How to Prevent Duplication in SQLite
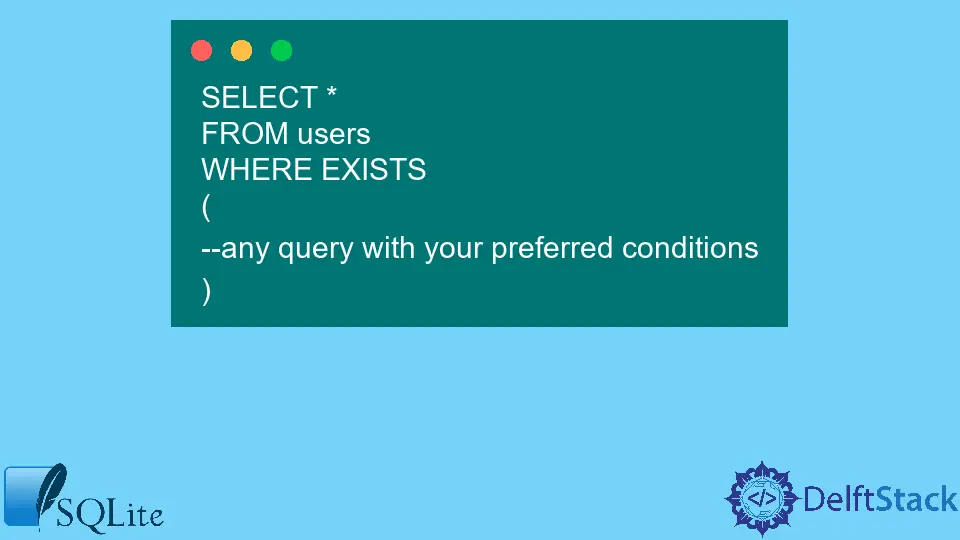
When populating a database, one of the most common issues one might come across is data duplication. This can be problematic for many users, especially those with large databases.
It could have a range of issues, including memory wastage which can be quite costly.
Regardless it is necessary to avoid it ideally during insertion. This will prevent any access data from ever entering the database to begin with.
How to Avoid Duplicate Entries in SQLite
In SQLite, there are multiple ways to avoid duplicate entries. The main reasons that differentiate each of these methods are:
- The ease of applying them.
- The rate of effectiveness.
- The requirements of your database.
Manual Removal
Before advanced database queries, the norm was to go through entries and identify any duplication. This can still work for databases with very few records.
However, this is inefficient in all cases and unusable for much larger databases. These issues are why this method is hardly ever used.
Use Advanced Nested Queries
Advanced nested queries can do anything if they are applied correctly. However, this is often unappreciated as well.
Designing them is often complex and might not be accurate in all cases. Therefore, although this method works, it is unadvised as simpler methods exist.
the UNIQUE
Constraint
This constraint is often applied in SQLite during table creation. When applied to a row, it prevents users from entering duplicate entries into a database in the same row.
This constraint is also automatically applied when a primary key constraint is used. You can create a table and apply the unique constraint with the following code.
CREATE TABLE users(
users_id INTEGER,
user_age INTEGER,
UNIQUE(users_id, lessoninfo_id)
);
You may also apply it directly as follows:
CREATE TABLE users(
users_id INTEGER UNIQUE,
user_age INTEGER
);
If you have already created your table and need to add a modification to make any row unique, you will have to delete the whole table. You can also opt for a unique index, as shown in the code below.
CREATE UNIQUE INDEX index_name
ON users(users_id);
the EXISTS
Clause
Exists
is often used in queries and nested ones. It is easy to reduce complex queries into much more simplified ones.
The inverse is the NOT EXISTS
clause, which means the exact opposite. This can be used in queries after insertion, as shown below.
First, make sure your table is already created. You may use the following code to do so.
CREATE TABLE users(
users_id INTEGER,
user_age INTEGER
);
After this, begin creating your query. You can refer to the code below to use the clause correctly.
SELECT *
FROM users
WHERE EXISTS
(
--any query with your preferred conditions
)
Note: Here, if
EXISTS
were replaced withNOT EXISTS
, you would get the opposite result.
As explained below, you can also apply this logic directly during insertion using the clause differently.
Insert if NOT EXISTS
While creating a query that inserts values to your table in a database, the best and probably most commonly used method is using insert if not exists
.
This can be considered a better way to use the not exists
clause by combining it with the query where you insert data. An example of this is shown in the code below.
First, create your table.
CREATE TABLE users(
users_id INTEGER,
user_age INTEGER
);
Then, design a query such as the one in the code below.
INSERT INTO users(users_id,user_age)
SELECT * /*user whose id isn't 1.*/
WHERE NOT EXISTS
(
SELECT 1 FROM memos WHERE id = 5 AND name= 'abc'
);
As the label suggests, this query will only display those users, or data entries, where the user id
is not 1. You can always change the condition by altering the query in the brackets to whatever your requirements are.
INSERT OR IGNORE
Another method that would successfully prevent you from adding any redundancy in SQLite is the insert or ignore
clause. If the insertion violates any predefined terms, the insertion will be ignored.
Your query will run successfully, but no insertion will be made.
Let’s take another example where a primary key
exists. In a primary key
, the unique constraint exists, which means duplicate entries will not be accepted.
A table is first created with a primary key
as follows:
CREATE TABLE users
(
id INTEGER NOT NULL PRIMARY KEY,
age INTEGER
);
We can insert a value with the ignore
condition, as shown below.
INSERT OR IGNORE
INTO users(id, age) VALUES(123, 24)
If we were to assume that a user with id 123
already exists, this insertion would not take place, and there will be no changes to the original table.
Note: If you don’t have a unique constraint on the column you’re inserting the values into, the record will always be inserted.
the REPLACE
Clause
Instead of using the ignore
clause, you may also opt for replacing it. This is similar to the ignore
clause, as it first checks whether the values inserted are unique if the unique constraint (or primary key
) has been applied to a certain row.
The difference here is that if the record with the unique constraint already exists, it will replace it with the new record that you are inserting.
INSERT OR REPLACE
INTO users(id, age) VALUES(123, abc)
Here, if a user with id 123
already exists in the table, the name will be replaced with abc
.
That’s everything you need to know about avoiding duplicate entries in SQLite. We hope you now know exactly what to do to meet your needs and produce the most efficient database.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub