How to Reverse Log Scale in Seaborn Plots
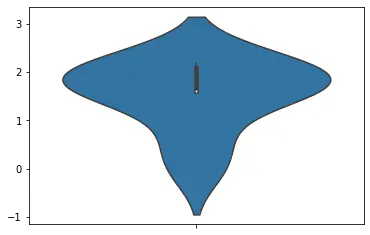
Python is well equipped with different functions to deal with logarithmic and exponential values. We use the log()
and exp()
functions available from the numpy and math modules to calculate such values.
For example,
print(np.log(50))
print(np.exp(3.912023005428146))
Output:
3.912023005428146
49.99999999999999
Many alterations of the above two functions allow us to calculate the log with different bases and more. Exponential values are considered inverse log or reverse log values.
It is possible to deal with such values on graphs and plots also. The seaborn module, which is based on the matplotlib module, is used for visualization purposes and can create many different types of graphs. We can tweak the final figure as per our requirement using different functions.
In this tutorial, we will learn to reverse log values on seaborn plots in Python.
In our example, we will use the log functions to calculate the required values and plot them using the violinplot()
function of the seaborn module.
See the code below,
import seaborn as sns
import numpy as np
lst = [1, 5, 8, 9, 5, 2, 5, 6, 9]
pl = sns.violinplot(y=np.log(lst))
We can customize the label tick values on both axis using the set_yticklabels()
and set_xticklabels()
function. We will use the reverse log function with the above functions to set the tick label values.
For example,
import seaborn as sns
import numpy as np
lst = [1, 5, 8, 9, 5, 2, 5, 6, 9]
pl = sns.violinplot(y=np.log(lst))
pl.set_yticklabels([f"{np.expm1(l):.2f}" for l in pl.get_yticks()])
The get_yticks()
function returns the default labels and we calculate their reverse log using the exp()
function. We format the final result to only two decimal places using fstrings used for string formatting in Python.
This way, we created a violin plot by calculating the log values of elements of a list and mentioning the original values on the tick labels using the reverse log function.
Note that we used the functions from the numpy module and not the math module in our examples. It is because that the numpy log()
and exp()
functions can directly calculate the required values of a list or an array also.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn