Synchronization in Scala
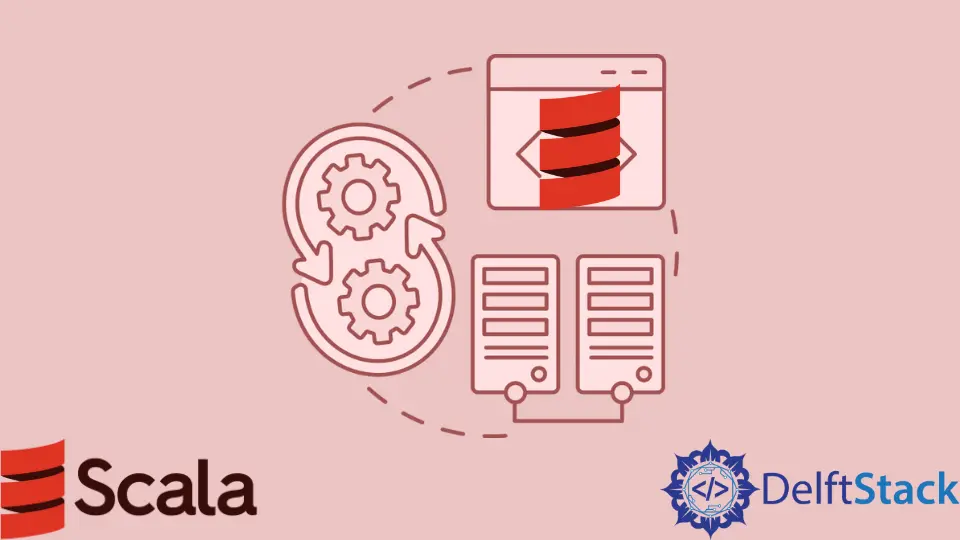
In this article, we will learn about synchronization in Scala.
In the present-day scenario, many applications need to support concurrency and parallelism. So when we have multiple processes or threads working on shared resources, we need a mechanism to avoid any inconsistency.
This is where synchronization comes into play.
Syntax of synchronized
in Scala
We can use Java classes to implement synchronization in Scala.
Syntax:
synchronized{
//our code here
}
We can create a synchronized block using Scala’s synchronized
keyword. Inside this block, we can write the code we want to synchronize.
This will prevent our resources from being modified by multiple threads simultaneously, maintaining data consistency.
Use Synchronized in Scala
We can use synchronization whenever we have shared resources, and we don’t want them to be modified simultaneously by various processes/threads. This is crucial in a real-world scenario as transactions or calculations depend on other data.
If we don’t synchronize, it might lead to inconsistency.
Let’s take a bank transaction example to understand this better.
Suppose A and B can access our joint account with 2000 dollars in it. Now, both of them want to withdraw the money simultaneously, but this is not possible because, at a time, only one person can get the amount.
So the account, which is a shared resource, has to be locked for some time. When the first person, let’s say B, is done with their operations, we only release the lock and allow the other person to access the resource so that any inconsistency does not occur.
Some points we must keep in mind while working with synchronization in Scala:
- It makes the program’s execution time very slow because only one thread or process is allowed to access the shared part at a time, and it could be possible that we have thousands of such threads trying to access the resources.
- During synchronization, executing a thread holds the lock so that no other can enter the shared part of the data, also termed a critical region. Meanwhile, all the other threads go into a waiting state until the current one finishes its execution.
- We should use the synchronization approach only when we depend on the previous value, affecting our current value in a concurrent and multiprogramming environment.
Example code:
object workspace extends App {
synchronized {
println("We are synchronizing")
for (i<- 0 to 5) {
println(i)
}
}
}
We will be printing the value of the integer from 0 to 5 inside the synchronized block.
Output:
We are synchronizing
0
1
2
3
4
5
Conclusion
We avoid data inconsistency in our application through synchronization, but it makes our application a bit slower as only one thread can execute the block at a time.
This can be avoided when using multicore and parallel distributed processing systems where different instances of the same code and threads can run on them.