How to Merge Two Maps in Scala and Then Sum the Values With the Same Key
-
Merging Two Maps in Scala Using the
++
Operator - Merging Maps and Summing the Values With the Same Key
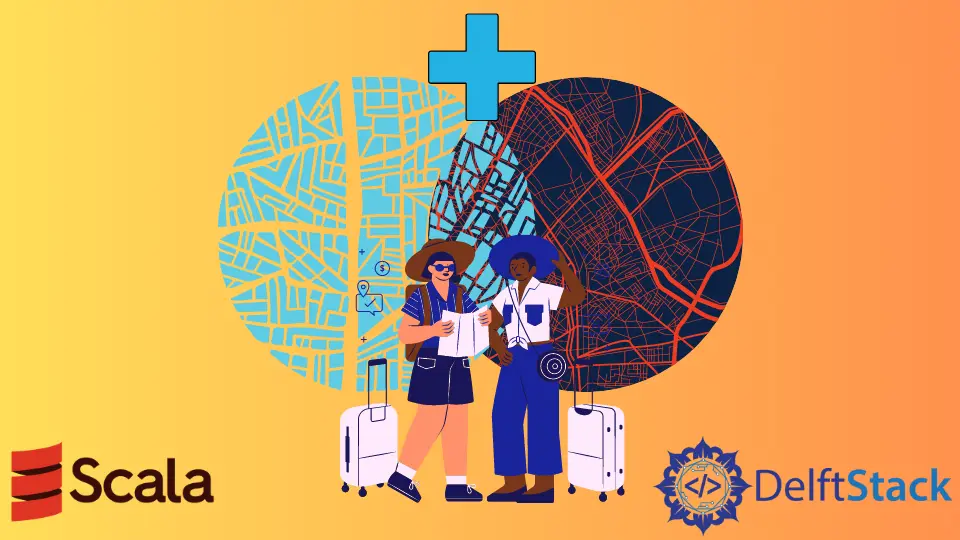
We will see how Maps
are merged using ++
in Scala, and then, we will look at different ways to merge and sum the values with the same key with the modified ++
and merged
method available with HashMap.
Merging Two Maps in Scala Using the ++
Operator
Syntax:
map1.++(map2)
Here map2 is merged with map1, and the resultant map
is returned as output.++
does merge the maps, but it eliminates the duplicates; the latest key, value
pair is considered whenever conflict happens between keys.
Example code:
object Main {
def main(args: Array[String])
{
//let's assume a mapping from Student -> marks
val map1_english = Map("Tony" -> 50, "Ruby" -> 89)
val map2_maths = Map("Tony"->77,"Ruth" -> 100, "Ben" -> 78)
println("Map1 : " + map1_english)
println("Map2 : " + map2_maths)
// merging the map
val mergedMap = map1_english.++(map2_maths)
println("Class marks are : " + mergedMap)
}
}
Output:
Map1 : Map(Tony -> 50, Ruby -> 89)
Map2 : Map(Tony -> 77, Ruth -> 100, Ben -> 78)
Class marks are : Map(Tony -> 77, Ruby -> 89, Ruth -> 100, Ben -> 78)
It can be observed that the latest key, value
pair i.e. Tony,77
is considered in output.
Merging Maps and Summing the Values With the Same Key
Method 1:
As using ++
does merge the maps but it eliminates the duplicate, the idea here is first to convert the maps into a list using toList
, then merge them so that duplicates are preserved, and then use the groupBy
function of the list to group the values based on keys and sum the values
for same keys.
Example code:
object Main {
def main(args: Array[String])
{
val map1_english = Map("Tony" -> 50, "Ruby" -> 89)
val map2_maths = Map("Tony"->77,"Ruby" -> 100, "Ben" -> 78)
val mergedList = map1_english.toList ++ (map2_maths.toList)
println("Merged List : " + mergedList)
val mergedKeys = mergedList.groupBy(_._1).map{case (k,v) => k -> v.map(_._2).sum}
println("Merged Map with summed up values : " + mergedKeys)
}
}
Output:
Merged List : List((Tony,50), (Ruby,89), (Tony,77), (Ruby,100), (Ben,78))
Merged Map with summed up values : Map(Ruby -> 189, Ben -> 78, Tony -> 127)
Method 2:
Using the merged
method available with HashMap.
Example code:
object Main {
def main(args: Array[String])
{
val map1_english = collection.immutable.HashMap("Tony" -> 50, "Ruby" -> 89)
val map2_maths = collection.immutable.HashMap("Tony"->77,"Ruby"-> 100, "Ben"->78)
val merged = map1_english.merged(map2_maths)({ case ((k,v1),(_,v2)) =>(k,v1+v2)})
println("Merged Map with summed up values : " + merged)
}
}
Output:
Merged Map with summed up values : Map(Ruby -> 189, Tony -> 127, Ben -> 78)