How to Compile Error When Printing an Integer in Rust
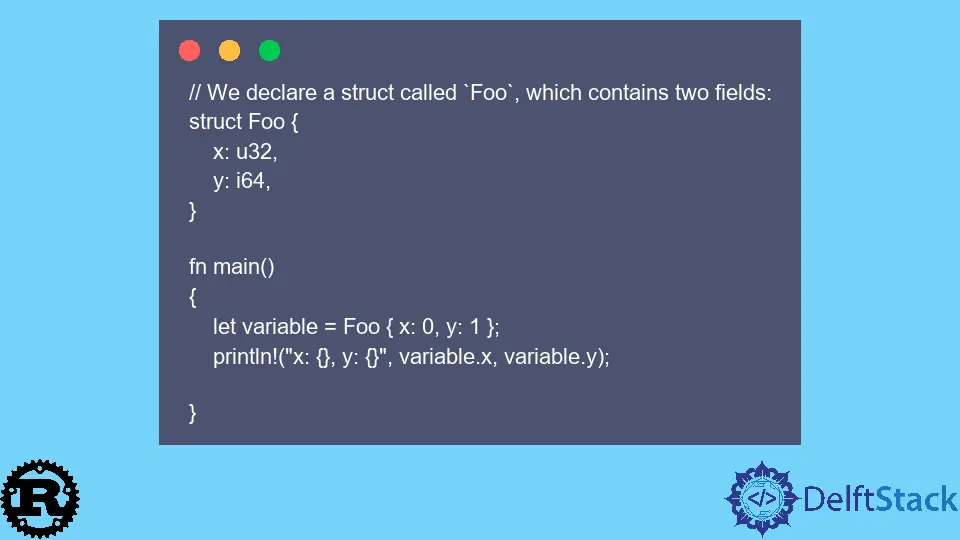
This article is about avoiding compile errors when trying to print an integer in Rust v0.13.0
.
Compilation Error in Rust
A compilation error occurs when a compiler struggles to build a portion of computer program source code, either because of flaws in the code or, more often, because of compiler problems.
To avoid the compilation error in Rust v0.13.0
, we need to explicitly give an integer type.
Example Code:
fn main(){
let x = 10;
println!("x = {}", x);
}
Output:
x = 10
The code will work in the new compiler with Rust v0.13.0
; we do not need to specify i32
explicitly. However, we can use i32
to print the integer without compilation error.
Example Code:
fn main(){
let x: i32 = 15;
println!("x = {}", x);
}
Output:
x = 15
Access a Field on a Primitive Type in Rust
Code example with error:
let x: u32 = 0;
println!("{}", x.foo);
The above code has errors, and it does not print the integers.
The right code for printing the value of integers is:
// We declare a struct called `Foo`, which contains two fields:
struct Foo {
x: u32,
y: i64,
}
fn main()
{
let variable = Foo { x: 0, y: 1 };
println!("x: {}, y: {}", variable.x, variable.y);
}
We declare a struct
called Foo
, which contains two fields: x
and y
. We created an instance of Foo
to access its fields.
Output:
x: 0, y: 1
Add Two Integers in Rust
The compile error can be avoided using the code below for adding two integers and printing its result.
Example Code:
fn main() {
let x: i32 = 20;
let y: i32 = 40;
let sum = return_sum(x, y);
println!("The sum is = {}", sum);
}
fn return_sum(i: i32, j: i32) -> i32 {
i + j
}
Output:
The sum is = 60