How to Convert String to Integer in Scala
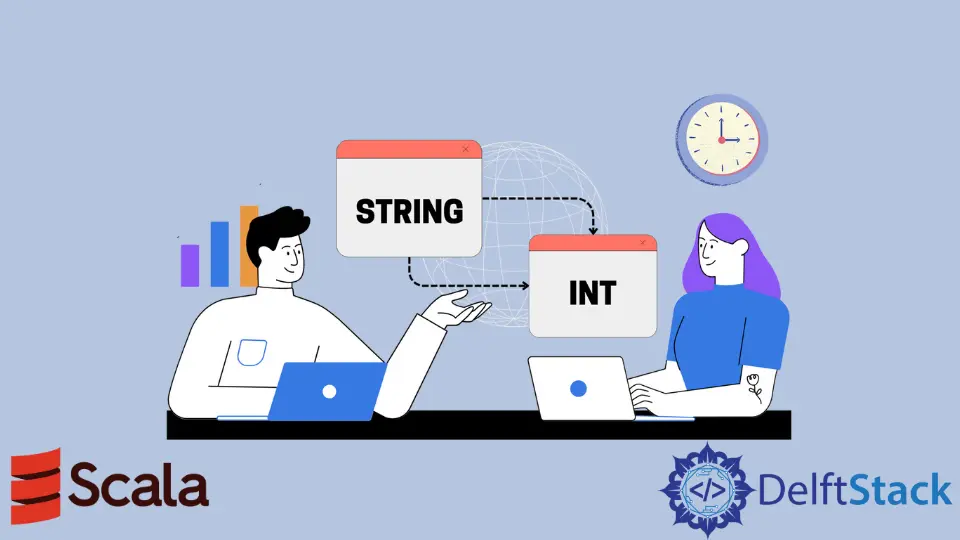
This article will tackle converting a String
to an integer
in Scala.
Use the toInt
Method in Converting String to Integer in Scala
In Scala, if we want to convert a String to an Integer, we can use the toInt
method. It is available on String
objects.
Syntax:
our_String.toInt
Example Code:
object MyClass {
def main(args: Array[String]) {
val str = "1234"
val number = str.toInt
println(number)
println(number.getClass)
}
}
Output:
1234
int
We used the toInt
method to cast the string "1234"
to an integer, but the issue with the above code is that if the string is not a pure integer, we will get NumberFormatException
just like in the following example output.
Example Code:
object MyClass {
def main(args: Array[String]) {
val str = "scala"
val number = str.toInt
println(number)
println(number.getClass)
}
}
Output:
java.lang.NumberFormatException: For input string: "scala"
at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:67)
So we have to handle this exception using a try-catch
block. This next code snippet is a more Java-like
way of converting a string to an integer and handling the exception.
Example Code:
object MyClass {
def toInt(str: String): Int =
{
try {
str.toInt
}
catch {
case ex: Exception => 0
}
}
def main(args: Array[String]) {
val str = "scala"
val number = toInt(str)
println(number)
}
}
Output:
0
The function toInt
returns the correct value if the string can be converted (like if the input is "1234"
); else, it returns 0
if it is not possible to convert (like if the input is "scala"
).
Let’s have another example of converting a string to an integer using Option[]
.
Example Code:
object MyClass {
def toInt(str: String): Option[Int] =
{
try {
Some(str.toInt)
}
catch {
case ex: Exception => None
}
}
def main(args: Array[String]) {
val str = "scala"
val number = toInt(str)
println(number)
}
}
Output:
None
The function toInt
returns Some(Int)
if the string can be converted (like if the input is "1234"
); else, it returns None
if it is not possible to convert it to an integer.
We can also write our Scala toInt
method and Try
, Sucess
, and Failure
.
Example Code:
import scala.util.{Try, Success, Failure}
object MyClass {
def makeInt(str: String): Try[Int] = Try(str.trim.toInt)
def main(args: Array[String]) {
val str = "scala"
val number = makeInt(str)
println(number)
}
}
Output:
Failure (java.lang.NumberFormatException: For input string: "scala")