How to Exclude Property in TypeScript
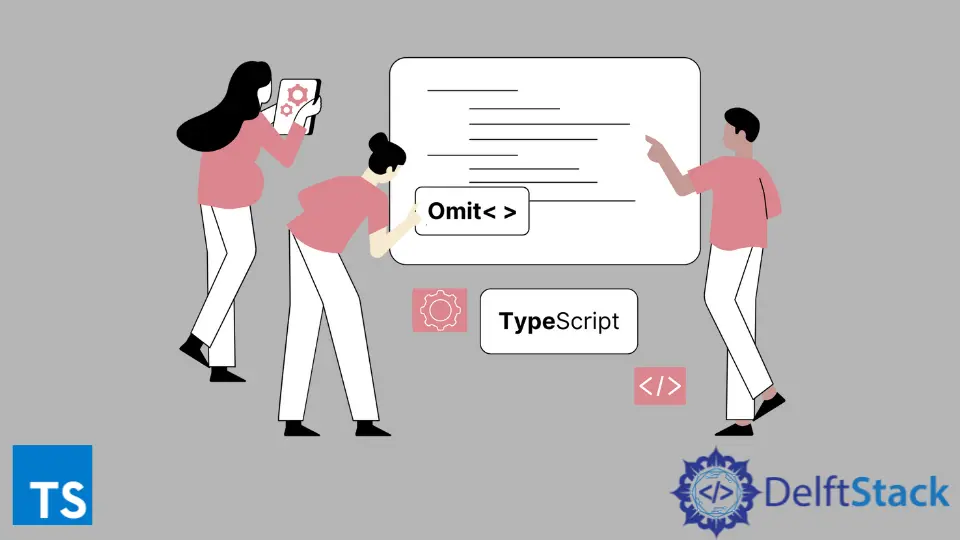
This tutorial will discuss excluding property from a type in TypeScript. An exclude property is used to make another type with less or modified property from an existing type.
Use Omit
to Exclude Property From a Type in TypeScript
TypeScript allows the user to exclude property from a type. The user must use the Omit
type to exclude a property from the existing type.
The Omit
type makes a new type from the existing type.
Syntax:
Omit<Type_Name, 'property'>
Example:
type Info = {
firstName: string,
lastName: string,
age: number,
country: string,
};
type Info1 = Omit<Info, 'country'>;
const info1: Info1 = {
firstName: 'John',
lastName: 'Nash',
age: 27,
};
console.log(info1);
Output:
{
"firstName": "John",
"lastName": "Nash",
"age": 27
}
In the above example, a new type is introduced from the existing type using Omit
. Info
type has four properties, and Info1
has three.
We can even introduce a new type from the existing type Info
and can exclude multiple properties.
Example:
type Info = {
firstName: string,
lastName: string,
age: number,
country: string,
};
type Info1 = Omit<Info, 'country'>;
const info1: Info1 = {
firstName: 'John',
lastName: 'Nash',
age: 27,
};
type Info2 = Omit<Info, 'country' | 'age'>;
const info2: Info2 = {
firstName: 'Stewen',
lastName: 'Gold',
};
console.log(info1);
console.log(info2);
Output:
{
"firstName": "John",
"lastName": "Nash",
"age": 27
}
{
"firstName": "Stewen",
"lastName": "Gold"
}
As seen above, the type Info2
is a modified version of type Info
. Notice how multiple properties are excluded in the Omit
type.
Use Omit
to Modify Property in TypeScript
TypeScript allows the user to modify properties. An error will be raised if the user tries to modify a type without the Omit
type.
Example:
type Info = {
firstName: string,
lastName: string,
age: number,
country: string,
};
type Info1 = Info & {
country: {
countryName: string,
cityName: string,
};
};
const info1: Info1 = {
firstName: 'John',
lastName: 'Nash',
age: 27,
country: {
countryName: 'China',
cityName: 'Wuhan',
},
}
This code has no output because an error will be raised on the object info1
property country
. The error message is: Type countryName and cityName is not assignable to type string
.
To overcome this error, the user must use the omit
type to modify the type.
Example:
type Info = {
firstName: string,
lastName: string,
age: number,
country: string,
};
type Info1 = Omit<Info, 'country'> & {
country: {
countryName: string,
cityName: string,
};
};
const info1: Info1 = {
firstName: 'John',
lastName: 'Nash',
age: 27,
country: {
countryName: 'China',
cityName: 'Wuhan'
},
}
console.log(info1);
Output:
{
"firstName": "John",
"lastName": "Nash",
"age": 27,
"country": {
"countryName": "China",
"cityName": "Wuhan"
}
}
In the above example, notice that Info1
has a modified country
type. Notice the syntax for the updated property.
Using the Omit
type is a good practice to eliminate complexity and make work efficient.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn