How to Get the Current Timestamp in Scala
-
Get the Current Timestamp Using the
now()
Method in Scala -
Get the Current Timestamp Using the
Date
Class in Scala
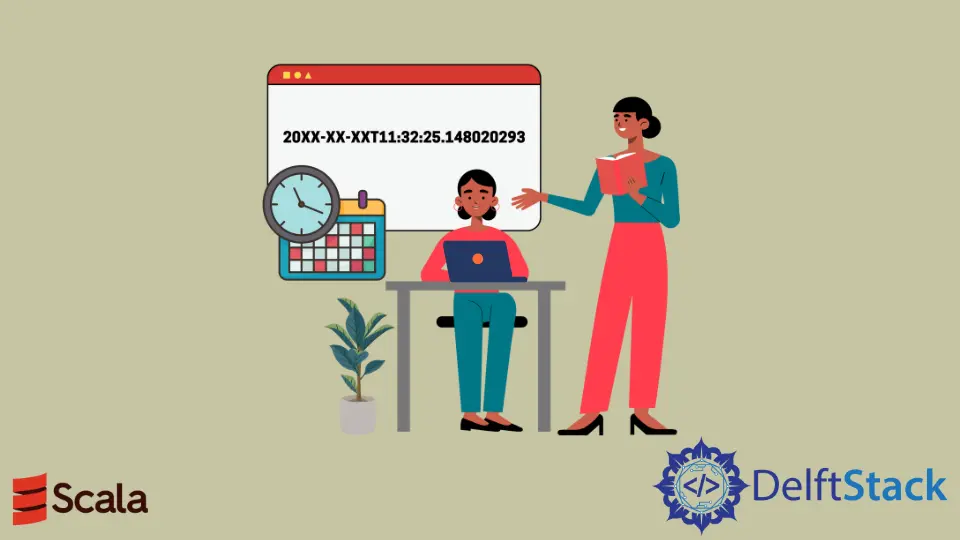
This tutorial will discuss the procedure to get the current timestamp in Scala.
Date and time are essential parts of every computer language, including Scala. Scala supports the Java date-time API, so we can use its classes and methods to handle the date-time.
The timestamp is a combination of date and time, such as 2022-03-12T11:32:25.148020293
. Let’s understand with some examples.
Get the Current Timestamp Using the now()
Method in Scala
Here, we use the now()
method of the LocalDateTime
class that returns the current date-time.
This is a static method, so we don’t need the object of LocalDateTime
to call it. We can directly call it using the class name.
Example:
import java.time.LocalDateTime
object MyClass
def main(args: Array[String]) {
val timstamp = LocalDateTime.now()
println(timstamp)
}
}
Output:
2022-03-12T11:32:25.148020293
The timestamp returned by the above code uses a specific format. But if you wish to use another, use the ofPattern()
method.
The ofPattern()
method takes a string argument, and using the format()
method, it returns the required formatted timestamp.
Example:
import java.time.LocalDateTime
import java.time.format.DateTimeFormatter
object MyClass {
def main(args: Array[String]) {
val timstamp = LocalDateTime.now()
val timstamp2 = DateTimeFormatter.ofPattern("yyyy-MM-dd_HH:mm").format(timstamp)
println(timstamp2)
}
}
Output:
2022-03-12_11:37
Get the Current Timestamp Using the Date
Class in Scala
We can also use the Date
class to get the current Timestamp in Scala. We used the Date()
constructor that returns the date-time in a new format.
Example:
import java.util.Date
object MyClass {
def main(args: Array[String]) {
val timstamp = new Date()
println(timstamp)
}
}
Output:
Sat Mar 12 11:42:28 GMT 2022
Again, if you wish to format the date-time of the Date
class, use the format()
method and provide the format string in the SimpleDateFormat()
constructor.
Example:
import java.util.Date
import java.text.SimpleDateFormat
object MyClass {
def main(args: Array[String]) {
val timstamp = new Date()
val timstamp2 = new SimpleDateFormat("YYYYMMdd_HHmmss").format(timstamp)
println(timstamp2)
}
}
Output:
20220312_114449