How to Return a Value in Scala
- Return an Integer Value in Scala
- Return an Anonymous Value in Scala
- Return a List or Vector Value in Scala
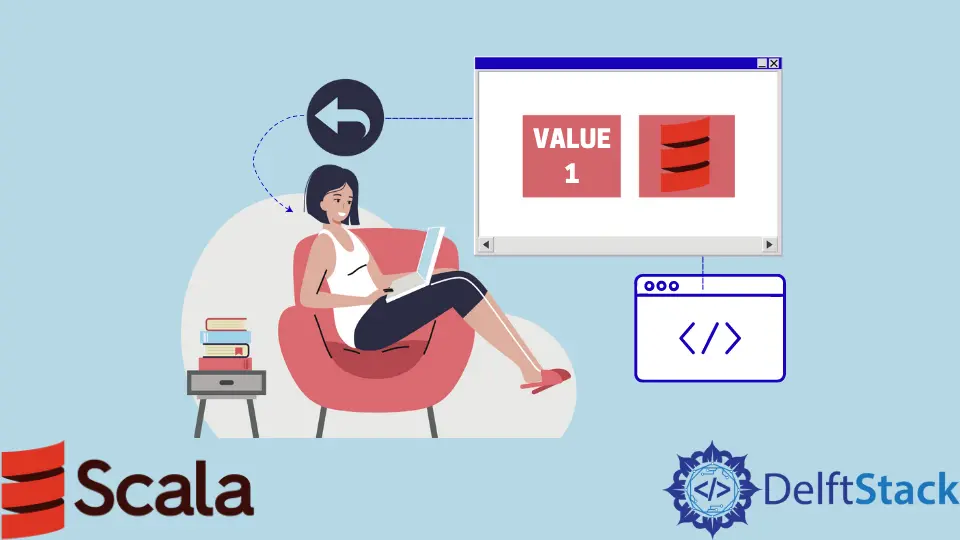
This tutorial will discuss how to return a value from a function in Scala.
Return an Integer Value in Scala
In Scala, a function may or may not return any value to the caller function. It depends on the function implementation.
A function that returns a value can specify in its signature so that the caller will get to know what type of value will be returned. We created a function that takes two integer arguments and returns an integer in this example.
Code:
object MyClass {
def main(args: Array[String]) {
var result = getSum(10,20);
print(result);
}
def getSum( a:Int, b:Int ) : Int = {
var sum:Int = 0
sum = a + b
return sum
}
}
Output:
30
Return an Anonymous Value in Scala
We declare a getSum()
that takes two integer arguments but does not specify the return type. Now, it’s up to the Scala interpreter to infer the type of return value by the last expression of the function.
The function implemented in the example below returns an integer value.
Code:
object MyClass {
def main(args: Array[String]) {
var result = getSum(10,20);
print(result);
}
def getSum( a:Int, b:Int ) = {
var sum:Int = 0
sum = a + b
sum
}
}
Output:
30
Similarly, we can return string values as well. The function takes two string arguments based on the last expression/statement type.
Code:
object MyClass {
def main(args: Array[String]) {
var result = getSum("Hello","Hi");
print(result);
}
def getSum( a:String, b:String ) = {
var sentence:String = ""
sentence = a + b
sentence
}
}
Output:
HelloHi
Return a List or Vector Value in Scala
We can also return a complete container such as an array, list, or vector. The function returns a vector after looping and yielding in the code below.
Code:
object MyClass {
def main(args: Array[String]) {
var result = getList(1,5);
print(result);
}
def getList(a:Int, b:Int ) = {
var list = for( c <- a to b) yield c
list
}
}
Output:
Vector(1, 2, 3, 4, 5)
In Scala, the final expression is assumed to be the return value if there is no return value. If the last expression is what you want to return, you can skip the return
keyword.