Sealed Traits in Scala
- Understanding Sealed Traits
- Benefits of Using Sealed Traits
- When to Use Sealed Traits
- Conclusion
- FAQ
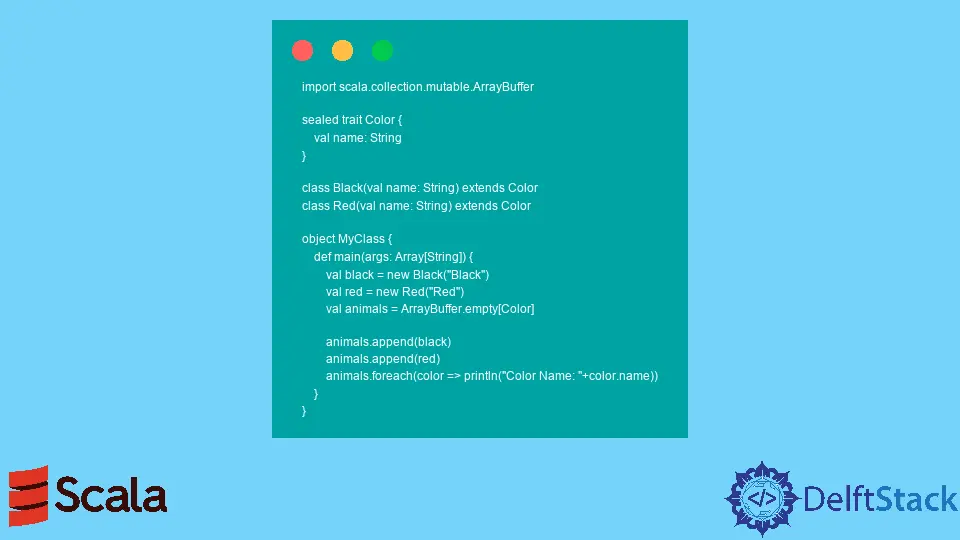
Scala is a powerful programming language that blends functional and object-oriented programming paradigms. One of its unique features is the concept of sealed traits, which play a crucial role in type safety and pattern matching.
In this article, we will explore sealed traits in Scala, how they work, and why they are beneficial for developers. Sealed traits allow you to define a limited set of subclasses, which can lead to more predictable code and fewer runtime errors. By the end of this article, you will have a solid understanding of sealed traits and how to effectively use them in your Scala projects.
Understanding Sealed Traits
Sealed traits are an integral part of Scala’s type system, designed to restrict the inheritance of a trait to a specific set of subclasses. When you declare a trait as sealed, you are essentially telling the compiler that all subclasses must be defined within the same file. This feature is particularly useful for pattern matching, as it ensures that all possible cases are known at compile time, reducing the risk of runtime exceptions.
Here’s a simple example to illustrate sealed traits:
sealed trait Animal
case class Dog(name: String) extends Animal
case class Cat(name: String) extends Animal
In this example, we define a sealed trait Animal
and two case classes, Dog
and Cat
, that extend it. Since Animal
is sealed, any other subclasses of Animal
must be defined in the same file. This restriction allows the Scala compiler to enforce exhaustive pattern matching.
The main advantage of using sealed traits is that they enhance type safety. When using pattern matching, if you forget to handle a case, the compiler will issue a warning. This feature helps you catch potential bugs early in the development process, making your code more robust and maintainable.
Benefits of Using Sealed Traits
Sealed traits offer several advantages that make them a preferred choice for many Scala developers. Here are some of the key benefits:
-
Exhaustive Pattern Matching: Since all subclasses must be defined in the same file, the compiler can ensure that all possible cases are handled during pattern matching. This leads to safer and more predictable code.
-
Improved Readability: By limiting the number of subclasses, sealed traits make it easier to understand the hierarchy of types. Developers can quickly see all possible implementations of a trait, which enhances code readability.
-
Better Refactoring Support: When you change a sealed trait, the compiler will help you identify all affected subclasses. This feature simplifies the refactoring process, as you can be confident that all related code is accounted for.
-
Encapsulation: Sealed traits allow you to encapsulate related types within a single file. This organization can reduce the chance of name clashes and makes it easier to manage and maintain your codebase.
By leveraging the benefits of sealed traits, developers can write more reliable and maintainable Scala applications. Whether you’re building a small project or a large-scale system, understanding sealed traits is essential for effective Scala programming.
When to Use Sealed Traits
Knowing when to use sealed traits can significantly influence the design and architecture of your Scala applications. Here are some scenarios where sealed traits are particularly useful:
-
Defining a Finite Set of Types: If you have a known set of related types, sealed traits are an excellent choice. For example, when modeling different payment methods (CreditCard, PayPal, etc.), a sealed trait can clearly define these types.
-
Implementing State Machines: Sealed traits can be used to represent different states in a state machine. Each state can be a case class extending the sealed trait, ensuring that all states are accounted for in pattern matching.
-
Handling Domain-Specific Languages (DSLs): When creating a DSL, sealed traits can help define the various constructs in a clear and type-safe manner. This approach allows you to enforce rules and constraints at compile time.
- Error Handling: Sealed traits can be utilized to model different error types in an application. By defining a sealed trait for errors, you can ensure that all possible error cases are handled, improving the robustness of your error handling logic.
In summary, sealed traits are an excellent tool for defining a closed set of types in your Scala applications. They provide type safety, improve code readability, and facilitate easier refactoring. By understanding when to use them, you can enhance the quality of your code and reduce potential bugs.
Conclusion
In conclusion, sealed traits are a powerful feature of Scala that can greatly enhance your programming experience. They provide a way to define a restricted set of subclasses, ensuring type safety and making pattern matching more predictable. By using sealed traits, you can improve the readability of your code, simplify refactoring, and encapsulate related types effectively. Whether you’re a beginner or an experienced Scala developer, understanding sealed traits is essential for writing robust and maintainable code. Embrace this feature in your next Scala project, and you’ll likely find it a valuable addition to your programming toolkit.
FAQ
-
What are sealed traits in Scala?
Sealed traits are traits that restrict the inheritance to a limited set of subclasses defined within the same file, enhancing type safety and pattern matching. -
How do sealed traits improve pattern matching?
Sealed traits ensure that all subclasses are known at compile time, allowing the compiler to enforce exhaustive pattern matching and catch potential bugs early. -
Can I extend sealed traits in different files?
No, subclasses of a sealed trait must be defined in the same file. This restriction helps maintain type safety and predictability in your code. -
When should I use sealed traits?
Use sealed traits when you have a finite set of related types, when implementing state machines, creating domain-specific languages, or modeling error handling. -
Are sealed traits only for classes?
Sealed traits can be extended by case classes or regular classes, making them flexible for various use cases in Scala.