How to Write a Switch Statement in Ruby
-
Use the
case
Statement in Ruby -
Use the
case
Statement to Match Ranges in Ruby -
Use the
case
Statement to MatchRegEx
in Ruby -
Use the
case
Statement to MatchProc
in Ruby -
Use the
case
Statement With Arrays in Ruby - Conclusion
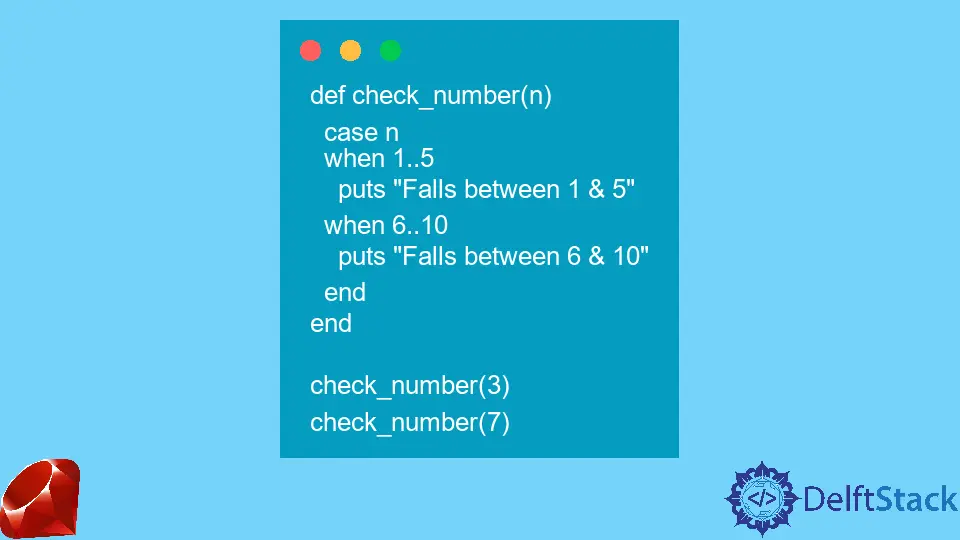
Similar to how the if
statement works, the switch
statement allows us to control the execution of a code based on some specific condition. In Ruby, the case
statement is used as a switch
statement, which is a control flow structure that allows code to be executed based on the value of a variable or expression.
This tutorial will look at how the case
statement is used in Ruby. We will be looking at some basic ways of using it and then some complex examples that are not so common.
Use the case
Statement in Ruby
The case
statement is a powerful control flow structure that allows you to compare a given expression against a set of values and run the code that corresponds to the first matched value. It’s a cleaner alternative to a series of if-elsif-else
statements in certain situations.
Syntax of the case
statement in Ruby:
case argument
when condition
# do something
else
# do something else if nothing else meets the condition
end
In the above syntax, we evaluate a specified argument
, which can be a variable or an expression, against various conditions using when
. If a when
condition is true, the associated code block is executed.
The else
block serves as a default condition, executing its code when none of the conditions in the when
clauses are true. This allows you to provide a fallback or default action, specified under else
, for cases where none of the specified conditions are met.
Let’s give a basic example of how to use a case
statement in Ruby.
Example Codes:
def continent_identifier(country)
case country
when 'Nigeria'
puts 'Africa'
when 'Netherlands'
puts 'Europe'
when 'India'
puts 'Asia'
else
puts "I don't know"
end
end
continent_identifier('India')
continent_identifier('Netherlands')
continent_identifier('Germany')
Output:
Asia
Europe
I don't know
In the code above, we use a case
statement to identify a country’s continent. Looking at the above example, the following are worth noting.
Unlike the switch
statement in most other programming languages, Ruby’s case
statement does not require a break
at the end of each when
. Ruby’s case
statement allows us to specify multiple values for each when
so we can return a result if our variable matches any of the values.
Example Code:
def continent_identifier(country)
case country
when 'Nigeria'
puts 'Africa'
when 'Netherlands', 'Germany'
puts 'Europe'
when 'India'
puts 'Asia'
else
puts "I don't know"
end
end
continent_identifier('India')
continent_identifier('Netherlands')
continent_identifier('Germany')
Output:
Asia
Europe
Europe
The code above defines a Ruby method continent_identifier
that takes a country as input and prints the continent it belongs to. We used the case
statement to check the country and print the corresponding continent.
For India
, it prints Asia
because India
belongs to Asia
. For Netherlands
and Germany
, it prints Europe
because these countries are in Europe
.
Ruby’s case
statement under the hood uses the ===
operator to do the comparison between the case
variable and the values supplied, i.e., value === argument
. As a result, Ruby’s case
allows us to make more clever comparisons.
Use the case
Statement to Match Ranges in Ruby
The case
statement can be utilized to match ranges of values in Ruby. This involves using the when
clauses within the case
statement, where each when
clause is associated with a specific range of values.
When the expression being tested by the case
statement falls within any of the specified ranges, the corresponding code block associated with that when
clause is executed. This provides a clear and organized way to handle multiple conditions based on the ranges of values within a Ruby program.
In this example, we check if the supplied case
variable is included in any of the ranges specified.
Example Code:
def check_number(n)
case n
when 1..5
puts 'Falls between 1 & 5'
when 6..10
puts 'Falls between 6 & 10'
end
end
check_number(3)
check_number(7)
Output:
Falls between 1 & 5
Falls between 6 & 10
In this code, the case
statement checks the value of n
against the specified ranges using the ..
(inclusive range) operator. If n
falls within the range 1..5
, it prints Falls between 1 & 5
.
If n
falls within the range 6..10
, it prints Falls between 6 & 10
. In the output, both 3
and 7
will satisfy one of the range conditions (1..5
and 6..10
respectively), so both messages will be printed.
The case
statement is a convenient way to handle multiple ranges or conditions based on the value of a variable.
Use the case
Statement to Match RegEx
in Ruby
In Ruby, the case
statement can be employed to match regular expressions (RegEx
). This involves using the when
clauses within the case
statement, where each when
clause is associated with a specific regular expression pattern.
When the expression being tested by the case
statement matches any of the specified regular expressions, the corresponding code block associated with that when
clause is executed. This provides a concise and readable way to handle multiple conditions based on regular expression matches within a Ruby program.
Example Code:
def month_indentifier(month)
case month
when /ber$/
puts "ends with 'ber'"
when /ary$/
puts "ends with 'ary'"
end
end
month_indentifier('February')
month_indentifier('November')
Output:
ends with 'ary'
ends with 'ber'
In this code, the regular expressions /ber$/
and /ary$/
are used to check if the given month
parameter ends with 'ber'
or 'ary'
respectively. Depending on which condition is met, the corresponding message is printed.
The month_identifier('February')
matches the second condition and prints ends with 'ary'
, and month_identifier('November')
matches the first condition and prints ends with 'ber'
.
Use the case
Statement to Match Proc
in Ruby
The case
statement can be employed to match Proc
objects in Ruby. This involves using the when
clauses within the case
statement, where each when
clause is associated with a specific Proc
.
A Ruby Proc
encapsulates a block of code that can be stored in a variable or passed around.
When the expression being tested by the case
statement is determined to be equal to one of the specified Proc
objects, the corresponding code block associated with that when
clause is executed. This provides a flexible and expressive way to handle multiple conditions based on different Proc
instances within a Ruby program.
In the following example, we are already using Proc
objects (created with the ->
syntax) as conditions in the when
clauses.
Example Code:
def check_number(number)
case number
when ->(n) { n.even? }
puts "It's even"
when ->(n) { n.odd? }
puts "It's odd"
end
end
check_number(3)
check_number(6)
Output:
It's odd
It's even
In this code, the case
statement checks the value of number
against the conditions provided by the Proc
objects. The ->(n) { n.even? }
creates a Proc
that checks if the given number is even, and ->(n) { n.odd? }
checks if the number is odd.
Depending on which condition is met, the corresponding message is printed. In the output, both 3
and 6
will satisfy one of the conditions (odd?
and even?
respectively), so both messages will be printed.
Use the case
Statement With Arrays in Ruby
In Ruby, the case
statement can be utilized to match arrays. This involves using the when
clauses within the case
statement, where each when
clause is associated with a specific array or array pattern.
When the expression being tested by the case
statement matches any of the specified arrays or array patterns, the corresponding code block associated with that when
clause is executed. This provides a convenient and structured way to handle multiple conditions based on the content or structure of arrays within a Ruby program.
We can use a case
statement with arrays by treating the array as the “target” of the case
and specifying individual arrays as the conditions.
Example Code:
numbers = [1, 2, 3]
case numbers
when [1, 2]
puts 'The array contains 1 and 2.'
when [1, 2, 3]
puts 'The array contains 1, 2, and 3.'
else
puts "The array doesn't match any specified condition."
end
Output:
The array contains 1, 2, and 3.
In this code, the case
statement checks the value of my_array
against the specified conditions. If my_array
matches the first condition ([1, 2]
), the corresponding message is printed.
If it matches the second condition ([1, 2, 3]
), a different message is printed. If the array doesn’t match any specified condition, the else
branch is executed.
Note that this is different from using a case
statement with values (not arrays) as conditions, as in the latter case, the ===
operator is used for matching. When using arrays, the entire array is compared, not individual elements.
Conclusion
The Ruby case
statement provides a flexible and readable way to control code execution based on specific conditions, offering a cleaner alternative to traditional if-elsif-else
structures. The tutorial explores various uses, including matching values, ranges, regular expressions, and Proc
objects.
The simplicity of the syntax and the ability to handle diverse scenarios make the case
statement a powerful tool for efficient and concise decision-making in Ruby programming.