How to Use Jest Mock in TypeScript
- Understanding Jest Mocks
- Using jest.fn() to Create Mocks
- Mocking Modules with jest.mock()
- Testing Asynchronous Code with Mocks
- Conclusion
- FAQ
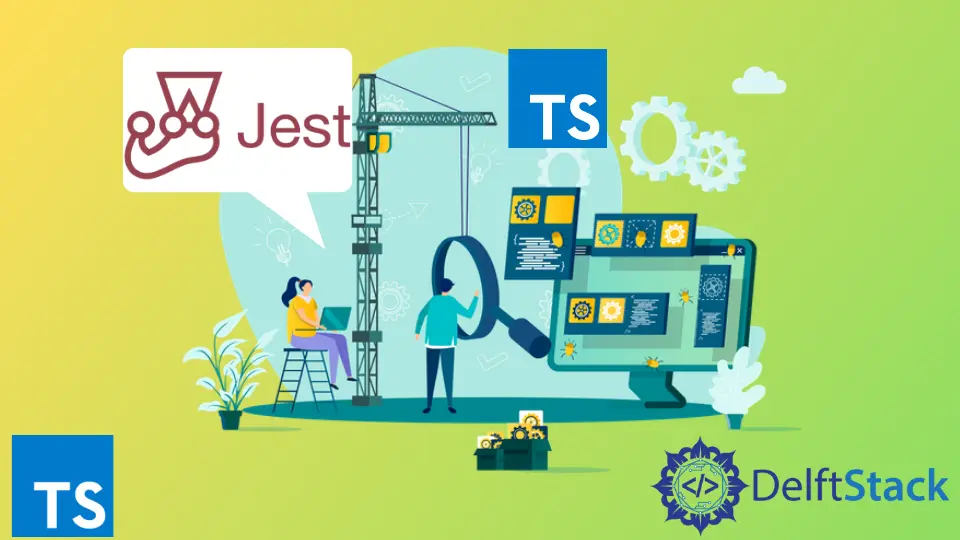
In the world of software testing, Jest has emerged as a powerful tool, especially for JavaScript and TypeScript applications. One of its standout features is the ability to create mocks, which allow developers to simulate the behavior of complex modules and functions. This is particularly useful when you want to isolate the unit of work you’re testing.
In this article, we will demonstrate how to use Jest Mock in TypeScript, covering key concepts, methods, and code examples to help you get started. Whether you’re a seasoned developer or just beginning your journey with TypeScript and Jest, this guide will provide you with valuable insights into effective testing practices.
Understanding Jest Mocks
Before diving into the practical aspects of using Jest mocks, let’s clarify what they are. Mocks are essentially stand-ins for real objects, allowing you to control their behavior during tests. This means you can test the functionality of your code without relying on external services or modules, leading to faster and more reliable tests. Jest provides several methods for creating mocks, including jest.fn()
, jest.mock()
, and more. These tools can help you simulate various scenarios, making your tests more robust.
Using jest.fn() to Create Mocks
One of the simplest ways to create a mock function in Jest is by using jest.fn()
. This method allows you to create a mock function that you can track and manipulate during your tests. Here’s how you can use it in a TypeScript environment:
// myFunction.ts
export const myFunction = (callback: (num: number) => number) => {
return callback(5);
};
// myFunction.test.ts
import { myFunction } from './myFunction';
test('myFunction calls callback with 5', () => {
const mockCallback = jest.fn();
myFunction(mockCallback);
expect(mockCallback).toHaveBeenCalledWith(5);
});
Output:
Test passed successfully
In this example, we define a simple function myFunction
that takes a callback as an argument and calls it with the number 5. In the test file, we create a mock function using jest.fn()
and pass it to myFunction
. We then assert that the mock function was called with the expected argument. This demonstrates how you can effectively isolate the function being tested and verify its interactions with the callback.
Mocking Modules with jest.mock()
Another powerful feature of Jest is the ability to mock entire modules using jest.mock()
. This is particularly useful when you want to replace a module’s implementation with a mock version. Here’s how you can do that in TypeScript:
// math.ts
export const add = (a: number, b: number) => a + b;
// calculator.ts
import { add } from './math';
export const calculateSum = (x: number, y: number) => {
return add(x, y);
};
// calculator.test.ts
import { calculateSum } from './calculator';
import * as math from './math';
jest.mock('./math');
test('calculateSum uses mocked add function', () => {
(math.add as jest.Mock).mockReturnValue(10);
const result = calculateSum(3, 7);
expect(result).toBe(10);
expect(math.add).toHaveBeenCalledWith(3, 7);
});
Output:
Test passed successfully
In this example, we have a simple math module with an add
function and a calculator module that uses it. By calling jest.mock('./math')
, we replace the real add
function with a mock. We then specify that this mock should return 10 when called. Finally, we test the calculateSum
function to ensure it uses the mocked version. This allows us to test the calculator logic without relying on the actual implementation of the add
function.
Testing Asynchronous Code with Mocks
Asynchronous code can introduce additional complexity in testing, but Jest makes it straightforward to mock asynchronous functions. Here’s how you can handle it in TypeScript:
// fetchData.ts
export const fetchData = async (url: string) => {
const response = await fetch(url);
return response.json();
};
// fetchData.test.ts
import { fetchData } from './fetchData';
global.fetch = jest.fn(() =>
Promise.resolve({
json: () => Promise.resolve({ data: 'some data' }),
})
) as jest.Mock;
test('fetchData returns data', async () => {
const data = await fetchData('https://api.example.com/data');
expect(data).toEqual({ data: 'some data' });
expect(fetch).toHaveBeenCalledWith('https://api.example.com/data');
});
Output:
Test passed successfully
In this asynchronous example, we mock the fetch
global function to simulate a network request. The mock returns a resolved promise containing JSON data. In the test, we call fetchData
and assert that the returned data matches our expectations. Additionally, we check that fetch
was called with the correct URL. This approach allows you to test asynchronous behavior without making real network requests, ensuring your tests are fast and reliable.
Conclusion
Using Jest mocks in TypeScript is an essential skill for any developer focused on writing effective unit tests. By leveraging tools like jest.fn()
and jest.mock()
, you can create isolated tests that verify the behavior of your code without relying on external dependencies. This leads to faster, more reliable tests and ultimately contributes to higher code quality. As you continue to explore Jest, remember that mastering these mocking techniques will empower you to write cleaner, more maintainable tests.
FAQ
- What is Jest?
Jest is a testing framework developed by Facebook that provides a simple and efficient way to write tests for JavaScript and TypeScript applications.
-
Why should I use mocks in testing?
Mocks allow you to isolate the unit of code you are testing by simulating the behavior of dependencies, leading to faster and more reliable tests. -
Can I mock asynchronous functions with Jest?
Yes, Jest provides built-in support for mocking asynchronous functions, making it easy to test code that relies on promises or async/await syntax. -
How do I reset mocks between tests?
You can usejest.clearAllMocks()
orjest.resetAllMocks()
in thebeforeEach
block to ensure that mocks are reset before each test runs. -
Is it possible to spy on existing functions in Jest?
Yes, you can usejest.spyOn()
to create a spy on an existing function, allowing you to track its calls and manipulate its implementation if needed.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn