Garbage Collector in Rust
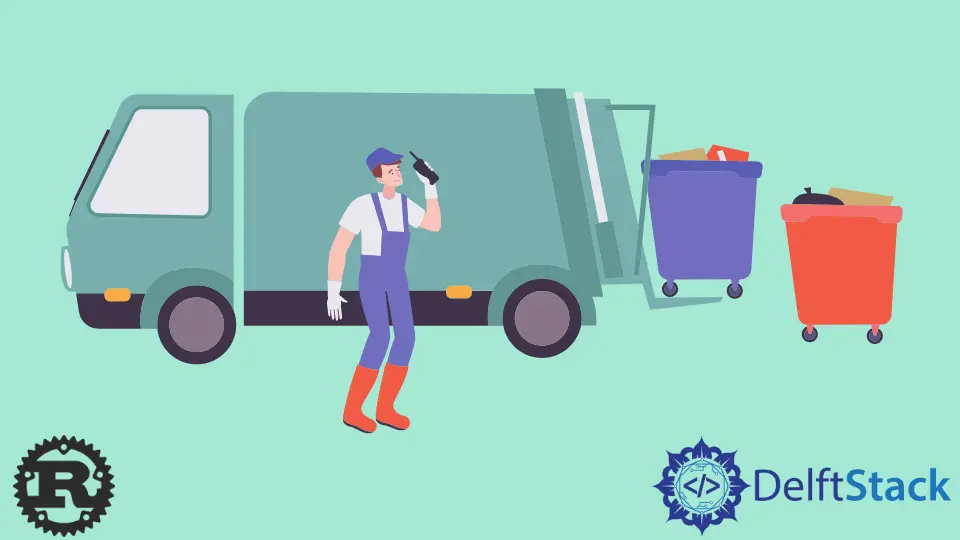
Garbage collection is a crucial aspect of memory management in programming languages, impacting performance and reliability. Rust, known for its focus on safety and performance, approaches memory management differently than languages that rely on garbage collectors. Instead of automatic garbage collection, Rust employs a unique ownership model that eliminates the need for a traditional garbage collector.
In this tutorial, we will explore how Rust manages memory, the implications of its ownership system, and how developers can effectively work with memory without the overhead of garbage collection. Whether you’re a seasoned Rustacean or just starting your journey, this guide will provide valuable insights into Rust’s memory management philosophy.
Understanding Rust’s Ownership Model
Rust’s ownership model is one of its key features that sets it apart from other programming languages. At its core, ownership is about managing memory without the need for a garbage collector. Each piece of data in Rust has a single owner, which is responsible for cleaning up that data when it goes out of scope. This approach ensures memory safety and eliminates common issues like dangling pointers or memory leaks.
When a variable is created, it becomes the owner of the data. If the owner goes out of scope, Rust automatically deallocates the memory. This is done at compile time, meaning that many potential runtime errors are caught before the program even runs. Here’s a simple example to illustrate this concept:
fn main() {
let s = String::from("Hello, Rust!");
println!("{}", s);
}
Output:
Hello, Rust!
In this example, the variable s
owns the String
data. When s
goes out of scope at the end of the main
function, Rust automatically cleans up the memory used by the string. This eliminates the need for a garbage collector, providing both performance benefits and safety guarantees.
Borrowing and References
In addition to ownership, Rust introduces the concept of borrowing. Borrowing allows you to reference data without taking ownership. This is particularly useful when you want to read data without modifying it or when you need to share data between multiple parts of your program. Rust enforces strict rules around borrowing to ensure memory safety.
There are two types of borrowing in Rust: mutable and immutable. Immutable references allow multiple parts of your code to read data simultaneously, while mutable references allow only one part to modify the data at a time. Here’s how borrowing works in practice:
fn main() {
let s = String::from("Hello, Rust!");
let r = &s; // Immutable borrow
println!("{}", r);
}
Output:
Hello, Rust!
In this example, r
is an immutable reference to s
. You can have multiple immutable references to the same data, but you cannot have a mutable reference while immutable references exist. This strict borrowing rule prevents data races and ensures that your program remains safe and efficient.
The Role of Lifetimes
Lifetimes in Rust are a way to ensure that references are valid as long as they are being used. They are a core part of Rust’s ownership and borrowing system. Lifetimes tell the Rust compiler how long a reference is valid, which helps prevent issues like dangling references.
When you create a reference, you can annotate it with a lifetime, which is a way of expressing how long that reference should be valid. Here’s a simple example:
fn longest<'a>(s1: &'a str, s2: &'a str) -> &'a str {
if s1.len() > s2.len() {
s1
} else {
s2
}
}
fn main() {
let s1 = String::from("Hello");
let s2 = String::from("Rust");
let result = longest(&s1, &s2);
println!("The longest string is: {}", result);
}
Output:
The longest string is: Rust
In this example, the function longest
takes two string slices as parameters and returns the longest one. The lifetime annotation 'a
indicates that the returned reference will live at least as long as both input references. This ensures that the returned reference is valid and prevents any potential memory safety issues.
Conclusion
Rust’s approach to memory management, with its ownership model, borrowing, and lifetimes, provides a powerful alternative to traditional garbage collection. By enforcing strict rules around ownership and references, Rust ensures memory safety and performance without the overhead of a garbage collector. Understanding these concepts is crucial for any Rust developer looking to write efficient and safe code. As you continue your journey with Rust, keep these principles in mind to harness the full potential of this remarkable language.
FAQ
-
What is garbage collection in programming?
Garbage collection is an automatic memory management process that reclaims memory occupied by objects that are no longer in use, preventing memory leaks. -
How does Rust manage memory without a garbage collector?
Rust uses an ownership model that enforces strict rules about how memory is allocated and deallocated, eliminating the need for a garbage collector. -
What are the benefits of Rust’s ownership model?
The ownership model provides memory safety, eliminates data races, and improves performance by allowing the compiler to optimize memory usage. -
What are lifetimes in Rust?
Lifetimes are annotations that specify how long references are valid, helping the compiler ensure that references do not outlive the data they point to. -
Can I have multiple mutable references in Rust?
No, Rust allows only one mutable reference to a piece of data at a time to prevent data races, while multiple immutable references are permitted.