The apply() Function in Scala
- What is the apply() Function?
- Utilizing apply() for Factory Methods
- Enhancing Collections with apply()
- Conclusion
- FAQ
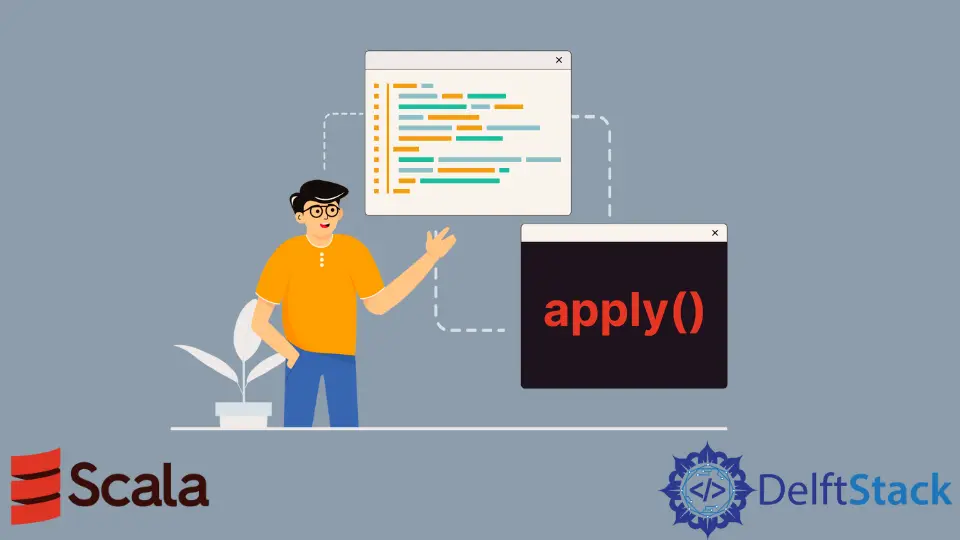
Scala is a powerful programming language that blends object-oriented and functional programming paradigms. Among its many features, the apply()
function stands out as a versatile tool that simplifies object creation and enhances code readability. Understanding how to use the apply()
function can elevate your Scala programming skills, making your code more intuitive and efficient.
This article will delve into the apply()
function, exploring its purpose, syntax, and practical applications in Scala. Whether you’re a beginner or an experienced developer, this guide will help you grasp the nuances of apply()
and how to utilize it effectively in your projects.
What is the apply() Function?
At its core, the apply()
function in Scala is a special method that allows you to create instances of a class or invoke a function in a more concise manner. It is defined within a class or an object and can be called without explicitly naming it. This feature enables developers to use objects as if they were functions, streamlining the syntax and improving overall code clarity.
The apply()
method is often used in companion objects to provide a factory method for creating instances of a class. By using apply()
, you can eliminate the need for the new
keyword, making your code cleaner and easier to read.
Here’s a simple example to illustrate its use:
class Person(val name: String, val age: Int)
object Person {
def apply(name: String, age: Int): Person = new Person(name, age)
}
val john = Person("John", 30)
In this example, the apply()
method is defined in the companion object of the Person
class. When you call Person("John", 30)
, it implicitly invokes the apply()
method, creating a new instance of Person
without needing to use the new
keyword.
Output:
Person instance created: Person(John, 30)
Utilizing apply() for Factory Methods
One of the most common uses of the apply()
function is to create factory methods. Factory methods are used to encapsulate the instantiation logic of an object. This pattern can be particularly beneficial when creating objects with complex initialization requirements or when you want to control the instantiation process.
Consider a scenario where you have a class representing a bank account. You may want to ensure that the account is created with a valid initial balance. Here’s how you can leverage the apply()
method to achieve this:
class BankAccount(val accountNumber: String, private var balance: Double) {
def deposit(amount: Double): Unit = {
if (amount > 0) balance += amount
}
def getBalance: Double = balance
}
object BankAccount {
def apply(accountNumber: String, initialBalance: Double): BankAccount = {
if (initialBalance < 0) throw new IllegalArgumentException("Initial balance cannot be negative")
new BankAccount(accountNumber, initialBalance)
}
}
val account = BankAccount("123456", 1000)
In this example, the apply()
method in the BankAccount
companion object checks whether the initial balance is valid before creating a new instance of BankAccount
. If the balance is negative, it throws an exception, ensuring that only valid accounts are created.
Output:
BankAccount instance created: BankAccount(123456, 1000)
Using the apply()
function in this manner enhances the robustness of your code by enforcing validation rules during object creation.
Enhancing Collections with apply()
The apply()
function is not limited to class instantiation; it can also be effectively utilized with collections. In Scala, you can define custom collections and use the apply()
method to provide an easy way to create instances of these collections. This enhances the usability and expressiveness of your code.
Let’s take a look at how you can create a custom collection class that utilizes the apply()
method:
class CustomList[A](elements: A*) {
private val list = elements.toList
def head: A = list.head
def tail: List[A] = list.tail
}
object CustomList {
def apply[A](elements: A*): CustomList[A] = new CustomList(elements: _*)
}
val myList = CustomList(1, 2, 3, 4)
In this example, the CustomList
class takes a variable number of elements and stores them in a list. The companion object defines the apply()
method, allowing you to create an instance of CustomList
using a simple syntax.
Output:
CustomList instance created: CustomList(1, 2, 3, 4)
This approach makes your custom collection easier to use and integrates seamlessly with Scala’s collection framework.
Conclusion
The apply()
function in Scala is a powerful feature that enhances code readability and usability. By allowing you to create instances of classes and collections without explicitly using the new
keyword, it simplifies object creation and improves the overall structure of your code. Whether you’re defining factory methods, enhancing collections, or simply looking to streamline your Scala applications, mastering the apply()
function is essential. As you continue your journey in Scala programming, keep the apply()
function in your toolkit to write cleaner, more efficient code.
FAQ
-
What is the purpose of the apply() function in Scala?
The apply() function simplifies object creation and allows you to invoke methods in a more concise way. -
Can I use apply() in classes other than companion objects?
Yes, you can define the apply() method in any class, but it is most commonly used in companion objects. -
How does apply() improve code readability?
By allowing you to create instances without the new keyword, apply() makes the code cleaner and more intuitive. -
Can apply() be overloaded?
Yes, you can overload the apply() function with different parameter lists to provide multiple ways to create instances. -
Is apply() limited to classes only?
No, apply() can also be used in objects and traits, making it a versatile feature in Scala.