How to Parse JSON Strings in Scala
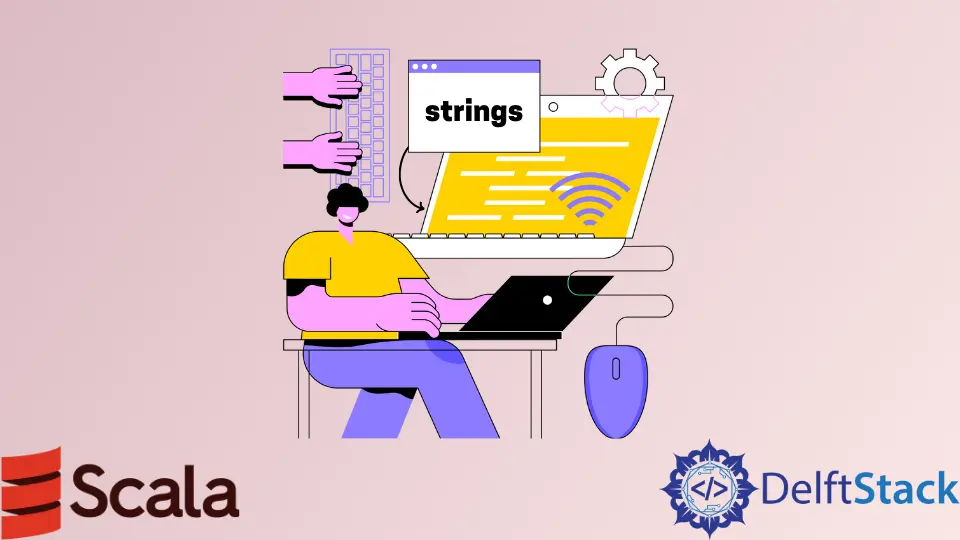
This article will teach us how to parse JSON strings in the Scala programming language.
JSON is a common format to exchange to and fro from a web server. So the objective here is that given a JSON string with an array of objects, we want to deserialize it into Scala objects so we can use it in our application.
We can parse the JSON using the plain Scala methods and features or use different APIs and libraries to parse JSON files like Lift-JSON library and Circe.
Use Option
to Parse JSON Strings in Scala
We can use Option
along with pattern match in Scala to parse a JSON string. Let’s assume we have the following JSON string:
{
"languages": [{
"name": "English",
"is_active": true,
"completeness": 4.5
}, {
"name": "Japanese",
"is_active": true,
"completeness": 1.4
}]
}
We can use the below code to map it to Scala objects:
class parse[T]
{
def unapply(X:Option[Any]):Option[T] = if(X.isEmpty) {
None
} else {
Some(X.get.asInstanceOf[T])
}
}
object A extends parse[Map[String,Any]]
object B extends parse[List[Any]]
object C extends parse[String]
object D extends parse[Double]
object E extends parse[Boolean]
for{
A(mp) <- List(JSON.parseFull(str))
B(languages) = mp.get("languages")
language<-languages
A(temp) = Some(language)
C(store_name) = temp.get("name")
E(check_active) = temp.get("is_active")
D(completeness_level) = temp.get("completeness")
}
yield{
(store_name,check_active,completeness_level)
}
Use APIs to Parse JSON Strings in Scala
We can use a well-known Lift-JSON library to parse the JSON string. This library contains many methods which could be used to deserialize the JSON string into Scala objects.
Example code:
import net.liftweb.json.DefaultFormats
import net.liftweb.json._
case class Employee(
Name: String,
id: Int,
username: String,
password: String,
DepartmentsWorking: List[String]
)
object ParseJsonArray extends App {
implicit val formats = DefaultFormats
val str ="""
{
"employees": [
{ "Account": {
"Name": "YMail",
"id": 3,
"username": "srj",
"password": "123456srj",
"DepartmentsWorking": ["sales", "IT", "HR"]
}},
]}
"""
val json = parse(str)
val contents = (json \\ "Account").children
for (temp <- contents) {
val m = temp.extract[Employee]
println(s"Employee: ${m.Name}, ${m.id}")
println(" Departments working: " + m.DepartmentsWorking.mkString(","))
}
}
In the above code, we have created a case class Employee
to match the JSON data.
The advantage of using APIs is that they help us write more concise code and provide many functionalities that primitive Scala methods might not provide.
Conclusion
In this article, we saw two methods one where we used plain old Scala methods and another where we used another method where we used a well-known API to parse the JSON string. And we understood that APIs are always preferred while parsing due to the simplicity of writing and its functionalities.