How to Handle Exceptions Using try..catch..finally in TypeScript
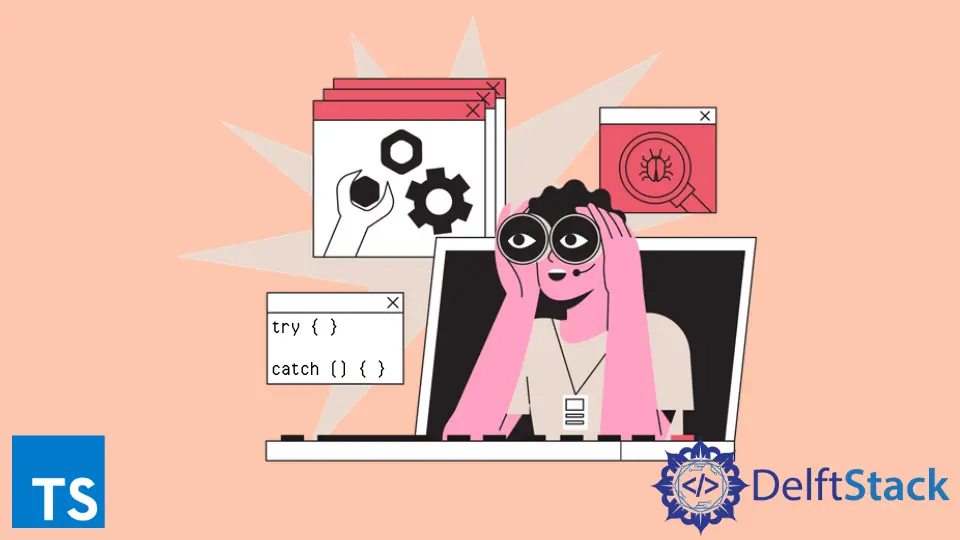
This tutorial will discuss handling exceptions in TypeScript using the try..catch..finally
statement.
Handle Exceptions in TypeScript
In TypeScript, the try..catch..finally
block handles exceptions that arise in the program at run time. It lets the program run correctly and does not end it arbitrarily.
The main code where an exception could arise is placed inside the try
block. If an exception occurs, it goes to the catch
block where it is handled; however, the catch
block is skipped if no error is encountered.
The finally
block will always execute in any case, whether an error arises or not in the program.
Below are some code examples of how we can use try..catch..finally
for exception handling in TypeScript.
function doOrThrow<T>(error: T): true{
if (Math.random() > .5){
console.log('true')
return true;
}
else{
throw error;
}
}
try{
doOrThrow('err1');
doOrThrow('err2');
doOrThrow('err3');
}
catch (e:any){
console.log(e,'error')
}
finally{
console.log("Terminated");
}
Output:
The function inside the try
block is called thrice, where it passes an argument. If the generated number is greater than 0.5, it will execute the if
block and return true
.
Otherwise, it will throw an error, which is handled at the catch
block. It tells which call it is thrown after the execution of try
and catch
.
Consider one more example below.
let fun: number; // Notice use of `let` and explicit type annotation
const runTask=()=>Math.random();
try{
fun = runTask();
console.log('Try Block Executed');
throw new Error("Done");
}
catch(e){
console.log("Error",e);
}
finally{
console.log("The Code is finished executing.");
}
Output:
unknown
Type of catch
Clause Variable
Before version 4.0
of TypeScript, the catch
clause variables have a type of any
. Since the variables have the type any
assigned to them, they lack the type-safety, leading to invalid operations and errors.
Now, version 4.0
of TypeScript allows us to specify the type unknown
of the catch
clause variable, which is much safer than the type any
.
It reminds us that we need to perform a type-check of some sort before operating on values.
try {
// ...
}
catch (err: unknown) {
// error!
// property 'toUpperCase' does not exist on 'unknown' type.
console.log(err.toUpperCase());
if (typeof err === "string") {
// works!
// We have narrowed 'err' down to the type 'string'.
console.log(err.toUpperCase());
}
}
Ibrahim is a Full Stack developer working as a Software Engineer in a reputable international organization. He has work experience in technologies stack like MERN and Spring Boot. He is an enthusiastic JavaScript lover who loves to provide and share research-based solutions to problems. He loves problem-solving and loves to write solutions of those problems with implemented solutions.
LinkedIn