the first object, info1
, so it was never called.
If the type checker is off and the user sets age in info1
equal to null
, it will raise an error. Now, suppose there were 100 objects, and only 10 of them need the age
property, so instead of declaring age
into null
, make it optional.
Union Type for Nullable in TypeScript
The union type is used when the user does not want to turn off the type checker. A union type is also a preferred method, but it has the complexity of assigning null
whenever the property is used.
Code:
interface Info {
name: string,
age: number | null,
city: string,
}
const info1: Info = {name: 'John', age: null, city: 'Washington'};
const info2: Info = {name: 'Jack', age: 13, city: 'London'};
console.log(info1);
console.log(info2);
Output:
{
"name": "John",
"age": null,
"city": "Washington"
}
{
"name": "Jack",
"age": 13,
"city": "London"
}
Notice the declaration of age
in the interface. A union type is used to assign multiple types to a property, and it works fine even in the strict mode.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn