How to Use Macro Across Module Files in React
- What Are Macros in React?
- Setting Up Macros in a React Project
- Using the Macro in a React Component
- Advanced Macro Usage
- Conclusion
- FAQ
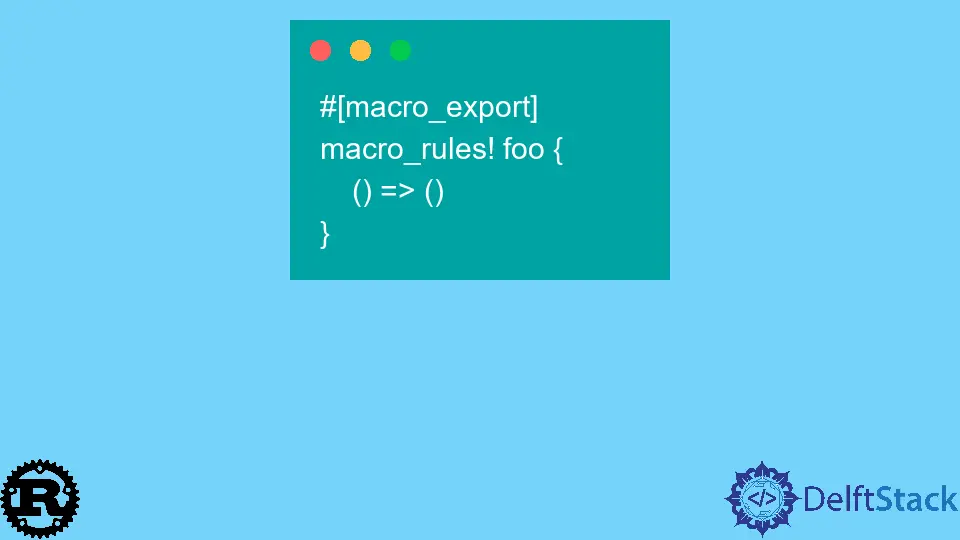
In the world of React development, maintaining clean and efficient code is essential. One powerful technique to achieve this is by using macros across module files. This approach not only enhances code reusability but also streamlines the development process, allowing developers to manage larger projects more effectively.
In this tutorial, we’ll explore how to use macros in React, focusing on practical examples and clear explanations. Whether you’re a seasoned developer or just starting out, understanding how to implement macros can significantly improve your workflow. Let’s dive into the specifics of using macros across module files in React, ensuring you have the tools and knowledge to elevate your coding practices.
What Are Macros in React?
Macros are essentially code transformations that allow you to write more concise and reusable code. In React, macros can help you automate repetitive tasks, making your components cleaner and easier to maintain. By leveraging tools like Babel, you can create macros that operate across different module files, enabling you to share functionality without duplicating code.
Benefits of Using Macros
- Code Reusability: Macros allow you to reuse code snippets across multiple components, reducing redundancy.
- Cleaner Code: By abstracting complex logic into macros, your component files remain clean and focused on their primary purpose.
- Easier Maintenance: Changes made in a macro reflect wherever that macro is used, simplifying updates and bug fixes.
Setting Up Macros in a React Project
To use macros in your React project, you need to set up a few tools. The most common approach is to use Babel with the babel-plugin-macros
package. Here’s how to do it:
Step 1: Install Babel Macros
First, ensure you have Babel installed in your project. If you haven’t set it up yet, run the following command:
npm install --save-dev babel-plugin-macros
Step 2: Configure Babel
Next, you need to configure Babel to use the macros plugin. Create or update your Babel configuration file (usually .babelrc
or babel.config.js
) to include:
{
"plugins": ["macros"]
}
This configuration allows Babel to recognize and process macros in your code.
Step 3: Create a Macro
Now, let’s create a simple macro. Create a new file named myMacro.macro.js
in your project. Here’s a basic example of a macro that logs a message:
export default function myMacro({ references, state }) {
references.default.forEach(referencePath => {
referencePath.replaceWithSourceString('console.log("Hello from Macro!")');
});
}
In this code, we define a macro that replaces calls to myMacro()
with a console log statement.
Output:
Hello from Macro!
This macro will log a message whenever it’s called in your React components.
Using the Macro in a React Component
Now that we have our macro set up, let’s see how to use it in a React component.
Step 1: Import the Macro
In your React component file, import the macro as follows:
import myMacro from './myMacro.macro';
function MyComponent() {
myMacro();
return <div>Check your console!</div>;
}
export default MyComponent;
Step 2: Render the Component
When you render MyComponent
, it will call the macro, which in turn logs the message to the console.
Output:
Hello from Macro!
By using the macro in this way, you can easily add functionality to your components without cluttering them with repetitive code.
Advanced Macro Usage
Once you’re comfortable with basic macros, you can explore more advanced use cases. For instance, you can create macros that accept parameters or manipulate more complex structures.
Example of a Parameterized Macro
Here’s an example of a macro that accepts a string parameter and logs it:
export default function logMessageMacro({ references, state }) {
references.default.forEach(referencePath => {
const message = referencePath.parentPath.get('arguments')[0].node.value;
referencePath.replaceWithSourceString(`console.log("${message}")`);
});
}
In your component, you can use this macro like this:
import logMessage from './logMessage.macro';
function AnotherComponent() {
logMessage("Hello from Parameterized Macro!");
return <div>Check your console again!</div>;
}
export default AnotherComponent;
Output:
Hello from Parameterized Macro!
This example showcases how macros can be dynamic, allowing you to pass different messages for logging. This flexibility makes macros a powerful tool in your React development toolkit.
Conclusion
Using macros across module files in React can significantly enhance your coding experience. By automating repetitive tasks and promoting code reusability, you can maintain cleaner and more efficient codebases. With the steps outlined in this tutorial, you should now be equipped to implement macros in your own projects. As you continue to explore the potential of macros, you’ll find that they can greatly simplify your development process, allowing you to focus on building amazing applications.
FAQ
-
What are macros in React?
Macros in React are code transformations that enable developers to write concise and reusable code, improving efficiency and maintainability. -
How do I set up macros in my React project?
To set up macros, install thebabel-plugin-macros
package and configure Babel to recognize macros in your project. -
Can I create parameterized macros?
Yes, you can create parameterized macros that accept arguments, allowing for dynamic functionality in your components. -
What are the benefits of using macros?
Macros promote code reusability, maintain cleaner code, and make updates easier by centralizing logic in one place. -
Are there any performance impacts when using macros?
Generally, the performance impact is negligible, but it’s essential to test your application to ensure it meets your performance needs.