How to Uses of implicit in Scala
-
implicit
as a Parameter Value Injector in Scala -
implicit
as a Type Converter in Scala -
implicit
as an Extension Method in Scala
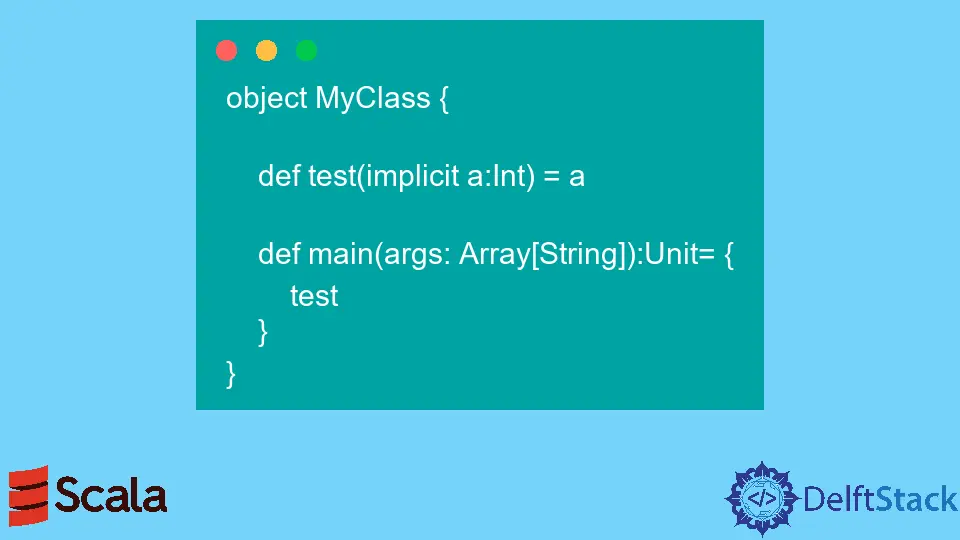
This article will discuss the different uses of implicit
in Scala.
implicit
as a Parameter Value Injector in Scala
Implicit parameters are passed to a method with the implicit
keyword in Scala.
The values are taken from the context (scope) in which they are called. In simple words, if no value or parameter is passed to a function, then the compiler looks for an implicit
value and passes it as a parameter.
Example 1:
object MyClass {
def test(a:Int) = a
def main(args: Array[String]) {
test //calling the function but this gives error as no value is passed
}
}
Here we called a function without passing any parameter value. This leads to an error. Scala compiles that first; it tries to pass a value, but it will not get the parameter’s direct value.
Example 2:
object MyClass {
def test(implicit a:Int) = a
def main(args: Array[String]):Unit= {
test
}
}
Here we called a function with a parameter with the implicit
keyword. The Scala compiler will look for any val
in the same scope with the same value type.
If found, it compiles successfully; else, we get an error. So, as there is no value in the scope of the same type, the above program gives an error.
Example 3:
object MyClass {
def test(implicit a:Int) = a
implicit val b:Int =789
def main(args: Array[String]):Unit= {
println(test)
}
}
Output:
789
The above code runs successfully because the compiler finds an implicit val
with the same type int
as the implicit parameter a
in the function.
implicit
as a Type Converter in Scala
We can also use implicit
keywords to convert one data type.
We convert string
to int
type in the code below.
object MyClass {
def main(args: Array[String]):Unit= {
val str :String = "hero"
val x:Int = str //we get error here
}
}
Since string
is not a sub-type of int
, it will error. The Scala compiler looks for an implicit
function in the scope which takes string
as an argument and returns an int
.
Here is another simple example.
object MyClass {
implicit def myfunc(a:String):Int = 500
def main(args: Array[String]):Unit= {
val str :String = "hero"
val x:Int = str // the compiler treats this as myfunc(str) and return 500
println(x)
}
}
Output:
500
The above code perfectly runs because when the Scala compiler looks for an implicit
function, it finds and treats val x:Int = str
as myfunc(str)
and returns 500.
implicit
as an Extension Method in Scala
Suppose we add a new method to the integer object, converting the meter to the centimeter. We create an implicit class
inside an object to achieve this.
This implicit class will have only one constructor parameter.
Below is a complete code example to better understand how it works.
object MyObject {
implicit class mtoCm(m:Int){
def mToCm={
m*100
}
}
}
We can implement this using the following code.
import MyObject._
object workspace {
def main(args: Array[String]):Unit= {
println(3.mToCm) //calling the mtoCm from MyObject
}
}
Output:
300
This imports the defined implicit class
, which we want to use from the above code.