How to Use Lodash in TypeScript
- Lodash in TypeScript
- Get the First and Last Array Elements Using Lodash in TypeScript
- Generate a Random Number Using Lodash in TypeScript
- Unwrap an Array Using Lodash in TypeScript
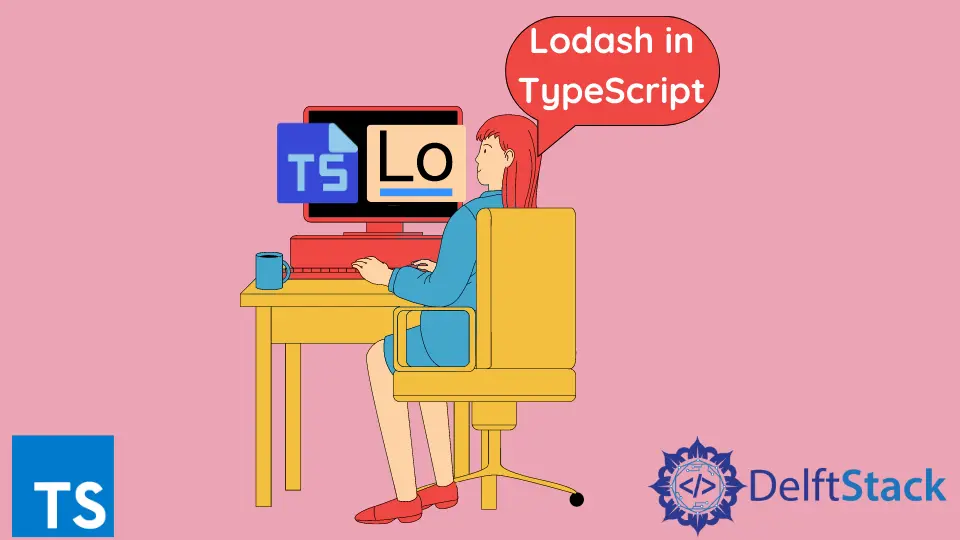
We will introduce Lodash in TypeScript and learn how to use it with examples.
Lodash in TypeScript
While working on a big project or application, we always have wondered about a library that could provide us with some basic functions or functionalities that could be used in every project to achieve some basic goals.
Lodash is a JavaScript library that provides utility functions for our everyday programming tasks. These include getting the first or last array element, a specific array element based on the position, chunking or slicing an array, etc.
Microsoft designed TypeScript as a superset of JavaScript with optional static typing. We can also include characteristics, such as classes and pointer functions that support more structure with its module system and optional type annotations.
TypeScript is very difficult when it comes to utilizing the code outside the TypeScript. The difference between JavaScript and TypeScript is that JavaScript is without type annotations.
Every object is treated as having any type in the library. All methods call the return of any type and immediately pass the data to the other libraries by untyped it.
We will look at Lodash’s _.flatten()
function, used to look at the new feature in TypeScript. Lodash is a great library that will utilize the functions for all sorts of things that will be useful in JavaScript, including array manipulation.
This article will go through multiple Lodash functions that can be used. Let’s start by installing this library using the following npm
command.
# typescript
npm i lodash
Once we have installed Lodash, we can check the version of the Lodash by using the following command, as shown below.
# lodash
const _ = require("lodash");
const ver = _.VERSION;
console.log(ver);
Output:
Sometimes after installing lodash
, we can get the error on importing from lodash
. The best method is to install the lodash
types using the following command.
# typescript
npm install --save-dev @types/lodash
Once we have installed the lodash
types, we can easily import the lodash
module using the following line of code.
# typescript
import * as _ from `lodash`;
Now, we will go through some basic functions that Lodash provides us for our common programming tasks one by one.
Get the First and Last Array Elements Using Lodash in TypeScript
Lodash deals a lot in array manipulation and some most commonly used scenarios. We can use the _.first
and _.last
functions to get the array’s first or last element.
We can also use the _.nth
to get the nth element of the array. We can get from the end of the array by using the negative index, as shown below.
# typescript
const _ = require("lodash");
let arraySample = ['This', 'is', 'Lodash', 'in', 'Typescript'];
let firstElem = _.first(arraySample);
let lastElem = _.last(arraySample);
console.log(`First element of Sample Array is ${firstElem}`);
console.log(`Last element of Sample Array is ${lastElem}`);
console.log(`3rd element of Sample Array is ${_.nth(nums, 3)}`);
console.log(`3rd last element of Sample Array is ${_.nth(nums, -3)}`);
Output:
As we can see from the above example, it is quite easy to get any of the elements from an array using the Lodash library.
Generate a Random Number Using Lodash in TypeScript
Lodash also allows us to get the random numbers using a simple function _.random()
. We can provide one or two parameters in this function.
If we provide only one parameter, it will take the starting point from 0. Let’s go through an example and try to generate a random number between 0 to 100 and 50 to 100.
# typescript
const _ = require("lodash");
let randNum = _.random(100);
console.log(randNum);
randNum = _.random(50, 100);
console.log(randNum);
Output:
As we can see from the above example, we can easily get a random number from a range by using this simple function.
Unwrap an Array Using Lodash in TypeScript
There are many situations in which we get a nested array, and we want to convert the nested array into a simple array. Lodash provides an easy function that can be used for unwrapping a nested array.
The function to unwrap an array is known as _.flatten()
. This function can take in two arguments.
The first argument is the nested array that we want to unwrap. The second argument is whether to continue unwrapping the nested array until a simple array is obtained as a result or not; the default value is false.
Let’s go through an example and try to unwrap a nested array, as shown below.
# typescript
const _ = require("lodash");
let simpleNested = _.flatten([1, 2, 3]);;
console.log(simpleNested);
let advNested = _.flatten([[1], [2, 3], [[4, 5]]]);
console.log(advNested);
let advNested2 = _.flatten([[1], [2, 3], [[4, 5]]], true);
console.log(advNested);
Output:
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn