How to Log in a Scala Application
- Understanding Scala Logging Libraries
- Setting Up Logback in Scala
- Using Scala Logging for Enhanced Functionality
- Best Practices for Logging in Scala Applications
- Conclusion
- FAQ
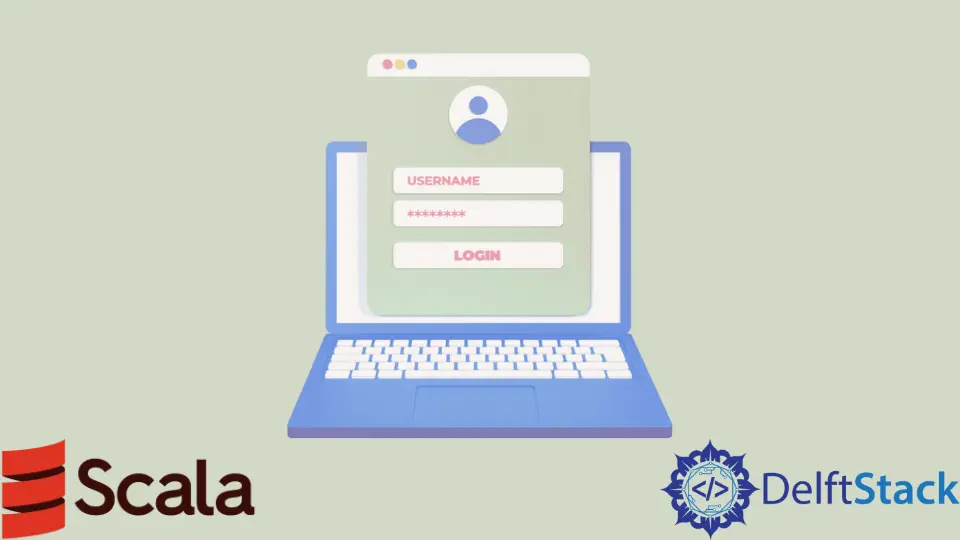
Logging is a crucial aspect of any application, and Scala is no exception. Whether you’re developing a small project or a large-scale enterprise application, effective logging can help you monitor performance, track errors, and gain insights into application behavior.
In this article, we will explore how to implement logging in a Scala application. We will focus on various logging libraries and techniques that can enhance your application’s observability. By the end of this article, you will have a solid understanding of how to log in a Scala application and the best practices to follow.
Understanding Scala Logging Libraries
Before diving into the implementation, it’s essential to understand the various logging libraries available for Scala. Some popular options include:
- Logback: A powerful and flexible logging framework.
- SLF4J: A simple facade for various logging frameworks, allowing you to plug in your preferred logging implementation.
- Scala Logging: A wrapper around SLF4J that provides a more Scala-friendly API.
Choosing the right logging library is crucial for your application’s success. Each library comes with its own set of features, so consider your specific needs, such as performance, ease of use, and integration with other tools.
Setting Up Logback in Scala
Logback is one of the most widely used logging frameworks in the Scala ecosystem. To get started, you’ll first need to add the necessary dependencies to your project. If you’re using SBT, add the following lines to your build.sbt
file:
libraryDependencies += "ch.qos.logback" % "logback-classic" % "1.2.3"
libraryDependencies += "org.slf4j" % "slf4j-api" % "1.7.30"
Once you have the dependencies set up, create a configuration file named logback.xml
in the src/main/resources
directory. Here’s a simple configuration example:
<configuration>
<appender name="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} - %msg%n</pattern>
</encoder>
</appender>
<root level="info">
<appender-ref ref="STDOUT" />
</root>
</configuration>
This configuration sets up a console appender that outputs log messages to the standard output. The log messages will include a timestamp and the message content.
To log messages in your Scala application, you can use the following code:
import org.slf4j.LoggerFactory
object MyApp extends App {
val logger = LoggerFactory.getLogger(this.getClass)
logger.info("Application started")
logger.warn("This is a warning message")
logger.error("An error occurred")
}
Output:
2023-10-01 12:00:00 - Application started
2023-10-01 12:00:01 - This is a warning message
2023-10-01 12:00:02 - An error occurred
In this example, we import the LoggerFactory
from SLF4J and create a logger instance for our application. We then log messages at different levels: info, warning, and error. The output will display the timestamp along with the log messages, providing clear insights into the application’s behavior.
Using Scala Logging for Enhanced Functionality
Scala Logging is a convenient wrapper around SLF4J that simplifies logging in Scala applications. To use Scala Logging, add the following dependency to your build.sbt
:
libraryDependencies += "com.typesafe.scala-logging" %% "scala-logging" % "3.9.2"
Once you’ve added the dependency, you can easily log messages in a more idiomatic Scala way. Here’s how to implement logging using Scala Logging:
import com.typesafe.scalalogging.Logger
object MyApp extends App {
val logger = Logger(this.getClass)
logger.info("Application started")
logger.warn("This is a warning message")
logger.error("An error occurred")
}
Output:
2023-10-01 12:05:00 - Application started
2023-10-01 12:05:01 - This is a warning message
2023-10-01 12:05:02 - An error occurred
With Scala Logging, you can create a logger instance using the Logger
class. The syntax is clean and integrates seamlessly with Scala’s functional programming style. You can log messages at various levels, just like with SLF4J, but with a more Scala-friendly API.
Best Practices for Logging in Scala Applications
When implementing logging in your Scala application, it’s essential to follow best practices to ensure that your logs are useful and maintainable. Here are some key points to consider:
-
Log Levels: Use appropriate log levels (e.g., debug, info, warn, error) to categorize your log messages. This helps you filter logs based on severity and focus on critical issues.
-
Structured Logging: Consider using structured logging formats (like JSON) to make it easier to parse and analyze logs later. This is especially useful when integrating with log management systems.
-
Avoid Logging Sensitive Information: Be cautious about logging sensitive data such as passwords, personal information, or any confidential data. Always sanitize logs to protect user privacy.
-
Log Context: Include relevant context in your log messages, such as user IDs, request IDs, or any other identifiers that can help trace the flow of execution.
-
Performance Considerations: Logging can introduce overhead, so avoid excessive logging in performance-critical sections of your code. Use lazy logging techniques to prevent unnecessary string concatenation.
By adhering to these best practices, you can ensure that your logging strategy is effective, efficient, and secure.
Conclusion
In this article, we explored how to implement logging in a Scala application using popular libraries like Logback and Scala Logging. We discussed the setup process, provided code examples, and highlighted best practices to follow for effective logging. By incorporating these techniques, you can enhance your application’s observability, making it easier to diagnose issues and monitor performance. Remember, good logging is an integral part of software development, and investing time in it will pay off in the long run.
FAQ
-
What is the best logging library for Scala?
Logback and Scala Logging are popular choices, with Logback being a robust framework and Scala Logging providing a more idiomatic Scala API. -
How can I configure logging levels in Logback?
You can configure logging levels in thelogback.xml
file by setting the<root level="info">
to your desired level (e.g., debug, warn, error). -
Is it safe to log sensitive information in my application?
No, you should avoid logging sensitive information such as passwords or personal data to protect user privacy and comply with regulations.
-
Can I use multiple logging libraries in a Scala application?
While it’s technically possible, it’s best to stick to one logging library to avoid conflicts and maintain consistency. -
How can I improve the performance of logging in my application?
Use lazy logging techniques and avoid excessive logging in performance-critical sections to reduce overhead.