How to Get Absolute Value in Rust
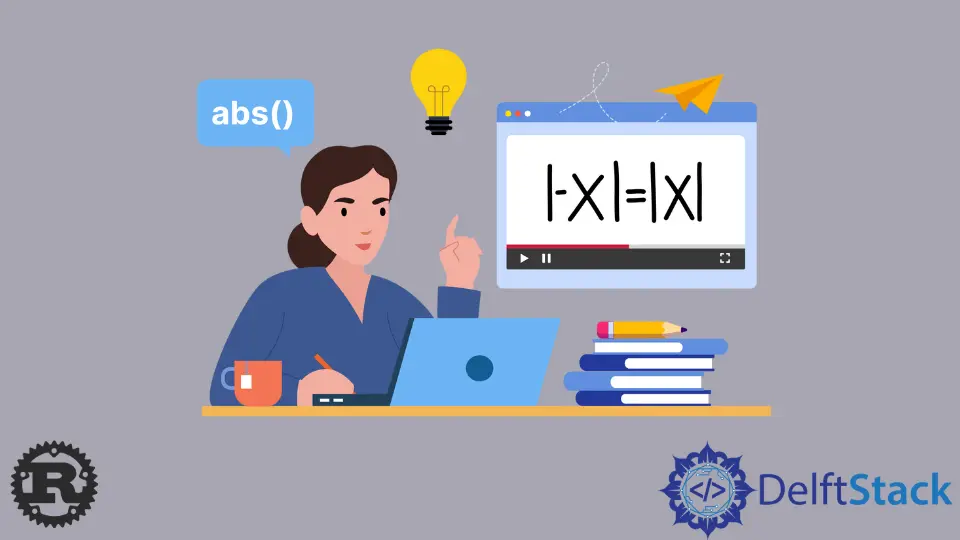
In previous versions of Rust, the std::num::abs
function was used to get the absolute value, but this function is no longer available. These days, the abs()
function is utilized to return the absolute value of most of the number types.
This article will discuss using the abs()
function to get the absolute value in Rust.
Use the abs()
Function to Get Absolute Value in Rust
To get absolute value, we need to specify the data types explicitly. We also need to know that the suffix i
tells Rust that the number in front of the suffix is an integer.
fn main(){
let num = -29i32;
let anum = num.abs();
println!("{}",anum);
}
We defined an immutable variable num
and assigned -29
to it. i32
refers to the 32-bit
signed integer type.
Output:
29
As seen in the output, 29
is an absolute value. Let’s take a look at another example.
fn main() {
let mut num1:i32 = -9;
let mut num2:i32 = 16;
let mut num:i32 = 0;
num = num1.abs();
println!(" The absolute value of num1: {}",num);
num = num2.abs();
println!("The absolute value of num2: {}",num);
}
We added mut
right after let
to make the variable mutable. Variable num1
is assigned -9
, and num2
is assigned 16
.
We will find the absolute value of num1
and num2
using the abs()
function.
Output:
The absolute value of num1: 9
The absolute value of num2: 16
The output above shows that we got the absolute values of num1
and num2
as 9
and 16
.