The break Statement in Scala
- Syntax of Break in Scala
-
Use the
breakable
Method to Stop a Loop in Scala -
Use the
breakable
Method to Stop an Inner Loop in Scala -
Use the
return
Statement to Break the Loop in Scala - Use the Conditional Statement to Break the Loop in Scala
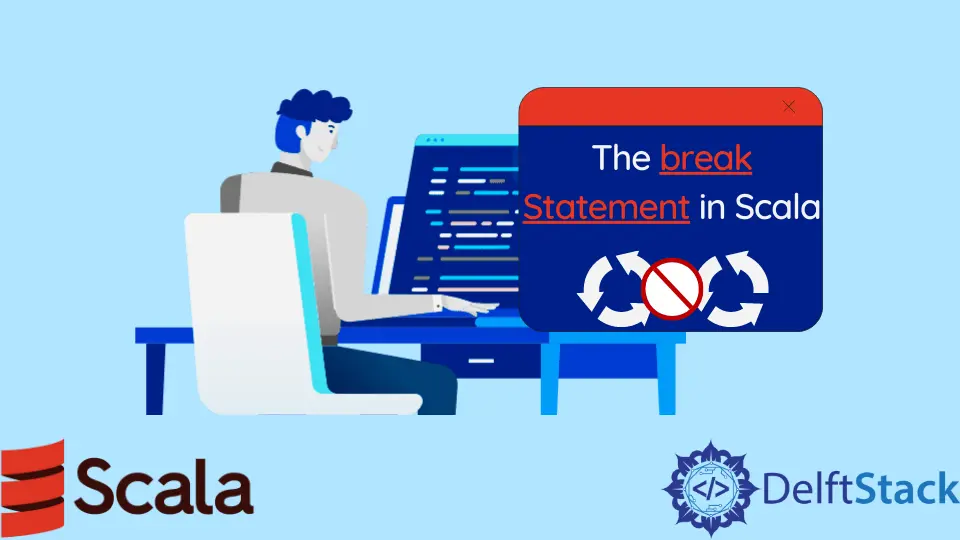
We will discuss in this article the break statement and its uses in Scala.
The break is one of the common features of any programming language used to control the code execution flow. In Scala, we can use the break to stop the loop iterations.
Scala does not provide any break statement but a break method located into the scala.util.control.Breaks
package. You must import the package into your code.
Syntax of Break in Scala
Scala provides a breakable {}
block inside which we can use the break statement. The following code is the general syntax of a break in Scala, and we use it to enclose the code to break the flow.
Syntax:
breakable {
for (x <- xs) {
if (cond)
break // breaking here
}
}s
Use the breakable
Method to Stop a Loop in Scala
Here, we have a loop that iterates 1 to 10 and breaks if the value is 5
. The execution stops immediately after matching the condition and control back to out of the loop, and the rest of the code executes.
import scala.util.control.Breaks._
object MainObject {
def main(args: Array[String]) {
breakable {
for(i<-1 to 10 by 2){
if(i==5)
break
else
println(i)
}
}
}
}
Output:
1
3
Use the breakable
Method to Stop an Inner Loop in Scala
You can use the breakable
method to terminate the execution of the inner loop in a nested loop. If you are working with a nested loop and want to stop the inner loop execution-only, then use the break statement inside the inner loop and see the result.
import scala.util.control.Breaks._
object MainObject {
def main(args: Array[String]) {
for(i <- 1 to 3){
breakable {
for(j <- 1 to 3){
if(i == 2 & j == 2 )
break
println(i+" "+j)
}
}
}
}
}
Output:
1 1
1 2
1 3
2 1
3 1
3 2
3 3
Use the return
Statement to Break the Loop in Scala
We used the return
statement to get out of the loop, which is a valid statement, but the issue is it does not execute the code written after the return
statement as the control goes out to the caller function.
import scala.util.control.Breaks._
object MainObject {
def main(args: Array[String]) {
var sum = 0
for (i <- 0 to 1000) {
sum += i;
if (sum>=10)
return
println(sum)
}
}
}
Output:
0
1
3
6
Use the Conditional Statement to Break the Loop in Scala
Conditionally breaking a loop is also one of the alternatives where you can set a flag and specify a condition. If the condition meets, then the loop stops execution.
import scala.util.control.Breaks._
object MainObject {
def main(args: Array[String]) {
var done = false
var i = 10
var sum = 0
while (i <= 1000 && !done) {
sum+=i
if (sum > 10) {
done = true
}
println(sum)
}
}
}
Output:
10
20