Difference Between && and & Operators in Scala
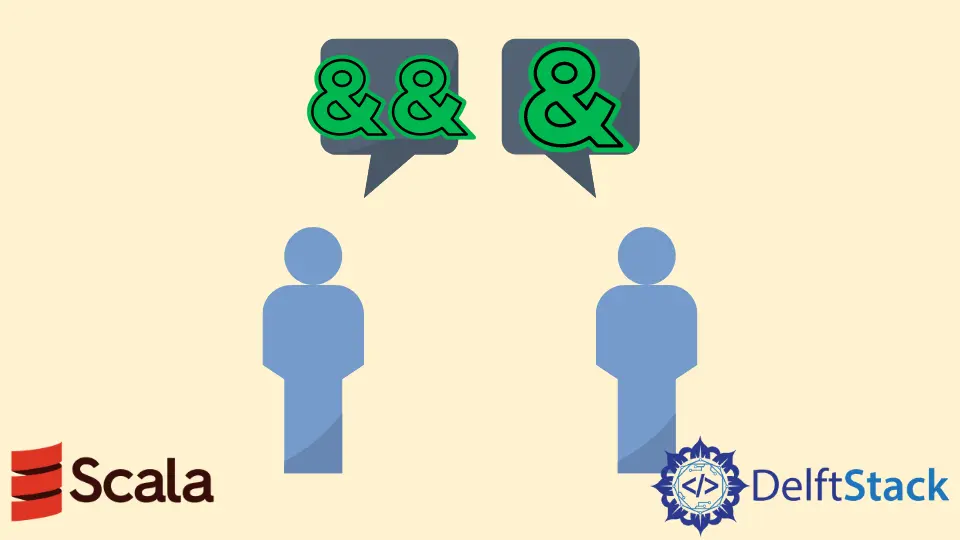
In the world of Scala programming, understanding the nuances of operators is crucial for writing efficient and effective code. Among these operators, the &&
and &
operators often cause confusion among developers, especially those new to the language. While both operators are used for logical operations, they serve different purposes and behave differently in certain situations.
In this article, we will explore the differences between the &&
and &
operators in Scala, highlighting their usage, performance implications, and practical examples. By the end, you will have a clear understanding of when to use each operator, which will enhance your coding skills and improve your code’s performance.
Understanding the && Operator
The &&
operator is known as the short-circuit logical AND operator. It evaluates two boolean expressions and returns true
only if both expressions are true. However, the key feature of &&
is that it does not evaluate the second expression if the first one is false. This behavior can lead to performance benefits, especially when the second expression is computationally expensive or could potentially cause an error.
Example of the && Operator
Let’s take a look at a simple example to illustrate the use of the &&
operator.
val x = 5
val y = 10
val result = (x > 0) && (y / x > 1)
println(result)
Output:
true
In this example, the first expression (x > 0)
evaluates to true
, so the second expression (y / x > 1)
is evaluated, which also returns true
. If x
were 0
, the second expression would not be evaluated, preventing a division by zero error.
The short-circuit nature of &&
makes it particularly useful in scenarios where the second condition relies on the first condition being true. This can help avoid unnecessary computations and potential runtime errors, making your code more efficient and safer.
Understanding the & Operator
On the other hand, the &
operator is a non-short-circuit logical AND operator. Unlike &&
, it evaluates both expressions regardless of the result of the first expression. This means that even if the first expression is false, the second expression will still be evaluated.
Example of the & Operator
To demonstrate how the &
operator works, consider the following example:
val a = 5
val b = 0
val result = (a > 0) & (b / a > 1)
println(result)
Output:
false
In this case, the first expression (a > 0)
evaluates to true
, but the second expression (b / a > 1)
leads to division by zero. Unlike &&
, which would skip the second evaluation, &
evaluates both expressions, resulting in a runtime error. This characteristic makes &
less commonly used for logical operations where short-circuiting is preferred.
It’s essential to understand the implications of using &
in your code. While it can be useful in certain scenarios, such as when you want to ensure both conditions are checked regardless of the outcome of the first, it can also lead to unintended consequences if not used carefully.
When to Use && vs. &
Choosing between &&
and &
ultimately depends on the specific requirements of your code. If you want to ensure that the second condition is evaluated only if the first condition is true, then &&
is the way to go. This is particularly useful for preventing errors and optimizing performance.
Conversely, if you have a scenario where both conditions must be evaluated, regardless of the outcome of the first, then &
is appropriate. However, be cautious, as this can lead to performance issues or runtime errors if the second condition is not safe to evaluate in all cases.
In summary, understanding the differences between &&
and &
operators in Scala is crucial for writing efficient and effective code. By utilizing the correct operator based on your needs, you can improve your code’s performance and reduce the likelihood of errors.
Conclusion
In conclusion, the &&
and &
operators in Scala serve distinct purposes in logical operations. The &&
operator is a short-circuit operator that evaluates the second expression only if the first one is true, making it safer and more efficient in many cases. In contrast, the &
operator evaluates both expressions regardless of the first’s outcome, which can lead to runtime errors if not used carefully. By understanding these differences, you can make informed decisions about which operator to use in your Scala code, enhancing both performance and reliability.
FAQ
-
What is the main difference between && and & in Scala?
The main difference is that&&
is a short-circuit operator, meaning it only evaluates the second expression if the first one is true. In contrast,&
evaluates both expressions regardless of the first’s result. -
When should I use the && operator?
You should use the&&
operator when you want to avoid unnecessary evaluations and potential errors, especially when the second condition depends on the first being true. -
Can using & lead to runtime errors?
Yes, using the&
operator can lead to runtime errors if the second expression is not safe to evaluate when the first expression is false. -
Are there performance implications when using these operators?
Yes, using&&
can improve performance by avoiding unnecessary evaluations, while&
may lead to slower performance due to evaluating both expressions. -
Is it common to use & in Scala?
While&
can be used in Scala, it is less common for logical operations compared to&&
, which is generally preferred for its short-circuit behavior.