How to Return Boolean With If-Else in Scala
- Understanding If-Else in Scala
- Returning Boolean from If-Else
- Simplifying Boolean Return with Expression
- Using If-Else with Multiple Conditions
- Conclusion
- FAQ
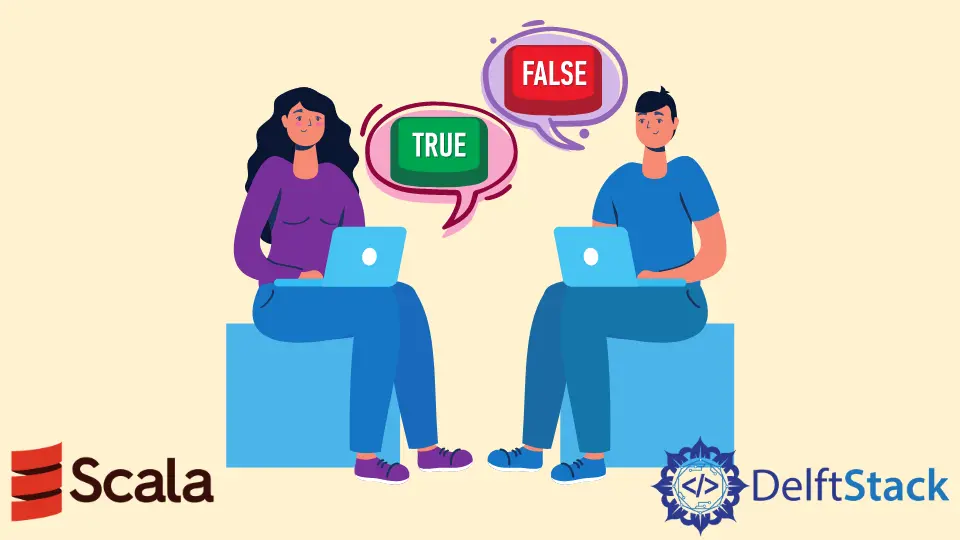
In the world of programming, returning Boolean values is a fundamental aspect, especially when using conditional statements. In Scala, the if-else construct allows developers to evaluate conditions and return Boolean values effectively. Understanding how to leverage this feature can enhance your coding efficiency and make your Scala applications more robust.
This article will guide you through the process of returning Boolean values using if-else statements in Scala, complete with clear examples and detailed explanations. Whether you’re a beginner or looking to brush up on your Scala skills, this guide is designed to help you master this essential concept.
Understanding If-Else in Scala
The if-else statement in Scala is a powerful tool for decision-making in your code. It allows you to execute different blocks of code based on whether a condition evaluates to true or false. The basic syntax is straightforward:
if (condition) {
// code to execute if condition is true
} else {
// code to execute if condition is false
}
When using if-else, you can directly return a Boolean value. For instance, you might want to check if a number is even or odd. This is where the true power of if-else shines, as it allows you to encapsulate logic cleanly and concisely.
Returning Boolean from If-Else
Returning a Boolean value from an if-else statement in Scala is as simple as placing the condition inside the parentheses. Let’s look at a straightforward example where we check if a number is positive:
def isPositive(number: Int): Boolean = {
if (number > 0) {
true
} else {
false
}
}
val result = isPositive(10)
println(result)
Output:
true
In this example, we define a function isPositive
that takes an integer as an argument. Inside the function, we use an if-else statement to check if the number is greater than zero. If it is, we return true
; otherwise, we return false
. The output confirms that the function correctly identifies 10 as a positive number.
Simplifying Boolean Return with Expression
Scala allows you to simplify the return of Boolean values even further by using a single expression. Instead of using an if-else block, you can directly return the result of the condition. Here’s how you can do this:
def isNegative(number: Int): Boolean = {
number < 0
}
val result = isNegative(-5)
println(result)
Output:
true
In this case, the isNegative
function checks if the number is less than zero and returns the result directly. This approach is more concise and leverages Scala’s ability to return the result of a Boolean expression without needing an explicit if-else structure. This not only makes your code cleaner but also enhances readability.
Using If-Else with Multiple Conditions
Sometimes, you may need to evaluate multiple conditions before returning a Boolean value. Scala’s if-else construct can handle this elegantly. Let’s consider an example where we check if a number is within a specific range:
def isWithinRange(number: Int, lower: Int, upper: Int): Boolean = {
if (number >= lower && number <= upper) {
true
} else {
false
}
}
val result = isWithinRange(15, 10, 20)
println(result)
Output:
true
In this function, isWithinRange
, we check if the given number is between the specified lower and upper bounds. The if-else statement evaluates the condition using logical operators, and the function returns true
or false
based on the evaluation. This is particularly useful in scenarios where you need to validate input or enforce specific business rules.
Conclusion
Returning Boolean values using if-else statements in Scala is an essential skill for any developer. Whether you’re checking if a number is positive, negative, or within a specific range, understanding how to structure your conditions efficiently can significantly enhance your programming capabilities. By simplifying your code with direct Boolean expressions and handling multiple conditions gracefully, you can write cleaner, more maintainable Scala applications. Embrace these techniques, and you’ll find your coding experience more enjoyable and productive.
FAQ
-
how do you return a Boolean in Scala?
You can return a Boolean in Scala using if-else statements or by directly returning the result of a Boolean expression. -
can you use if-else in a single line in Scala?
Yes, Scala allows you to use a single line for if-else statements by returning the result of the condition directly. -
what are the advantages of using if-else in Scala?
The advantages include clear decision-making, concise code, and the ability to handle multiple conditions effectively. -
how do you check multiple conditions in Scala?
You can check multiple conditions using logical operators like&&
(and) and||
(or) within an if-else statement. -
is it possible to use if-else without curly braces in Scala?
Yes, if the if-else statement contains a single expression, you can omit the curly braces for a more concise syntax.