Difference Between ::: And ++ for Concatenating Lists in Scala
Suraj P
Jan 30, 2023
- Concatenation of Lists in Scala
-
Use
:::
to Concatenate Lists in Scala -
Use
++
to Concatenate Lists in Scala
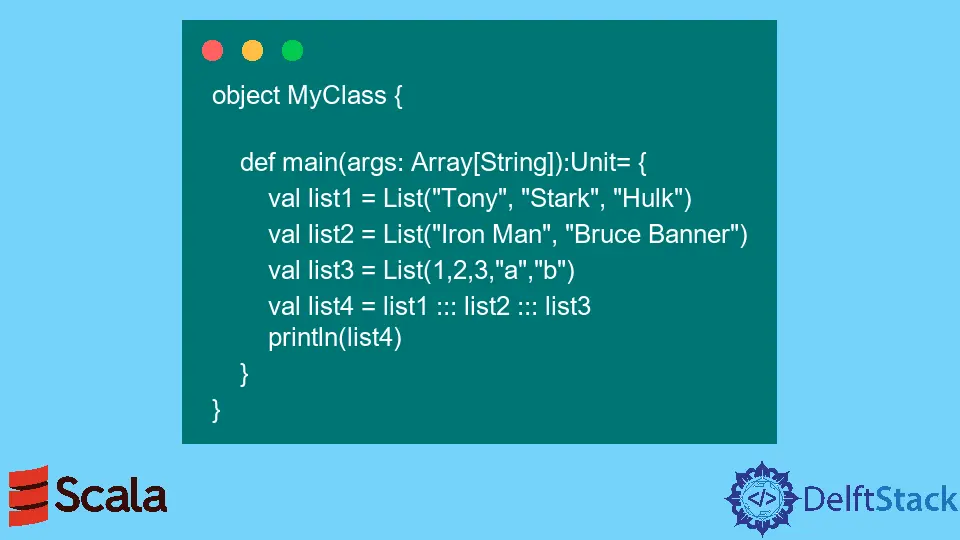
In this tutorial, we’ll look at the differences between the :::
and ++
concatenation methods for Lists.
Concatenation of Lists in Scala
In Scala, a list is a collection used to store data in the form of a linked list.
Example:
val names: List[String] = List("Tony", "Stark", "Hulk")
There are two methods to concatenate lists in Scala. The ++
and :::
operators.
Use :::
to Concatenate Lists in Scala
The :::
operator can only be used to concat lists. In terms of computation, using the :::
operator is faster as it is right-associative.
Syntax:
val finalList = list1 ::: list2 ::: list3 ::: list4.........
Example:
object MyClass {
def main(args: Array[String]):Unit= {
val list1 = List("Tony", "Stark", "Hulk")
val list2 = List("Iron Man", "Bruce Banner")
val list3 = List(1,2,3,"a","b")
val list4 = list1 ::: list2 ::: list3
println(list4)
}
}
Output:
List(Tony, Stark, Hulk, Iron Man, Bruce Banner, 1, 2, 3, a, b)
Use ++
to Concatenate Lists in Scala
The ++
operator concatenates any two collections, not just lists. This method is very versatile as it can work with any two collections.
Syntax:
val finalList = list1 ++ list2 ++ list3 ++ list4.........
Example 1:
object MyClass {
def main(args: Array[String]):Unit= {
val list1 = List("Tony", "Stark", "Hulk")
val list2 = List("Iron Man", "Bruce Banner")
val list3 = List(1,2,3,"a","b")
val list4 = list1 ++ list2 ++ list3
println(list4)
}
}
Output:
List(Tony, Stark, Hulk, Iron Man, Bruce Banner, 1, 2, 3, a, b)
Example 2:
object MyClass {
def main(args: Array[String]):Unit= {
val seq1 = Seq(1,3,5,7,9)
val seq2 = Seq(2,4,6,8)
val seq3 = seq1 ++ seq2
println(seq3)
}
}
Output:
List(1, 3, 5, 7, 9, 2, 4, 6, 8)
Let’s summarize the differences between the two operators.
::: operator |
++ operator |
---|---|
Only lists can be concatenated using this. | Any two collections can be concatenated using this, not just lists. |
It is faster compared to ++ as it is right-associative. |
It is slower compared to ::: when used for concatenating lists. |
Not versatile. | Versatile as it can concat any two iterables. |