How to Plot the Graph Using the seaborn.lmplot() Function
- What is seaborn.lmplot()?
- Basic Usage of seaborn.lmplot()
- Customizing the Plot with seaborn.lmplot()
- Adding Regression Lines and Confidence Intervals
- Conclusion
- FAQ
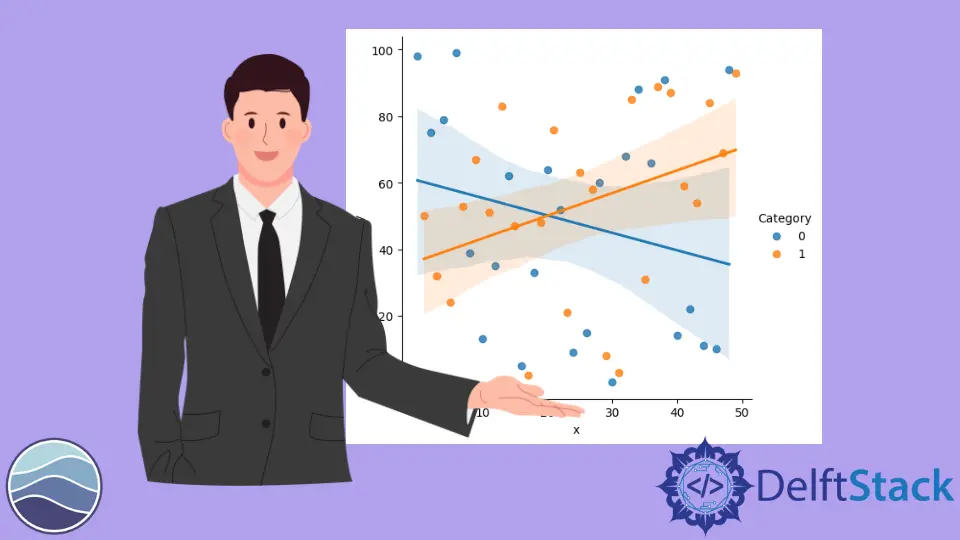
In the realm of data visualization, the seaborn library in Python stands out for its ease of use and impressive capabilities. One of its most popular functions is seaborn.lmplot()
, which allows you to create linear regression plots effortlessly.
This tutorial will guide you through the process of using lmplot()
to visualize relationships between variables in your dataset. Whether you’re a beginner or an experienced data analyst, understanding how to effectively use this function can enhance your data storytelling. From setting up your environment to customizing your plots, we’ll cover everything you need to know to get started with seaborn’s lmplot()
function.
What is seaborn.lmplot()?
The seaborn.lmplot()
function is designed to visualize the relationship between two continuous variables by fitting a linear regression model. This function not only plots the data points but also includes a regression line, making it easier to interpret the relationship between the variables. It can handle multiple subsets of data and offers various customization options, such as changing the color palette or adding additional regression lines.
To begin using lmplot()
, you’ll first need to install seaborn if you haven’t already. You can do this using pip:
pip install seaborn
Once you have seaborn installed, you can start plotting your graphs.
Basic Usage of seaborn.lmplot()
To create a basic linear regression plot, you need to provide your data and specify the x and y variables. Below is a simple example using a dataset that contains information about the relationship between hours studied and exam scores.
import seaborn as sns
import matplotlib.pyplot as plt
import pandas as pd
# Sample data
data = {
'Hours_Studied': [1, 2, 3, 4, 5, 6],
'Exam_Score': [50, 55, 65, 70, 80, 90]
}
df = pd.DataFrame(data)
# Create lmplot
sns.lmplot(x='Hours_Studied', y='Exam_Score', data=df)
plt.title('Hours Studied vs Exam Score')
plt.show()
In this example, we first import the necessary libraries: seaborn for visualization, matplotlib for plotting, and pandas for data manipulation. We then create a simple dataset using a dictionary and convert it into a DataFrame. The lmplot()
function takes the x and y variables as parameters, along with the dataset, and generates a scatter plot with a fitted regression line. The title of the plot is set using plt.title()
before displaying the plot with plt.show()
.
This basic usage is just the starting point. The true power of lmplot()
lies in its ability to customize and enhance your plots for better insights.
Customizing the Plot with seaborn.lmplot()
Customization is key when it comes to making your plots more informative and visually appealing. The lmplot()
function allows you to modify various aspects of your plot. For instance, you can change the color, add a hue for categorical variables, and adjust the markers. Here’s how you can customize your plot:
# Sample data with a categorical variable
data = {
'Hours_Studied': [1, 2, 3, 4, 5, 6, 1, 2, 3, 4, 5, 6],
'Exam_Score': [50, 55, 65, 70, 80, 90, 55, 60, 70, 75, 85, 95],
'Gender': ['Male', 'Male', 'Male', 'Male', 'Male', 'Male',
'Female', 'Female', 'Female', 'Female', 'Female', 'Female']
}
df = pd.DataFrame(data)
# Create customized lmplot
sns.lmplot(x='Hours_Studied', y='Exam_Score', data=df, hue='Gender', palette='muted', markers=['o', 's'])
plt.title('Hours Studied vs Exam Score by Gender')
plt.show()
In this example, we introduce a new categorical variable, “Gender.” The hue
parameter is used to differentiate the data points based on gender, while the palette
parameter allows you to choose a color scheme. Additionally, the markers
parameter specifies different markers for each gender. This customization helps in visualizing how the relationship varies between different groups within your dataset.
Adding Regression Lines and Confidence Intervals
One of the standout features of lmplot()
is its ability to show confidence intervals along with the regression line. By default, seaborn includes a 95% confidence interval around the regression line, which gives you a sense of the uncertainty associated with the estimate. You can also adjust the confidence level if needed.
# Create lmplot with confidence intervals
sns.lmplot(x='Hours_Studied', y='Exam_Score', data=df, ci=99)
plt.title('Hours Studied vs Exam Score with 99% Confidence Interval')
plt.show()
Output:
A scatter plot with a regression line and a 99% confidence interval shaded around it.
In this example, we set the confidence interval to 99% using the ci
parameter. The shaded area around the regression line represents the uncertainty of the predicted values. This is particularly useful when you want to convey the reliability of your regression model to your audience.
Conclusion
The seaborn.lmplot()
function is a powerful tool for visualizing linear relationships between variables in Python. With its straightforward syntax and extensive customization options, it allows you to create informative and aesthetically pleasing plots. Whether you’re working with simple datasets or more complex ones involving categorical variables, lmplot()
can help you convey your findings effectively. By mastering this function, you can elevate your data visualization skills and enhance your analytical capabilities.
FAQ
-
what is the purpose of seaborn.lmplot()?
seaborn.lmplot() is used to visualize the relationship between two continuous variables by fitting a linear regression model. -
can I customize the colors in my lmplot?
yes, you can customize the colors using the hue parameter and various color palettes available in seaborn. -
how do I add multiple regression lines in lmplot?
you can add multiple regression lines by using the hue parameter to differentiate between categories in your dataset. -
can I change the confidence interval level in lmplot?
yes, you can change the confidence interval level using the ci parameter in the lmplot() function. -
what libraries do I need to use lmplot()?
you need to have seaborn and matplotlib installed, and pandas is often used for data manipulation.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn