How to Write SQL Queries in Scala
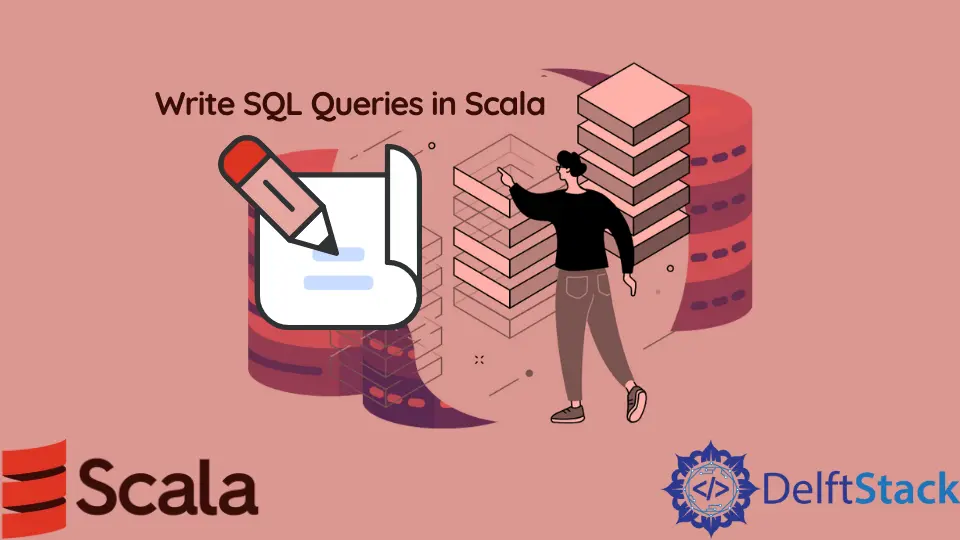
Writing SQL queries in Scala can be a powerful way to leverage the strengths of both languages. Scala, known for its functional programming capabilities, can seamlessly integrate with SQL databases, allowing developers to write complex queries with ease.
This article will guide you through the process of writing SQL queries in Scala, focusing on best practices, common methods, and practical examples. Whether you’re a seasoned developer or just starting out, you’ll find valuable insights to enhance your SQL skills within the Scala environment. Let’s dive in!
Understanding Scala’s Database Connectivity
To write SQL queries in Scala, you typically use libraries like JDBC (Java Database Connectivity) or frameworks like Slick. JDBC provides a standard method for connecting to databases, while Slick offers a more idiomatic way to write SQL queries in a Scala-like syntax. Both methods have their advantages, and your choice will depend on your project requirements.
Using JDBC for SQL Queries
JDBC is a low-level API that allows you to interact directly with databases. Here’s how you can write SQL queries using JDBC in Scala.
import java.sql.{Connection, DriverManager, ResultSet}
object JdbcExample {
def main(args: Array[String]): Unit = {
val url = "jdbc:mysql://localhost:3306/mydatabase"
val username = "root"
val password = "password"
var connection: Connection = null
try {
connection = DriverManager.getConnection(url, username, password)
val statement = connection.createStatement()
val resultSet: ResultSet = statement.executeQuery("SELECT * FROM users")
while (resultSet.next()) {
println(s"User ID: ${resultSet.getInt("id")}, Name: ${resultSet.getString("name")}")
}
} catch {
case e: Exception => e.printStackTrace()
} finally {
if (connection != null) connection.close()
}
}
}
Output:
User ID: 1, Name: John Doe
User ID: 2, Name: Jane Smith
In this example, we first import the necessary JDBC classes and establish a connection to the MySQL database. Then, we create a statement to execute our SQL query. The results are processed in a loop, where we retrieve each user’s ID and name. Finally, we ensure the connection is closed to prevent resource leaks.
Using Slick for SQL Queries
Slick is a functional relational mapping (FRM) library for Scala that allows you to write SQL queries in a more Scala-friendly way. Below is an example of how to use Slick to query a database.
import slick.jdbc.MySQLProfile.api._
import scala.concurrent.ExecutionContext.Implicits.global
import scala.concurrent.Await
import scala.concurrent.duration._
case class User(id: Int, name: String)
class Users(tag: Tag) extends Table[User](tag, "users") {
def id = column[Int]("id", O.PrimaryKey)
def name = column[String]("name")
def * = (id, name) <> (User.tupled, User.unapply)
}
object SlickExample extends App {
val db = Database.forConfig("mydb")
val users = TableQuery[Users]
val query = users.result
val future = db.run(query)
val result = Await.result(future, 2.seconds)
result.foreach(user => println(s"User ID: ${user.id}, Name: ${user.name}"))
}
Output:
User ID: 1, Name: John Doe
User ID: 2, Name: Jane Smith
In this Slick example, we define a case class User
to represent our data model. The Users
class extends Table
, defining the schema of our users
table. We create a query to fetch all users and execute it asynchronously. The results are then printed to the console. Slick abstracts much of the boilerplate code associated with JDBC, making your code cleaner and more maintainable.
Conclusion
Writing SQL queries in Scala can be straightforward and efficient with the right tools. Whether you opt for JDBC for more control or Slick for a more idiomatic approach, both methods have their unique benefits. By integrating SQL queries into your Scala applications, you can harness the full power of both languages, leading to more robust and scalable applications. As you continue your journey with Scala and SQL, remember to explore the extensive documentation and community resources available to deepen your understanding. Happy coding!
FAQ
-
How do I connect to a database in Scala?
You can connect to a database in Scala using JDBC or libraries like Slick. JDBC requires setting up a connection using DriverManager, while Slick provides a more idiomatic approach. -
What is Slick in Scala?
Slick is a functional relational mapping library for Scala that allows developers to write SQL queries in a Scala-like syntax, making database interactions more seamless and type-safe. -
Can I use Scala with any SQL database?
Yes, Scala can be used with any SQL database that has a JDBC driver, including MySQL, PostgreSQL, and SQLite. -
What are the advantages of using Slick over JDBC?
Slick offers a more concise and expressive syntax, better type safety, and the ability to work with asynchronous database queries, making it easier to manage database interactions in Scala. -
Is it necessary to close the database connection in Scala?
Yes, it is important to close the database connection to release resources and avoid potential memory leaks.