How to Import JSON File in TypeScript
- Import JSON File in TypeScript
-
Use the
import
Method to Import JSON in TypeScript -
Use the
require()
Function to Import JSON in TypeScript - the Wildcard Module in TypeScript
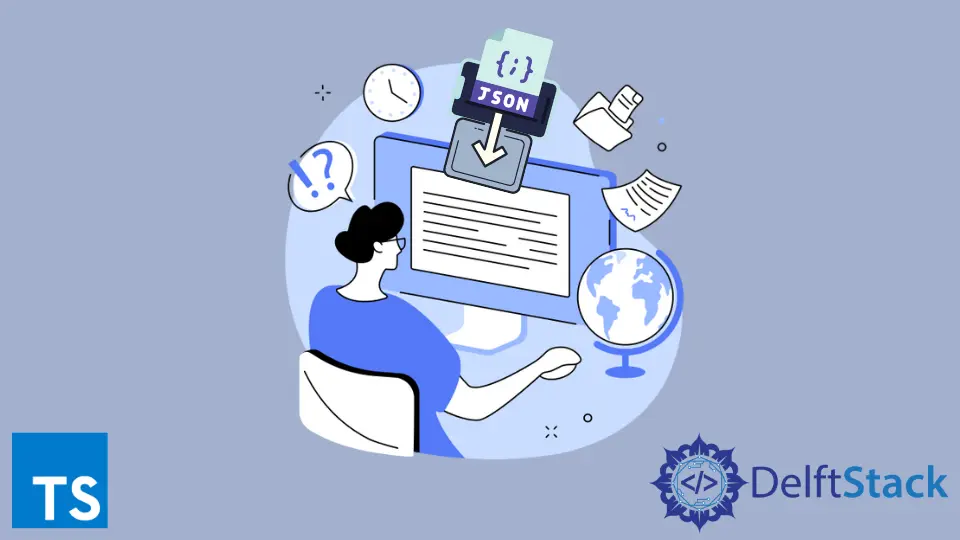
This tutorial demonstrates how we can import JSON files in TypeScript. We will also show a descriptive guide that simplifies the whole process.
The abbreviation of JSON
is JavaScript Object Notation
, a text format used for storing and transporting data.
JSON
is also defined as a lightweight interchange format and a language-independent. The following are an example of dummy JSON:
{
"squadGang": "Super hero squad",
"homeTown": "Metro City",
"invented": 2016,
"secretIndustry": "Super Ship",
"alive": true,
"teamSquad": [
{
"name": "Ant Man",
"age": 33,
"secretRole": "Jukes",
"powers": [
"Radiation Blockage",
"Turning Huge",
"Radiation Waves"
]
},
{
"name": "Randal Uppercut",
"age": 999,
"secretRole": "Jane",
"powers": [
"Million punch",
"Damage Resistance And tackle",
"Superhuman reflexes",
"Spiderman Web"
]
}
]
}
Import JSON File in TypeScript
There are different ways of importing JSON files in TypeScript. Three are the most popular and optimal solutions among the developer community which are:
- Importing JSON in TypeScript with
import
Method. - Importing JSON in TypeScript with
require()
Function. Wildcard
module declaration for JSON files.
Use the import
Method to Import JSON in TypeScript
The TypeScript version 2.9+
is quite easy and simple to import JSON files with the benefits of having features like type-safety and IntelliSense.
Consider having a JSON text with filename power.json
in a folder of FileJSON
having the relative path of FileJson\powers.json
.
[{
"name": "Molecule Man",
"age": 29,
"secretIdentity": "Dan Jukes",
"powers": [
"Radiation resistance",
"Turning tiny",
"Radiation blast"
]
},
{
"name": "Madame Uppercut",
"age": 39,
"secretIdentity": "Jane Wilson",
"powers": [
"Million tonne punch",
"Damage resistance",
"Superhuman reflexes"
]
}
]
In development IDE, it’ll look like this:
You can use JSON files in TypeScript using an import
keyword in the code.
import mode from './FileJson/powers.json';
for(let i = 0;i<mode.length;i++){
console.log(mode[i]);
}
Before starting to import, it is advised by the official documentation of TypeScript to add the following settings in the compilerOptions
section of your tsconfig.json
.
'resolveJsonModule': true, 'esModuleInterop': true,
Output:
You don’t necessarily need esModuleInterop
. It is only necessary for the default import of power.json
.
If you set it to false, then your import
will look like the following:
import * as mode from './FileJson/powers.json';
Use the require()
Function to Import JSON in TypeScript
The require()
function is a built-in function in NodeJS. It includes external modules that exist in separate files.
It also reads and executes the JavaScript file. Then returns the export object.
This can be done by enabling "resolveJsonModule":true
in tsconfig.json
file.
const mode = require('./FileJson/powers.json');
for(let i = 0;i<mode.length;i++){
console.log(mode[i]);
}
Output:
the Wildcard Module in TypeScript
In the TypeScript definition file, i.e., typings.d.ts
, you can declare a module that defines a configuration for every file ending with .json
.
Which is the most suboptimal practice for importing JSON in TypeScript is as follows:
declare module "*.json" {
const dataValue: any;
export default dataValue;
}
It states that all modules with a specific ending in .json
have a single default export. Following are several ways to use a module:
import power from "./FileJson/powers.json";
power.mainPower
Another way is,
import * as power from "./FileJson/powers.json";
power.default.mainPower
Last example to use the module is,
import {default as power} from "./FileJson/powers.json";
power.mainPower
With versions+ 2.9 of TypeScript, you can use the --resolveJsonModule
compiler flag to analyze imported .json
files in TypeScript.
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn